Get Current Date and Time in Java
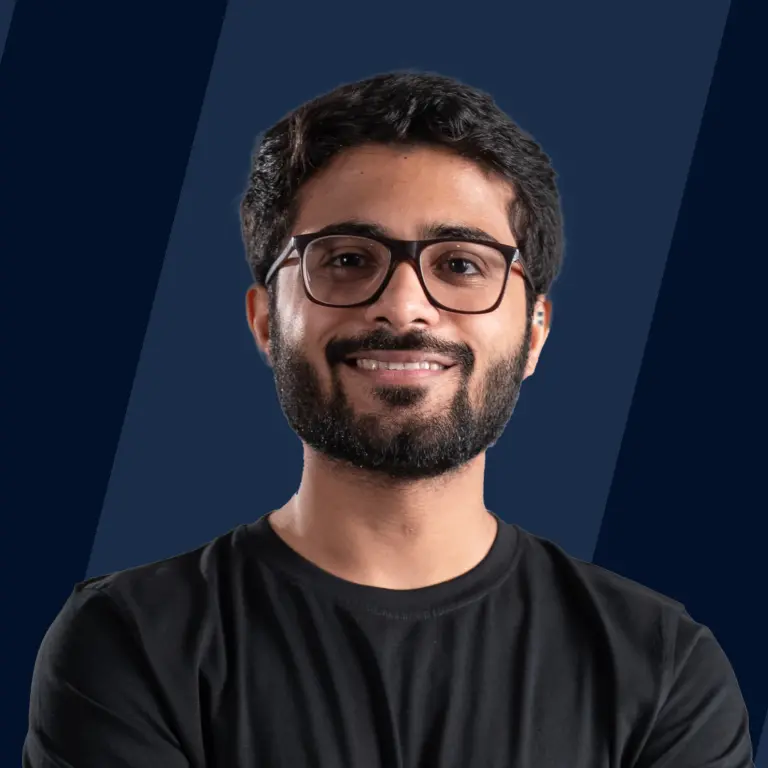
Overview
In this article, we are going to learn about how we can get current data and time in the Java programming language. In Java, there are several different ways to get current dates and times in Java. Let us learn and understand them in detail.
So let us now begin with the main agenda of our article, get the current date and time in Java.
Introduction
While coding, we might come to a situation, where we will be needing the current date and time, and for that, if we try to write its code manually, it will be quite difficult for us, and n, need to write several complicated codes too to accomplish this. Fortunately, In Java, we have various classes which can be used to achieve the same, preventing us from writing lengthy codes.
We will use the different methods of the classes to get the result in different formats. For instance, If we want our date and time in a simple default format we can use the LocalDateTime class, which will give the result as 2022-10-04T21:02:38.306696580 which is a default format as Year-Month-Day Hours:Minutes: Seconds. Milliseconds, but if we want our date time in some different pattern, let's say if we want our month part before the year part, we can also achieve that using the DateTimeFormatter class, which will help us in getting the date time in our own pattern choice, and will give the result as 10-2022-04 21:09:50.407 which is of format Month-Year-Day Hours:Minutes: Seconds.Milliseconds. Hence, in short, we can get data and time in Java in different formats using different classes.
Java Program to Get Current Date and Time
Now let us look at an example of getting the current date and time in Java programming language, for more clear understanding.
Abstract:
In this example, we will get the current date and time in Java using the default class in the Java programming language.
Code:
Output:
Explanation:
- In the above code, at first we are importing the LocalDateTime class, using which we will get the current date and time in Java.
- Inside the main method, we are calling the now() method of the LocalDateTime class, which will fetch the current date and time, and then we are storing the current date and time inside a variable of the LocalDateTime type.
- Then we are printing the current date and time. We can observe, the output format is in Year-Month-Day Hours:Minutes: Seconds. Milliseconds. We can also change the pattern of the format, which we will look into further.
