Java InputMismatchException
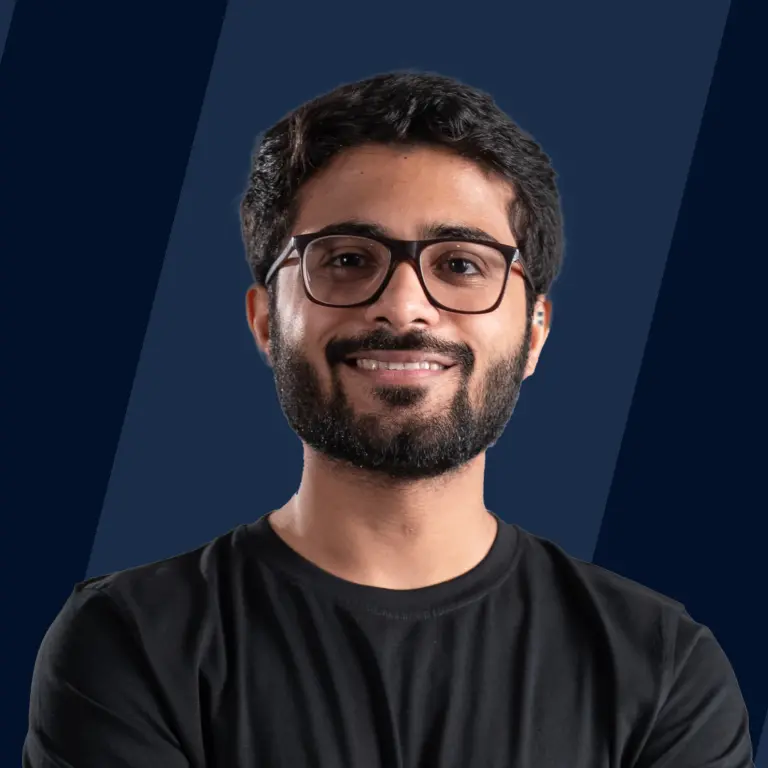
InputMismatchException is the most common exception that is experienced in Java. It is an unchecked exception since it resides in the subclass of java.lang.RuntimeException.
Introduction to Java InputMismatchException
The InputMismatchException is considered a runtime exception in Java since it is thrown by a scanner class or object to make the developer understand that the object does not match the expected type and is out of range. This exception is mostly thrown during the runtime when the developer passes input. Since it is an unchecked exception, the InputMismatchException doesn’t need to be declared in the constructor or method of the throws class. The hierarchy for the exception java.util.InputMismatchException is as follows:
It also provides all the methods that are provided by java.lang.Throwable class and the java.lang.Object class because it is the subclass of both of these classes.
Declaration
Constructor Summary of Java InputMismatchException
There are two constructors provided by the InputMismatchException class
Method | Description |
---|---|
InputMismatchException() | It constructs the exception, InputMismatchException with an error message as null in a string since it’s a default constructor. |
InputMismatchException(e) | It is known as a parameterized constructor of the exception InputMismatchedException that saves the reference to the error message e for retrieving the method getMessage(). |
Method Summary of Java InputMismatchException
There are two types of methods available in InputMismatchException of java. The first is inherited from java.lang.Throwable class and the one derived from java.lang.Object class. Following are the methods for this exception class in java:
Method name | Method declaration | Description |
---|---|---|
addSuppressed | public final void addSuppressed(Throwable exception) | This method is used to append the exception that is specified to the exception that is suppressed for delivering this exception. It is a thread-safe method and is called automatically with the try-catch statement. |
fillInStackTrace | public Throwable fillInStackTrace() | It is used for filling the execution stack trace. It also returns the throwable instance of the reference. |
getCause | public Throwable getCause() | It is used to return the cause of null or throwable in case the cause is not known. |
getLocalizedMessage | public String getLocalizedMessage() | It is used to create and return the localized description of the throwable. |
getMessage | public String getMessage() | It is used to return the detailed message of the typed string of this throwable. |
getStackTrace | public StackTraceElement[] getStackTrace() | It is used to return the array of a stack with all the trace elements that represent the stack trace. |
getSuppressed | public final Throwable[] getSuppressed() | It returns all the suppressed exceptions in an array. |
initCause | public Throwable initCause(Throwable cause) | It is used to initialize the cause of the throwable to a specified value and then return a reference to this throwable instance. |
printStackTrace | public void printStackTrace() | This method is used for printing the stack trace for this object throwable on the error output stream of the field System.err |
setStackTrace | public void setStackTrace(StackTraceElement[] stackTrace) | It is used for setting the stack trace elements. |
toString | public String toString() | It is used to return the string representation of this throwable object. |
clone | protected Object clone() throws CloneNotSupportedException | It is used for creating and returning the copy of the specified object. |
equals | public boolean equals(Object obj) | It is used to check if two objects are equal or not, returns true if same, or else false. |
finalize | protected void finalize() throws Throwable | The garbage collector generally calls it to check if there are no more references to an object. |
getClass | public final Class<?> getClass() | It is used for returning the class object that represents the object of the class in runtime. |
hashCode | public int hashCode() | It is used to return the hash code value of an object. |
notify | public final void notify() | It invokes a single thread waiting on the object. |
notifyAll | public final void notifyAll() | It invokes all the thread waiting on the object. |
wait | public final void wait() throws InterruptedException | It is used to make the current thread wait until another thread invokes the notify method. |
Java InputMismatchException Examples
Write a java program to show InputMismatchException at the output.
Output
Explanation: In this example, the InputMismatchException is thrown when, instead of a number, a string is entered, which is “Hello”. This is because the scanner input was expecting an integer or a number in the input and not a string value, which was why the InputMismatchException was thrown at the output.
How to Avoid InputMismatchException?
In order to avoid InputMismatchException, make sure that the input that is entered for the scanner object is of a valid type, as mentioned in the code. If this exception is thrown, then the format or the type of input data should be checked and fixed to execute the program correctly. For instance, in the above example, if an integer instead of a string, “Hello,” is given as input to the scanner object, then the InputMismatchException does not occur, and the program is executed properly.
Conclusion
- InputMismatchException is the most common exception experienced in Java. It is an unchecked exception since it resides in the subclass of java.lang.RuntimeException.
- The exception InputMismatchException is considered a runtime exception in java since it is thrown by a scanner class or object to make the developer understand that the object does not match the expected object type and that it is out of range. This exception is mostly thrown during the runtime when the developer passes input.
- The InputMismatchException doesn’t need to be declared in the constructor or method of the throws class since it is an unchecked exception.