Java FileWriter
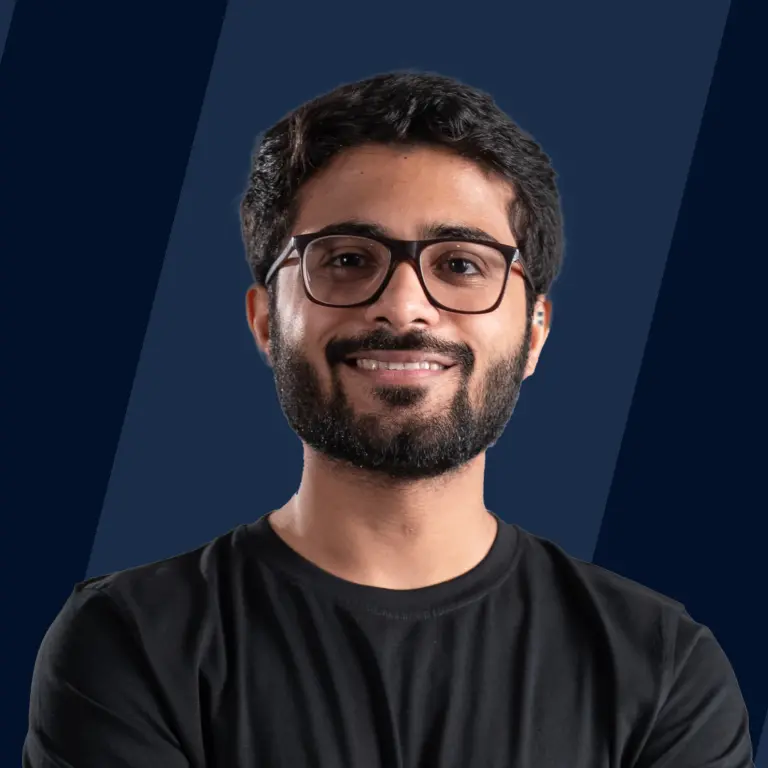
Overview
Data is written to files in character form using the Java FileWriter class of the java.io package. To write character-oriented data to a file, use the Java FileWriter class. It is a character-oriented class that Java uses to handle files.
Introduction to Java FileWriter
The java.io package contains the Java FileWriter class, this FileWriter is a sub-class of the java.io class It's designed for writing character streams. using its write() functions we can write character(s) or strings to a file Writer objects, like BufferedWriter or PrintWriter, offer superior performance and relatively flexible methods for the purpose of writing data, typically envelop FileWriters
Before we deep dive into it let's see what's a file in java.
Declaration
Let's see the declaration for Java.io.FileWriter class:
Create a FileWriter
We must import the Java.io.FileWriter package before we can create a file writer. Here is how to create the file writer after importing the package.
1. Using the Name of The File
Here, a file writer that will be connected to the file indicated by the name has been created
2. Using an Object of The File
Here, a file writer that will be connected to the file mentioned by the file's object has been constructed
The data are stored in the aforementioned example using standard character encoding.
Since Java 11, we can also define the character encoding type (UTF8 or UTF16)
In this case, the character encoding of the file writer has been specified using the Charset class.
Field Summary of Java FileWriter
Fields Inherited from Class Java.Io.Writer
the object that was utilized to synchronize actions on this stream. A character-stream object may, in the interest of efficiency, use another object in place of itself to safeguard crucial areas. As a result, a subclass ought to employ the object in this field instead of this or a synchronized method.
Constructor Summary of Java FileWriter
Constructor | Description |
---|---|
FileWriter(File) | Constructs a FileWriter given the File to write, using the platform's java. |
FileWriter(File, Boolean) | Constructs a FileWriter given the File to write and a boolean indicating whether to append the data written, using the platform's java. |
FileWriter(FileDescriptor fd) | Constructs a FileWriter given a file descriptor, using the platform's java. |
FileWriter(String fileName) | Constructs a FileWriter object given a file name. |
FileWriter(String fileName, boolean append) | Constructs a FileWriter object given a file name with a boolean indicating whether or not to append the data written |
Descriptor: A handle to the machine-specific structure representing an open file, an open socket, or another byte source or sink is provided by the FileDescriptor class. The handle may be in, out, or Create a FileInputStream or FileOutputStream to hold.
Constructor Detail of Java FileWriter
1. FileWriter(String fileName)
Parameters: fileName - of type string which is dependent on the system
Throws: IOException - this exception is thrown if the said file exists but is a directory rather than a normal file
2. FileWriter(String fileName, boolean append)
Here we've constructed a FileWriter object given a file name with a boolean indicating whether or not to append the data written.
Parameters: fileName - of type String, filename is system-dependent append - of type boolean, Data will be written at the file's end rather than its beginning if this value is true.
Throws: IOException - this exception is thrown if the said file exists but is a directory rather than a normal file
3. FileWriter(File file)
Constructs a FileWriter object given a File object.
Parameters: file - a File object to write to.