Using java.time.format.DateTimeFormatter Class
Till now, we were just fetching the default current date and time using the LocalDateTime.now() method which was give the result as 2022-10-04T21:02:38.306696580 which is a default format as Year-Month-Day Hours:Minutes: Seconds. Milliseconds. But in case we want our date and time format to be different from the default one, we can use the DateTimeFormatter class which is included in JDK 1.8. Using the DateTimeFormatter class, we can fetch the current date and time in any pattern we want. For instance, if we want the format of the current date and time something like Day-Month-Year Hours:Minutes: Seconds. Milliseconds, we can achieve this using the DateTimeFormatter class. Let us understand this with the help of an example code in Java programming language.
Example:
Now let us look at an example of getting the current date and time in our format in Java programming language, for more clear understanding.
Abstract:
In this example, we will get the current date and time in Java in different patterns in the Java programming language.
Code:
Output:
Explanation:
- In the above code, at first we are importing the LocalDateTime class, using which we will get the current date and time in Java.
- Then we are also importing the DateTimeFormatter class, using which we will format our date in some different pattern.
- Inside the main method, we are calling the now() method of the LocalDateTime class, which will fetch the current date and time, and then we are storing the current date and time inside a variable of the LocalDateTime type.
- Then we are formatting our current date and time in some different pattern, using the ofPattern() method of the DateTimeFormatter class. Inside the ofPattern() method we are sending our pattern structure as a parameter.
- Then we are printing the formatted current date and time. We can observe, the output is in Day-Month-Year Hours:Minutes: Seconds. Milliseconds format.
Using java. text.SimpleDateFormat Class
We can also get the current date and time in Java in our own format choice using the SimpleDateFormat class. But using the SimpleDateFormat class is an old method.
Example:
Now let us look at an example of getting the current date and time in our format in Java programming language, for more clear understanding.
Abstract:
In this example, we will get the current date and time in Java in different patterns using the SimpleDateFormat class in Java programming language.
Code:
Output:
Explanation:
- In the above code, at first we are importing the Date class, using which we will get the current date and time in Java.
- Then we are also importing the SimpleDateFormat class, using which we will format our date in some different pattern.
- Inside the main method, we are creating the instance of the Date class, which will fetch the current date and time.
- Then we are formatting our current date and time in some different pattern, by creating the instance of the SimpleDateFormat class, where we are sending our pattern structure as a parameter of the SimpleDateFormat constructor.
- Then we are printing the formatted current date and time. We can observe, the output is in Day/Month/Year Hours:Minutes: Seconds. Milliseconds format.
Using java. util.Date Class
In the above code, you can observe we have to get ctheucurrentdate and time in Java using the java. util.Date class. The java.util.Date class is used to fetch the current date and time. Different constructors and methods are provided by it to deal with the date and time in Java.
Interfaces like Serializable, Cloneable, and Comparable<Date> are implemented by this java.util.Date class. The java.util.Date class is inherited by the java.sql.Date, java.sql.Time, and java.sql.Timestamp interfaces.
However, most of the java.util.Date class constructors and methods are deprecated.
Example:
Now let us look at an example of getting the current date and time in Java using the java.util.Date class in Java programming language, for more clear understanding.
Abstract:
In this example, we will get the current date and time in Java using the java.util.Date class in the Java programming language.
Code:
Output:
Explanation:
- In the above code, at first we are importing the Date class, using which we will fetch the current date and time.
- Inside the main method, we are creating the instance of the Date class, which will fetch the current date and time.
- Then we are printing the current date and time.
Using java. util.Calendar Class
java. util.Calendar class is an abstract class, which has methods for converting dates between a given moment in time and a variety of calendar variables like MONTH, YEAR, HOUR, etc. It implements the Comparable interface and derives from the Object class.
getInstance() method : It is a method of the java.util.Calendar class, which is used to retrieve an instance of the calendar based on the current time zone defined by the Java runtime environment.
getTime() method : It is a method of the java.util.Calendar class, which returns the instance of java.util.Date class.
add() method : It is a method of the java.util.Calendar class, using which the provided calendar field is updated with the specified (signed) amount of time.
