What is Java CLASSPATH?
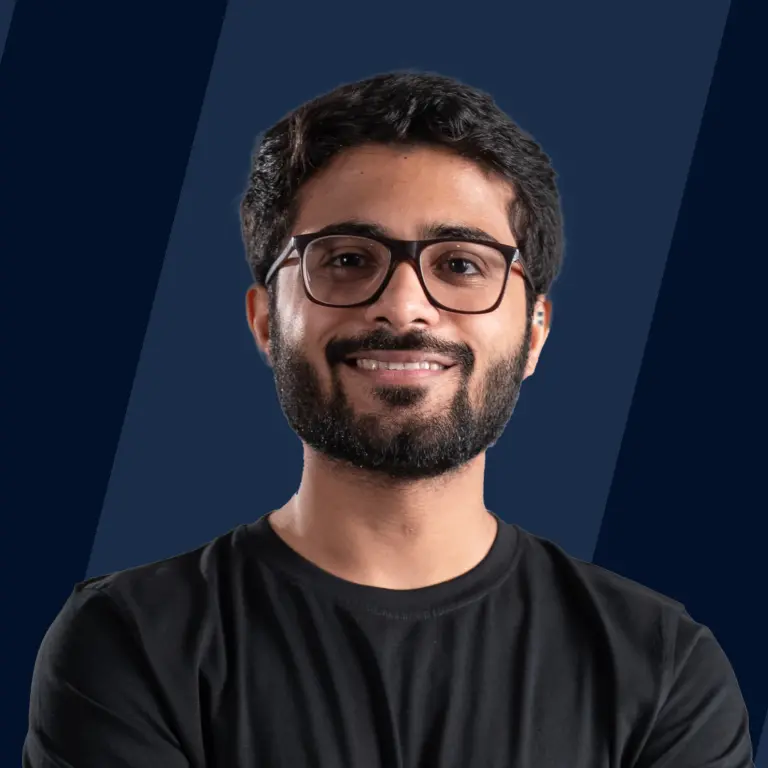
In order to encapsulate groups of classes, interfaces, and sub-packages we use packages in Java Let's see what all packages are used for:
- Avoiding naming disputes: For instance, the packages college.staff.cse.Employee and college.staff.ee.Employee each include two classes with the name Employee.
- Streamlining the use of classes, interfaces, enumerations, and annotations as well as searching for and finding them
- Ensuring restricted access: protected and default have access control at the package level. Classes in the same package and subclasses can access a protected member. Classes belonging to the same package are the only ones with access to a default member (without any access specifier).
Packages are an example of data encapsulation (or data-hiding). In this section, we'll talk about the role of the CLASSPATH environment variable in Java programming. As we go along, importing statements won't be as frequently used.
The CLASSPATH must be configured if:
- A class that is required to be loaded is not found in the current directory or any of its subdirectories.
- You must load a class that is not in the location that the extensions mechanism has designated.
Illustration and Examples of Using Java CLASSPATH
Take a look at this code
What does it all imply, exactly? It makes our current class accessible to the Menu class in the package org.company. such that when we execute the following command:
Let's see how we can implement Java Classpath
Output
This package offers serialization, the file system, and data streams for system input and output. A NullPointerException will be raised if we pass a null argument to a constructor or method in any class or interface in this package. All of the classes named below are imported; if you only wish to import one, follow the instructions below.
The class Menu can be located by the JVM. How will the JVM now be aware of this location?
It is not practical for it to look through each folder on your machine. In order to provide it the location where we want it to look, we use the CLASSPATH variable. The CLASSPATH variable contains directories and jar files.
Let's imagine the directory dir contains the aforementioned package. The Menu class file's complete path is dir/org/company/Menu. We will only include the directory dir in our classpath variable because the import statements will supply the other information about the path. Comparably, if you build a jar and specify its path in the variable, the virtual machine will search the jar file for the class.
In order to see it or to use it and play with multiple IDE, versions of the game simultaneously, one should be able to set a classpath if they haven't already after setting JDK in accordance with operating systems. It must be well understood by the individual
How to Set Java CLASSPATH?
The location of all the necessary files that are utilized by the application is described by CLASSPATH. The CLASSPATH is used by the JVM (Java Virtual Machine) and Java Compiler to find the necessary files. The online Java Compiler will fail to locate the necessary files if the CLASSPATH is not configured, resulting in the following error.
The above error is resolved when CLASSPATH is set
Output:
The CLASSPATH is dependent upon the purpose for which it is set. A directory name or file name appears at the end of the CLASSPATH. What should occur at the end of the CLASSPATH is described in the following sentences.