Throws: IOException - this exception is thrown if the said file exists but is a directory rather than a normal file
4. FileWriter(File file, boolean append)
gives a File object and creates a FileWriter object. Bytes will be written to the file's end rather than its beginning if the second parameter is true.
Parameters: file - a File object to write to append - if true, bytes will be written at the file's end rather than its start.
Throws: IOException - this exception is thrown if the said file exists but is a directory rather than a normal file
5. FileWriter(FileDescriptor fd)
This program constructs a FileWriter object associated with a file descriptor in java Parameters: fd - FileDescriptor object to write to.
Method Summary of Java FileWriter
Methods Inherited from Class Java.io.output Stream Writer
Character streams and byte streams are connected by the outputstream writer class. It uses a predefined charset to encode characters into bytes.
Declaration
Methods | Description |
---|---|
close() | This technique flushes the stream before closing it. |
flush() | This method flushes the stream |
String getEncoding() | This function returns the character encoding name that this stream is using. |
void write(char[] cbuf, int off, int len) | A subset of an array of characters is written using this approach. |
void write(int c) | This method writes a single character |
void write(String str, int off, int len) | This method writes a portion of a string. |
Methods Inherited From Class java.io.Writer
The java.io.Writer class is an abstract class. It is used to write to character streams.
Declaration
Methods
Method | Syntax | Description |
---|---|---|
append(char Sw) | java.io.Writer.append(char Sw) | appends a single character to the Writer |
append(CharSequence char_sq) | java.io.Writer.append(CharSequence char_sq) | appends specified character sequence to the Writer |
append(CharSequence char_sq, int start, int end) | java.io.Writer.append(CharSequence char_sq, int start, int end) | appends specified part of a character sequence to the Writer |
flush() | java.io.Writer.flush() | the Writer stream is flushed. All subsequent buffers in a chain will be flushed after one stream is flushed. |
close() | java.io.Writer.close() | closes character stream, flushing it first |
write(int char) | java.io.Writer.write(int char) | a single character is written to the character stream. The procedure ignores the remaining 16 higher bits of the "char" integer value, which include the characters that are being written. |
write(String str) | java.io.Writer.write(String str) | writes string to the character stream |
write(String str, int offset, int maxlen) | java.io.Writer.write(String str, int offset, int maxlen) | a portion of the string is written to the character stream. |
write(char[] carray) | java.io.Writer.write(char[] carray) | character stream is written with a character array. |
write(char[] carray, int offset, int maxlen) | java.io.Writer.write(char[] carray, int offset, int maxlen) | a portion of the character array is written to the character stream. |
Methods Inherited from Class java.lang.Object
There is an object class in the java.lang package. Every class in Java derives in some way from the Object class. A class is directly derived from an Object if it does not extend any other classes and is indirectly derived from other classes. As a result, all Java classes have access to the Object class methods. Therefore, in any Java program, the Object class serves as the root of the inheritance tree.
Methods | Description |
---|---|
toString() | The toString() enables the conversion of an object to a String and offers a String representation of an object. |
hashCode() | It gives back a hash value that can be used to look up items in a collection. When saving objects into hashing-related data structures like HashSet, HashMap, Hashtable, etc., the Java Virtual Machine (JVM) employs the hashcode method. |
equals(Object obj) | It contrasts the supplied item with "this" object (the object on which the method is called). It provides a general method to check for object equality. |
getClass() | To determine the actual runtime class of the object, it returns the class object of "this" object. It can also be used to obtain this class's metadata. |
finalize() | Just before an object is garbage collected, this method is called. When the garbage collector determines that there are no more references to an object, it is referred to as the garbage collector. |
clone() | It gives back a brand-new object that is an exact replica of the original.clone() method is described in Clone() |
notify() | awakens a single thread, waiting on the monitor of this object. |
notifyAll() | all the threads waiting on the monitor of this object are woken up. |
Java FileWriter Examples
Write(int c)
The single character specified by int c is written by this method.
The supplied byte value is written to the writer using Java's write(int) function of the Writer Class. The ASCII value of the byte value that was supplied as an integer value is used to specify this byte value. The parameter is this integer value.
Syntax:
Parameters: The ASCII value of the byte value to be written on the writer is the only required parameter for this method, and it is called ASCII.
Return Value: No value is returned by this method.
Let's see an example in Java
Let's see another example of Java FileWriter
Since the FileWriter class supports the AutoCloseable interface, we can try with resources while using it.
What is this AutoCloseable interface you ask?
A source or destination of the data that needs to be closed is referred to as a Closeable. When we need to release resources that are being held by objects, such as open files, we call the close() method. It is a crucial interface for stream classes. Closeable Interface, which is described in java.io, was first introduced in JDK 5. We are required to use the AutoCloseable interface starting with JDK 7+. An older interface called the closeable interface was created in order to maintain backward compatibility.
The Closeable interface extends the AutoCloseable interface therefore any class that implements Closeable also implements AutoCloseable.
Now how can we try with resources while using the AutoCloseable interface?
Support for try-with-resources — introduced in Java 7 — allows us to declare resources to be used in a try block with the assurance that the resources will be closed after the execution of that block.