Example:
Now let us look at an example of getting the current date and time using the java.util.Calendar class in Java programming language, for more clear understanding.
Abstract:
In this example we will fetch the current date and time using the java.util.Calendar class in the Java programming language. Along with that, we will also fetch some other dates in the calendar too.
Code:
Output:
Explanation:
- In the above code, at first we are importing the Calendar class, using which we will fetch the current date and time.
- Inside the main method, we are creating the instance of the Calendar class according to the current time zone using the getInstance() method.
- Then we are printing the current date and time using the getTime() method.
- Then we are also getting the calendar date of 15 days ago, using the add() method. There are two parameters inside the add() method they are: the field which we need to update (for ex: Date, Month, Y, ear), and the amount by which we will update.
- Then we are also fetching the calendar date of 4 months later and 2 years later using the same add() method.
Using java. time.LocalDate class
The java. time.LocalDate class is an immutable class, which is used to fetch the date with a default format of yyyy-mm-dd. The ChronoLocalDate interface is implemented by it, and the Object class is inherited by it.
LocalDate.now(): The LocalDate.now() method is used to return the instance of the LocalDate class. We will get the current date if we try to print the instance of the LocalDate class.
**Example **
Now let us look at an example of getting the current date using the java.time.LocalDate class in Java programming language, for more clear understanding.
**Abstract **
In this example, we will fetch the current date using the java.time.LocalDate class in the Java programming language.
**Code **
Output:
explanation:
- In the above code, at first we are importing the LocalDate class, using which we will fetch the current date.
- Inside the main method, we are creating the instance of the LocalDate class using the now() method, and storing the current date inside a date variable ofLatheeoxalate types.
- Then we are fetching yesterday's date using the minusDays() method, inside which we are giving the amount by which our date is to be subtracted as a parameter. Then we are storing yesterday's date inside a yesterday variable of LocalDate type.
- Then we are fetching tomorrow's date using the plus days) method, inside which we are giving the amount by which our date is to be added as a parameter. Then we are storing tomorrow's date inside a tomorrow variable of LocalDate type.
Using java. time.LocalTime class
The java. time.LocalTime class is an immutable class, which is used to fetch the time with a default format of HH:mm: ss. zzz.
LocalTime.now(): The LocalTime.now() method is used to return the instance of the LocalTime class. We will get the current time if we try to print the instance of the LocalTime class.
Example:
Now let us look at an example of getting current time using the java.time.LocalTime class in Java programming language, for more clear understanding.
Abstract:
In this example, we will fetch the current time using the java.time.LocalTime class in the Java programming language.
Code:
Output:
Explanation:
- In the above code, at first we are importing the LocalTime class, using which we will fetch the current time.
- Inside the main method, we are creating the instance of the LocalTime class using the now() method, and storing the current time inside a time variable of the LocalTime type.
- Then we are printing the time. The format is HH:mm: ss. zzz.
Using java. time.LocalDateTime class
The java. time.LocalDateTime class is an immutable class, which is used to fetch the date and time with a default format of yyyy-MM-dd-HH-mm-ss. zzz. The ChronoLocalDate interface is implemented by it, and the Object class is inherited by it.
LocalDateTime.now(): The LocalDateTime.now() method is used to return the instance of the LocalDateTime class. We will get the current date and time if we try to print the instance of the LocalDateTime class.
Example:
Now let us look at an example of getting the current date and time using the java.time.LocalDateTime class in Java programming language, for more clear understanding.
Abstract:
In this example, we will fetch the current date and time using the java.time.LocalDateTime class in the Java programming language.
Code:
Output:
Explanation:
- In the above code, at first we are importing the LocalDateTime class, using which we will fetch the current date and time.
