Java Hello World Program
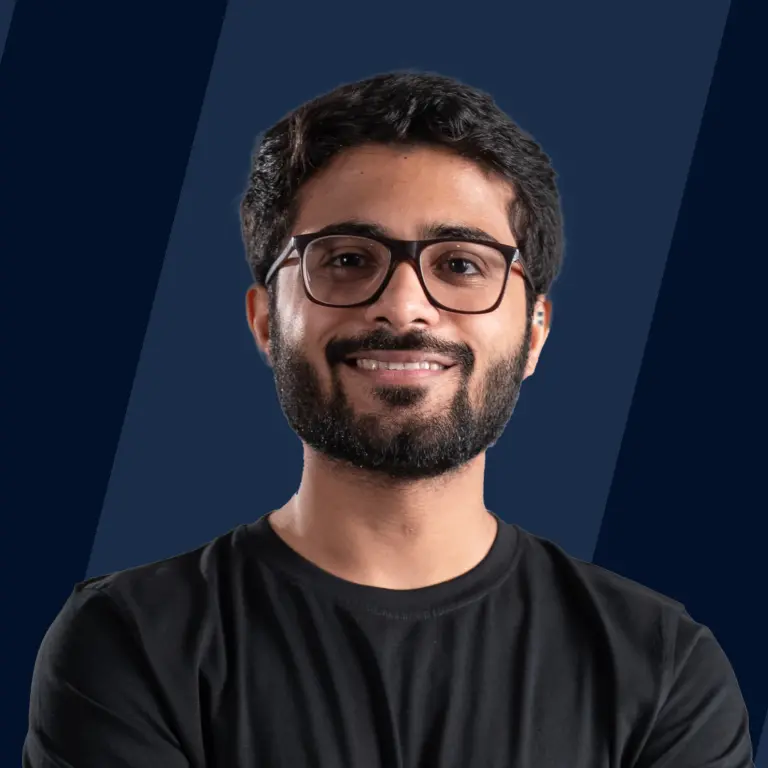
Overview
The starting point of learning any programming language is to write a Hello World Program in that language. This is necessary as the Hello World program helps the programmer understand the basic structure of a program in that language. In this article, we will write a Hello World program in Java.
Introduction to Java Hello World Program
The first step to learning any programming language is to learn how to print “Hello World” using that language, especially when learning to program for the first time. This article will teach us how to write a Hello World program in Java. ' What are the various prerequisites to running the Java Hello World program` on our local computer?
The process of Java programming can be simplified in three steps:
- Using a text editor, write the program's code, then save it as HelloWorld.java.
- Type "javac HelloWorld.java" to compile it in the terminal window.
- Enter "java HelloWorld" to run (or execute) it in the terminal window.
Prerequisites
- Installing JDK from Oracle
- Setting the path of jdk/directory. [C:\Program Files\Java\jdk-13\bin]
- Installing an IDE (Optional but recommended).
Note - You can also execute your program in an online IDE if you don’t want to set up JAVA on your local machine.
We want to print Hello, World! on our screen/console using Java, so our flow will be -
- Writing Program
- Compiling it
- Executing it
And that’s it 😁.
Example of Java Hello World Program
Here’s what a Java Hello world program looks like:
Code Explanation:
class Main -
‘Class‘ keyword in Java is used to declare a new class. We have declared a Main class in the above program. It is necessary to create a class in a Java program and all of our code should be inside a class.
This is because Java is a "pure" object-oriented programming language; therefore, everything extends from an Object class.
For example -
‘class ClassName’ is used to create a class named ‘ClassName’.
public static void main() -
Every Java program must have a main function. The compiler starts executing the program only from the main function.
Let’s see the significance of all the keywords that are used to declare the main function.
public -
It is used so that JVM (Java virtual machine) can execute the program without any hassle. In other words, we can understand that when the function is public, it will be visible to JVM so that it can invoke the function.
static -
It is used to call the main function without creating an object of the class. The main advantage of using the static keyword is that it doesn't require creating an object to invoke the main function, which ultimately saves memory.
void -
'void' is a keyword in Java that specifies that the function doesn't return anything. It is also the return type of the main function. This means the Java program terminates as soon as the main function goes out of scope.
main() -
It is the program's starting point. The program's execution starts from here. This means that whenever code is executed, the compiler starts the execution from the main function. Any Java program must contain the main function. Also, it's the default name in JVM.
String args[] -
It is used to pass arguments to the main function. As of now, one doesn’t need to worry about this.
Comments -
Double slashes (//) are used to write single-line comments in Java. While for multiline comments, /* …. */ is used.
Alternatively, you can comment on each line individually by double slashes.
For example -
System.out.println(“Hello world”); -
This line is used to print Hello World on the screen. The text written inside the double quotes is known as a string in Java. println(), which is a method Printstream class, is responsible for printing Hello world on the screen, while System is the class that provides access to the console.
Compilation:
Now that we have written our program, we need to compile it, i.e., convert human-readable code to machine code, to run the code.
- Open any text editor
- Write the given program
- Save the file as Main.java
- Open terminal/command prompt and type javac Main.java
Execution:
To get the output of the compiled program, you must type java Main.java in the command prompt.
Note - Ensure you are in the same directory in your source file.
We can see on executing the Main.java file; we get “Hello, World!” as our output.
Important Points for Java Hello World Program
- Java is a case-sensitive language, so please check the case of the text if you are getting any errors.
- JDK should be installed on your PC to compile and run a Java program beforehand.
Conclusion
- In this blog, we have seen a simple hello world program in Java. We learned how to print hello world in java.
- We sincerely hope that this blog gave you a quick glimpse of Java's Hello World program, including the steps to write, compile, and execute it. We hope that now you are confident enough to start your journey with Java by writing a simple Java program to print Hello World.
- If you have a keen interest in software engineering, programming, Data Structures, and Algorithms and want to upskill yourself to stand out of the crowd, do check out Scaler. There, you will get video lectures, one-on-one guidance from top-notch software engineers working in your dream company, and a certification.