The resources declared the need to implement the AutoCloseable interface.
Simply put, to be auto-closed, a resource has to be both declared and initialized inside the try:
Now that we've got that cleared up let's see an example in Java
Note: Because character A's decimal value is 65, the preceding program's fileWriter.write(65) function will write A to the file, and the same will happen for the remaining characters.
Write(String str, int off, int len)
This method writes an int len-sized chunk of String str from int off.
- str: String that is supposed to be written
- off: starting point for reading characters' offset
- len: total number of characters supposed to be written
If the len parameter's value is zero, no characters are written.
Write(char[] cbuf, int off, int len)
This method writes from int off to int len a subset of the characters from the array supplied by char[] cbuf.
- cbuf: It's a character array
- off: starting point for reading characters' offset
- len : Character count for writing
Write(char[] cbuf)
This method outputs the character array that cbuf has specified.
Write(String str)
This method writes the string value specified by str into the file.
Append(char c)
When c is the 16-bit character to be appended, this method appends the provided character to this writer.
Flush()
This technique cleans the stream. When the flush() method is used, the output file is immediately updated with the data.
Close()
Before closing the stream, this technique flushes it. Invoking the write() or flush() methods after the stream has been closed will result in an IOException being thrown. A stream that has already been closed has no impact.
Output:
Example Java: How To Append Text to A File with The FileWriter Class
To create a FileWriter instance with the append flag set to true, do as follows:
A sample data file
Let's say you want to append data to a file called checkbook.dat to examine how to attach text to the end of a file in Java. Let's say that checkbook.dat now has the following two entries:
The Java FileWriter and BufferedWriter classes make it simple to add data to the end of a text file. The right way to call the FileWriter constructor is the key component of the solution, in this case.
An "Append to file" illustration The following is an example mini-application that adds data to the checkbook.dat data file's end. Be mindful of how the FileWriter constructor is called:
The Java FileWriter constructor is called like this:
By using this straightforward constructor, you tell the computer to write to the file in append mode.
GetEncoding()
The type of encoding that is used to write the data can be determined using this method.
Output:
- Output1: Leaves out the character encoding information. Thus, the getEncoding() method returns the character encoding that is set to default.
- Output2: Identifies UTF11 as the character encoding. Consequently, the getEncoding() method returns the character encoding that was specified.
Write Performance
An array of characters can be written to a Java FileWriter more quickly than a single character. The speedup can increase by up to 10 times or more, which is quite a bit. So wherever possible, it is advised to use the write(char[]) method.
The specific speedup you experience depends on the computer's hardware and operating system (OS) when running Java code. The increase in speed depends on factors such as memory speed, hard disc speed, and buffer sizes, among others.
FileWriter vs FileOutputStream
FileOutputStream is used to write streams of raw bytes, whereas FileWriter is intended for writing streams of characters.
While FileOutputStream handles 8-bit bytes, FileWriter deals with 16-bit characters.
While FileOutputStream writes bytes to a file and does not accept characters or strings, it must be wrapped by OutputStreamWriter to accept strings while FileWriter can handle Unicode strings.
Overwriting vs. Appending the File
If a file already exists with the same name, you can choose whether to overwrite it or to append to it when you create a Java FileWriter. By selecting the FileWriter constructor you want to use, you can decide that.
Any existing file will be overwritten by the FileWriter function constructor, which only accepts one parameter, the file name:
The function Object() constructor of FileWriter also accepts a file name and a boolean as inputs. Whether to add or overwrite an existing file is indicated by the boolean. Following are two Java FileWriter examples demonstrating:
Java FileWriter & BufferedWriter
BufferedWriter can enhance FileWriter's performance. BufferedWriter writes text to a character-output stream while buffering individual characters, arrays, and strings to increase writing speed. The default buffer size is adequate for the majority of tasks, but it can also be modified.
In the illustration, we read the HTML code for Wikipedia's main page and write it to a file. The home page is big enough to take buffering into account.
The BufferedWriter receives the FileWriter as an argument. The text is then written using the BufferedWriter's write method.
The BufferedReader class also buffers the reading action.
Variable width character encoding is known as UTF-8. While UTF-8 can be as compressed as ASCII, it can also contain any unicode character with a slight increase in file size. Unicode Transformation Format is referred to as UTF. The "8" indicates that a character is designated by 8-bit blocks. A character can be represented by anywhere between 1 and 4 blocks.
Conclusion
- Data are written to files in character form using the Java FileWriter class of the java.io package
- Higher-level Writer objects, like BufferedWriter or PrintWriter, which offer superior performance and higher-level, more flexible methods to write data, typically envelop FileWriters
- java.io.Writer class is an abstract class. It is used to write to character streams.
- in any Java program, the Object class serves as the root of the inheritance tree.
- FileOutputStream is used to write streams of raw bytes, whereas FileWriter is intended for writing streams of characters.
- The increase in speed depends on factors such as memory speed, hard disc speed, and buffer sizes, among others.