- If a JAR or zip file contains class files, the name of the zip or JAR file appears at the end of the CLASSPATH.
- The directory containing the class files is where the CLASSPATH stops if the class files are contained in an unnamed package.
- CLASSPATH ends with the directory containing the root package in the whole package name, which is the first package in the full package name if class files are stored in a named package.
- A dot is used as CLASSPATH's default value (.). It indicates that the only directory currently searched. When you set the CLASSPATH variable or use the -classpath command, the default value of CLASSPATH is overridden (for short -cp). If you want to include the current directory, put a dot (.) in the new setting.
- No matter whether a class with the same name is located in another directory that is also included in the CLASSPATH, if CLASSPATH discovers a class file that is present in the current directory, it will load the class and utilize it.
- You must use a semicolon to separate each CLASSPATH if you wish to set more than one classpath (;).
- The CLASSPATH environment variable can be changed by the third-party apps that use the JVM (MySQL and Oracle). The classes can be kept in archives or directories. In rt.jar, the Java platform's classes are kept.
There are two ways to ways to set CLASSPATH: through Command Prompt or by setting Environment Variable. Let's take a look
a) Steps to Set Java CLASSPATH in Windows
Set the CLASSPATH in JAVA in Windows Command Prompt:
You may note that here in the command above, the dot (.) is the default value for CLASSPATH, and the semicolon (;) is used as a separator.
GUI
- Select Start
- Go to the Control Panel
- Select System and Security
- Select Advanced System settings
-
Click on Environment Variables
-
Click on New under System Variables
- Add CLASSPATH as the variable name and the path of files as a variable value.
- Select OK.
b) Steps to Set Java CLASSPATH in UNIX or LINUX
Command Line:
Find out where you have installed Java, basically, it’s in the /usr/lib/jvm path. Set the CLASSPATH in /etc/environment using
Then, add the following lines,
Here, in the command above, the dot (.) is the default value for CLASSPATH, and the colon (:) is used to designate a different directory.
Run to determine the current CLASSPATH.
c) Steps to Set Java CLASSPATH from DOS Prompt
To set the classpath temporarily from DOS prompt, use the command set CLASSPATH.
This method of setting the classpath variable has no lasting effect. Only the DOS window that is currently open will have access to the classpath. It won't be accessible in any additional windows or programs that are launched in brand-new command prompts.
How to Find and Print CLASSPATH Value?
Anytime you want to check every path entry in the CLASSPATH variable, use the echo command to do so.
- Windows
- Linux
You will get CLASSPATH: Undefined variable error (Solaris or Linux) on the terminal or simply %CLASSPATH% written in the Windows command prompt if CLASSPATH is not configured.
Overriding Classpath in Java
When launching your program, you can alter the environment variable CLASSPATH's definition of Java's classpath by using the -cp or -classpath JVM command-line options.
How to Fix Errors Related to CLASSPATH in Java? How to Fix Errors Related to CLASSPATH in Java
ClassNotFoundException and NoClassDefFoundError are two of the most frequent errors and exceptions linked to the Java classpath that you may have seen when working with Java.
We've observed that many Java developers attempt to fix this mistake through trial and error; they just don't go deeper and attempt to determine the cause of these java classpath-related errors. They frequently believed that these two mistakes were the same.
Here is the reason for these Java classpath errors:
When a Java program dynamically attempts to load a Java class at runtime and cannot locate the necessary class file on the classpath, a ClassNotFoundException exception will be thrown. Learn more about runtime and dynamic errors here
When you attempt to load the JDBC driver using Class.forname("driver name") and encounter java.lang, this is a classic illustration of one of these issues.
In other words, Java encounters this issue when attempting to load a class using the forName() or loadClass() methods of the ClassLoader.
The most important point to keep in mind is that the Java classpath does not check for the presence of that class at compile time. As a result, even if such classes are missing from the Java classpath, your program will still compile properly; the only time it will crash is when you attempt to run it.
In contrast, ClassNotFoundException is an exception that may be recovered from, whereas NoClassDefFoundError is an error and is more serious. When a specific class was on the Java Classpath at compile time but not accessible at run time, a NoClassDefFoundError is generated.
Using log4j.jar for logging purposes while forgetting to add it to the classpath in Java during run-time is a classic illustration of this blunder. visit for additional information on logging into Java.
The class was present at compile time but was unavailable at run time, which is the crucial phrase here. This typically happens when a Java program invokes a method on a class that is a member of a library but not in the classpath.
Let's see how ClassNotFoundException and NoClassDefFoundError are different
When the class is not found at runtime, both the ClassNotFoundException and NoClassDefFoundError exceptions are thrown. They are connected to Java's classpath.