- Inside the main method, we are creating the instance of the LocalDateTime class using the now() method, and storing the current date and time inside a date variable of LocalDate type.
- Then we are printing the date and time. The format is yyyy-MM-dd-HH-mm-ss. zzz.
Using java. time.ZonedDateTime
The java. time.ZonedDateTime class is an immutable class, which is used to fetch the date and time with a time zone. The ChronoLocalDate interface is implemented by it, and the Object class is inherited by it.
All the date and time fields are stored to the accuracy of nanoseconds, and also the time zone with a zone offset which is used to manage cryptic local date-time using the ZonedDateTime class.
ZonedDateTime.now(): The ZonedDateTime.now() method is used to rreturnthe instance of the ZonedDateTime class. We will get the current date and time in the default time zone from the system clock if we try to print the instance of the ZonedDateTime class.
Example:
Now let us look at an example of getting the current date and time using the java.time.ZonedDateTime class in Java programming language, for more clear understanding.
Abstract:
In this example, we will fetch the current date and time using the java.time.ZonedDateTime class in the Java programming language.
Code:
Output:
Explanation:
- In the above code, at first we are importing the ZonedDateTime class, using which we will store the current date and time field along with the time zone.
- Inside the main method, we are storing the date time field along with the zone inside a zone variable of ZonedDateTime type.
- Then we are printing the zone.
Using java. time.Clock class
Using the time zone, the current date and time are accessed using the java. time.Clock class. Object class is inherited by this java. time.Clock class.
Using the Clock class is not necessary because all date-time classes provide a now() function that uses the system clock in the default time zone. The Clock class's purpose is to enable you to connect an additional clock whenever you need one. Applications use an object to retrieve the current time rather than a static function. It makes the testing procedure easier. A Clock can be used as a parameter in a method that needs the current instant.
Example:
Now let us look at an example of getting the current date and time using the java.time.Clock class in Java programming language, for more clear understanding.
Abstract:
In this example we will fetch the current date and time using the java.time.Clock class in the Java programming language.
Code:
output:
**Explanation **
- In the above code, at first we are importing the Clock class, using which we fetch the current date and time.
- Inside the main method, we are using the clock. systematic).instant()` method which will return the current date and time. We are then storing the date and time in a variable of Clock type.
- Then we are printing the current date and time. The format is `yyyy-MM-dd-HH-mm-ss. z zzz.
Using java .time.Instant
The specific moment on the timeline is represented by the java. time.Instant class. The object class is inherited by it and it also implements the Comparable interface.
Instant. now(): the current instant from the system clock is obtained by this method.
Example:
Now let us look at an example of getting the current date and time using the java.time.Instant class in Java programming language, for more clear understanding.
Abstract:
In this example, we will fetch the current date and time using the java.time.Instant class in the Java programming language.
Code:
Output:*
**Explanation **
- In the above code, at first we are importing the Instant class, using which we will fetch the current date and time.
- Inside the main method, we are using the instant now()` method which will return the current date and time. We are then storing the date and time in a variable of Instant type.
- Then we are printing the current date and time. The format is yyyy-MM-dd-HH-mm-ss. zzz.
Using java. time.OffsetDateTime
The java. time.OffsetDateTime class is an immutable class, which is used to fetch the date and time with an offset. The object class is inherited by it and it also implements the Comparable interface.
The date and time field to the accuracy of nanoseconds are stored in the OffsetDateTime class.
Example:
Now let us look at an example of getting the current date of the month using the java.time.OffsetDateTime class in Java programming language, for more clear understanding.
Abstract:
In this example, we will fetch the current date of the month using the java.time.OffsetDateTime class in Java programming language.
Code:
Output:
**Explanaion **
- In the above code, at first we are importing the OffsetDateTime class, using which we will fetch the current date and time.
