What are Java Default Parameters?
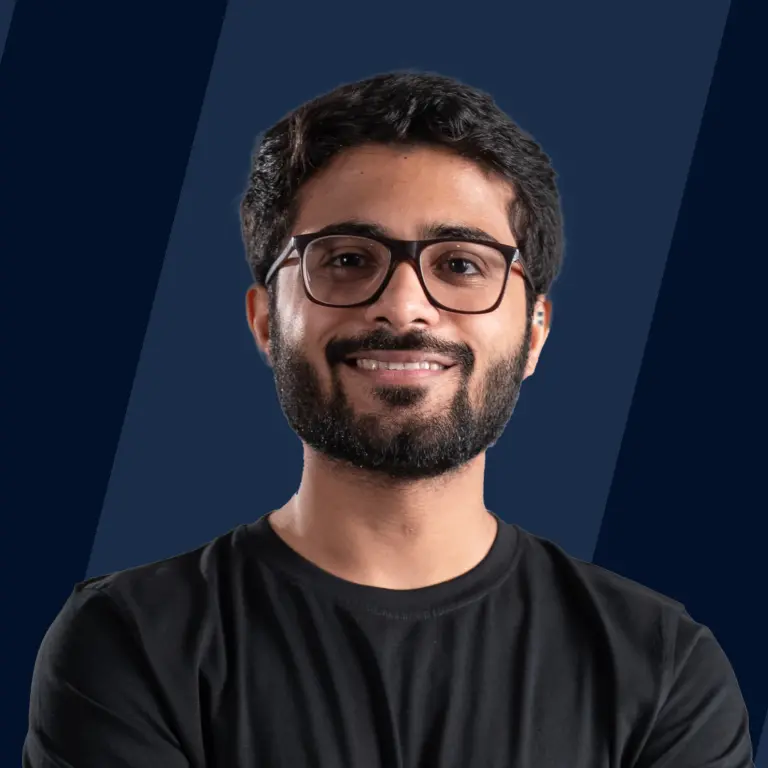
Suppose you have a function that can do the sum of three numbers. It means that function takes three integers as an argument and returns the sum of all three numbers. But what if we pass only two numbers as an argument? The third number should be automatically set to zero. In other words, the third number should have a default value of zero. Similarly, if we pass a single number as an argument, then the other two numbers should be automatically set to zero. To solve the above situation, we use the concept of Default Parameters.
Default Parameters: The value of the default parameter is used when no value is passed as an argument. In Java, default parameters are not supported. But there are some techniques that can help us in solving the above problem. In this blog, we will be covering commonly used techniques of simulating Java Default Parameters.
How to Simulate Java Default Parameters?
There are two ways to simulate Java Default Parameters. One way to simulate Java default parameters is by method overloading, and another is by builder patterns. There is a drawback with the method overloading technique. This approach doesn’t work if you have two optional arguments of the same data type, and any of them can be neglected. To use builder patterns, we use constructors and create a separate builder class for them.
Java Default Parameters Using Method Overloading
In this part, we will be using the method overloading technique to simulate Java default parameters.
Code
Output
Explanation
In the above example, we have created a class DefaultParameter that consists of two methods. The first Display method accepts two arguments first is int, and another is a string. The second Display method accepts a single argument int. The first method is overloaded by the second method (as both methods have the same name). The first time we call the Display method, we pass both int and string as an argument. In this case, the first Display method will be invoked. The second time we call the Display method, the overloaded method will be invoked as we have passed only a single argument (int) in the function. Then we call the first Display method within the overloaded method. We are setting the default value of the string (optional argument) to be null.
Java Default Parameters Allowing Nulls as Method Parameters
In this method, we create only one function that accepts all the possible arguments. Now, inside the body of the method we check if any argument has null value. If any argument has null value then we assign them a default value.
Code
Output
Explanation In the above example, we have made a universal function Display that accepts three arguments. The three arguments are integer, string, and boolean.