-
ClassNotFoundException error When attempting to load a class using the loadClass() or class.forName() methods at runtime and the required classes are not present in the classpath, a ClassNotFoundException is raised. This exception usually occurs when you try to launch an application without adding JAR files to the classpath. You must explicitly handle this checked Exception since it derives from the java.lang.Exception class. In Java, an exception also happens if a ClassLoader tries to access a class that is loaded by another ClassLoader or if there are two class loaders. You must be wondering what actually is classloader in Java. The Java Runtime Environment's Java ClassLoader dynamically loads Java classes into the JVM (Java Virtual Machine)
-
NoClassDefFoundError error When the class was present at compile time, the program was successfully compiled and linked, but the class was missing at runtime, a NoClassDefFoundError is generated. It is a variation of the LinkageError error. Linkage error happens when a class has some dependencies on another class and the later class changes after the compilation of the former class. NoClassFoundError is the result of a class being implicitly loaded because a method was called or a variable was accessed from that class. Debugging and determining the cause of this problem is more challenging. Therefore, in this situation, you should always look for the classes that depend on this class.
By the way, other causes for the NoClassDefFoundError include failures in static initializers and classes that are hidden from classloaders in the J2EE environment. For further information, see "3 approaches to fix NoClassDefFoundError in Java."
How Does ext Folder Work in Java?
Similar to the CLASSPATH, the ext directory also operates. A component of the class loading method is the ext directory. Java applications can access the classes included within JARs in the ext directory.
The major distinction between the Extension Mechanism and the CLASSPATH is shown in the following table:
Characteristics | CLASSPATH | Extension Mechanism |
---|---|---|
Class loading order | After bootstrap and extension loading, CLASSPATH would load | ext would load after bootstrap loading but also before CLASSPATH loading |
Specification | It is specified by name including the extension.jar and directory containing .class files. | In specific JAR files are loaded |
Scope | It is product-specific. The environment variable CLASSPATH contains all JREs on the host. | All JVMs are running in specific JRE java.ext.dirs. |
Package name | java.class.path is used to find the directories and JAR archives containing class files | java.ext.dirs is used to specify where the extension mechanism loads classes. |
Even if a file doesn't have the .jar extension, the method will still pick it up from the extension directory. This is implemented by allowing users to alter a jar's name and extension to something other than.jar when it is placed in a classpath directory. The asterisk (*) does not recognize it. The extension directory will not support this method.
Let's understand the execution process through an example
A.java
B.java
Make the A.java file compile. The compiled A.class file will be archived as an A.jar file. Put the compiled B.class file in a different directory than the JAR file.
We put the A.jar file in the directory C:JavaPrograms to show how to use the classpath. B will then access that JAR by using the wildcard (*) character.
We discovered that even after deleting A.class from the current directory, B could still load it. C:JavaProgram was specifically searched for to find the Java launcher. It is also possible to load a class without requiring it to be in the same directory or explicitly specified in the classpath.
Because all programs utilizing that JRE can see the same classes without explicitly putting them on the classpath, this is frequently regarded to as an advantage of adopting the extension technique.
What happens if we rename A.jar to A.backup in the same directory that CLASSPATH references. When we try the same, we run into a NoClassDefFoundError error since the CLASSPATH-reference does not have the .jar extension.
Difference between PATH and CLASSPATH
Let's see the difference between PATH and
CLASSPATH
PATH | CLASSPATH |
---|---|
PATH is an environment variable | CLASSPATH is an environment variable |
The operating system uses this to find executable files (.exe). | Application ClassLoader uses it to find the .class file |
There's a need to include the directory which contains .exe files. | All directories containing .class and JAR files must be included |
Once set, the PATH environment variable cannot be changed | The CLASSPATH environment variable can be overridden by the command-line options "-cp" or "-CLASSPATH" for the javac and java commands, respectively. |
Learn More
- Learn more about JVM here
- Learn more about Packages in Java here
- Learn about errors and exceptions in Java here
- Learn more about jar files in Java here
Explore Scaler Topics Java Tutorial and enhance your Java skills with Reading Tracks and Challenges.
Conclusion
- In order to encapsulate groups of classes, interfaces, and sub-packages we use packages in Java
- The location of all the necessary files that are utilized by the application is described by CLASSPATH
- A class that is required to be loaded is not found in the current directory or any of its subdirectories
- ClassNotFoundException and NoClassDefFoundError are two of the most frequent errors and exceptions linked to the Java classpath that you may have seen when working with Java
- Packages are an example of data encapsulation (or data-hiding)
- Java encounters this ClassNotFoundException issue when attempting to load a class using the forName() or loadClass() methods of the ClassLoader.