- Inside the main method, we are using the OffsetDateTime.now() method which will return the current date and time. We are then storing the date and time in a variable of OffsetDateTime type.
- Then we are printing the current date and time. The format is yyyy-MM-dd-HH-mm-ss. zzz.
- We are also getting the day of the month using the getDayOfMonth() method.
Using java. time.Year and java. time.Monday
**java. time. Yea ** java.t itimeYear class is an immutable class, which is used to fetch the year. The object class is inherited by it and it also implements the Comparable interface.
Example:
Now let us look at an example of getting the current year using the java.time.Year class in Java programming language, for more clear understanding.
Abstract:
In this example, we will fetch the current year using the java.time.Year class in Java programming language.
Code:
Output:
Explanation:
- In the above code, at first we are importing the java. time.Year class, using which we will fetch the current year.
- Inside the main method, we are using the Year. now() method which will return to the current year. We are then storing the year in a variable of Year type.
- Then we are printing the current year. The format is yy.
java. time.Monday: java. time. Monday class is an immutable class, which is used to fetch the combination of a month and day-of-month. The object class is inherited by it and it also implements the Comparable interface.
Example:
Now let us look at an example of getting the current month and day-of-month using the java.time.MonthDay class in Java programming language, for more clear understanding.
Abstract:
In this example, we will fetch the current month and day-of-month using the java.time.MonthDay class in Java programming language.
Code:
Output:
Explanation:
- In the above code, at first we are importing the java. time.Monday class, using which we will fetch the current month and day-of-month.
- Inside the main method, we are using the MonthDay.now() method which will return the current month and day of the month. We are then storing the month and day-of-month in a variable of MonthDay type.
- Then we are printing the current month and day-of-month. The format is --MM--DD.
Using java.sql.Date class
We can print the current date in Java, using the instance of java.sql.Date class. with this class we can only print the date, but not the time. Generally, the date which is fetched with this instance, we store in the database.
Example:
Now let us look at an example of getting the current date using the java.sql.Date class in Java programming language, for more clear understanding.
Abstract:
In this example, we will fetch the current date using the java.sql.Date class in Java programming language.
Code:
Output:
Explanation:
- In the above code, at first we are importing the java.sql.Date class, using which we will fetch the current date.
- Inside the main method, we are creating the instance of Date.
- Then we are printing the current date. The format is YYYY-MM-DD.
Conclusion
In this article, we learned about how to get current date and time in java. Let us recap the points we discussed throughout the article:
- In Java there are several different ways to get current dates and times Java
- If we want our date and time format to be different from the default one, we can use the DateTimeFormatter class which is included in JDK 1.8.
- We can also get the current date and time in Java in our own format choice using the SimpleDateFormat class. But using the SimpleDateFormat class is an old method.
- The java.util.Date class is used to fetch the current date and time.
- java. util.Calendar class is an abstract class, which has methods for converting dates between a given moment in time and a variety of calendar variables like MONTH, YEAR, HOUR, etc.
- The java. time.LocalDate class is an immutable class, which is used to fetch the date with a default format of yyyy-mm-dd.
- The java. time.LocalTime class is an immutable class, which is used to fetch the time with a default format of HH:mm: ss. zzz.
- The java. time.LocalDateTime class is an immutable class, which is used to fetch the date and time with a default format of yyyy-MM-dd-HH-mm-ss. zzz.
- The java. time.ZonedDateTime class is an immutable class, which is used to fetch the date and time with a time zone.
- Using the time zone, the current date and time are accessed using the java. time.Clock class.
- The specific moment on the timeline is represented by the java. time.Instant class.
- The java. time.OffsetDateTime class is an immutable class, which is used to fetch the date and time with an offset.
- java. time.Year class is an immutable class, which is used to fetch the year.
- java. time.Monday class is an immutable class, which is used to fetch the combination of a month and day-of-month.
- We can print the current date in Java, using the instance of java.sql.Date class.