- The first time we call the Display function, we pass 10, “Hello”, and a null value as a set of arguments. Now the value of the third parameter is null. In the display function, we check whether all the arguments are null or not. Here the third argument is null, so by default, we are setting the value of the third argument to true.
- The second time we call the Display function, we pass 5, null, and false as a set of arguments. Here the string is null, and the default valur for the string is “empty string”.
- The third time we call the Display function, we pass null, “Soham”, true as a set of arguments. Here the first argument is null so the default is set to 0.
Drawbacks of Allowing Nulls as Method Parameters
You need to pass all the arguments in the function. Suppose a function accepts 5 arguments, three of them are optional and two are mandatory. You need to pass all the five arguments while calling the method. You cannot skip optional arguments while calling the method. Also, you need to know which parameters are nullable. We cannot know about the parameters just by looking at the function.
Java Default Parameters Using var-args in Java
var-args in Java allows you to pass a variable number of arguments in the function. This is a different approach to set Java default parameters. For instance, you have to get the sum of n numbers where n > 1. In this case, you can either overload the function for every value of n or pass n numbers through the function and check by allowing nulls as a parameter. But both these methods will be complicated. So, var-args provide you with a feature to pass a variable number of arguments into the function.
Code
Output
Explanation In the above example, we have passed the different number of arguments through the function. In the sum method, the variable ‘a’ will be a dynamic array of integers. It consists of all the arguments that are passed into the sum function. For iterating the ‘a’ we use for loop and store the sum in the variable.
Java Default Parameters as Empty String in Java
This method is basically a simple method where you can pass empty string as an argument. The empty string also holds null as a default value.
Code
Output
HelloWorld!
ILoveProgramming
~Soham
Explanation
In the above example, we have created a display function that accepts three strings as an argument. While calling the Display function we just pass an empty string as a default parameter if we want to omit the parameter.
Java Default Parameters Using Builder Pattern
The most efficient way to create objects of classes having optional parameters is by using a builder pattern. A builder object is created with the necessary arguments, and after that, setter-like methods are invoked to set the optional parameters. By invoking the build() method, the actual object is created. Let us see an example of a builder pattern to understand the working.
Code
Output
Explanation
In the above example, we have created a Vehicle class that has several parameters. Among all the parameters, “brand” is a mandatory parameter, and the rest of the parameters are optional. We are creating a builder class inside the Vehicle class. The builder class takes a single string as an argument (“brand” mandatory argument). Optional parameters are set by the setter methods inside the builder class. If a particular setter method is not called then the default value will be assigned to that parameter.
While creating the object of the Vehicle class, we did not pass two arguments i.e., color and price of the car. The default value of color is set to “Black” and the price to “above 1 crore”.
Java Default Parameters Using Optional
This approach is the same as the method overloading approach. In this approach, you will create multiple constructors. Each constructor accepts a different number of optional parameters. Basically, you will be creating a constructor with a combination of parameters. Let us understand this with the help of an example.
Code
Output
Explanation
In the above example, the firstname parameter is the mandatory parameter. Age and lastname are the optional parameters. We are creating a constructor for each permutation. The permutations are as follows:
- First Constructor: This constructor will be invoked when only the firstname is passed as an argument.
- Second Constructor: This constructor will be invoked when firstname and age are passed as an argument.
- Third Constructor: This constructor will be invoked when firstname and lastname are passed as an argument.
- Fourth Constructor: This constructor will be invoked when all three parameters are passed.
The firstname is the mandatory parameter, and the lastname and age are optional parameters.
Learn More
In the above article, we have used many concepts like method overloading, var-args, constructors, and builder class. To learn more about the above-mentioned topics, you can visit Scaler Topics.
Conclusion
- We can simulate Java default parameters using many methods, but we mainly use method overloading or builder pattern.
- It is not a good idea to simulate Java default parameters using Optional, because we have to make a constructor for every possible combination of optional parameters.
- We can also simulate Java default parameters by allowing nulls as method parameters. But in this approach, you need to know whether a particular parameter is nullable or not.
- Simulating Java default parameters allows you to pass a variable number of arguments into the function.