Java.lang.ExceptionInInitializerError
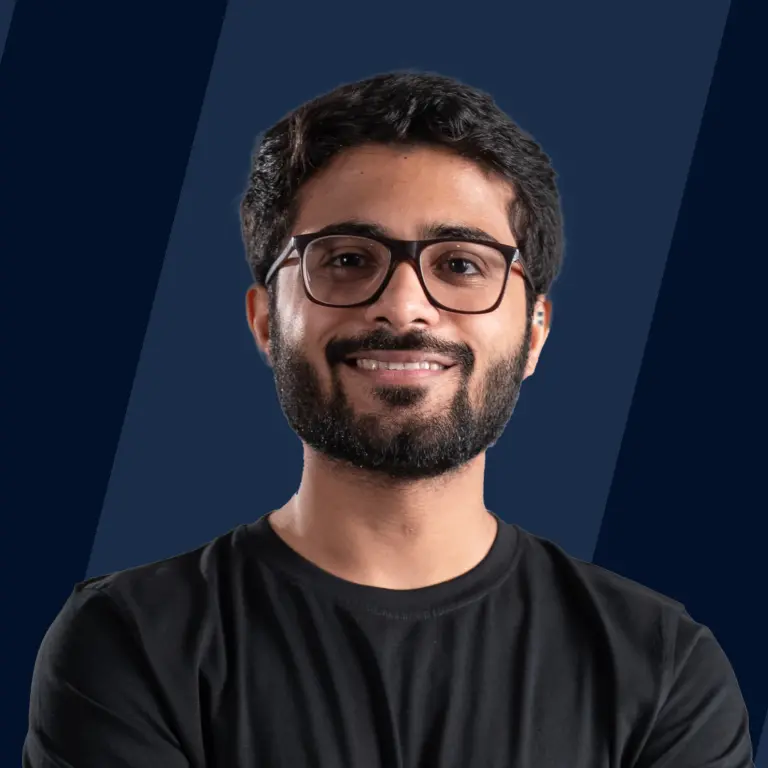
Overview
In Java, we often need static members in the class to use them even before the instantiation of a class. But RuntimeException (unchecked exceptions) may be encountered while we initialize the static variables, possibly in a static initializer block. It is when, during the class loading, the JVM throws a java.lang.ExceptionInInitializerError.
Introduction to java.lang.ExceptionInInitializerError
java.lang.ExceptionInInitailizerError is an unchecked exception as it is a child class of Error. After a successful compilation, the Java Virtual Machine (JVM) performs dynamic loading, linking and initializing of classes and interfaces. The process is called class loading. The evaluation of all static initializer blocks and static variable assignments present in compiled code is part of this process. Hence JVM throws a java.lang.ExceptionInInitializerError, if, during the evaluation, an exception occurs. As a result, that particular class is also not loaded in the memory.
To take this further, when JVM fails to load a class, JVM throws another very common exception - NoClassDefFoundError. In that case, programmers should carefully inspect the logs before looking into the classpath, path and java.library.path because the reason might be a java.lang.ExceptionInInitializerError. The JVM also wraps an unchecked exception (within the instance of ExceptionInInitializerError that maintains a reference to it as the root cause) that occurred inside a static initializer block or static variable assignment.
Declaration
ExceptionInInitializerError is a part of java.lang package. If this error occurs then the class is not loaded in JVM memory because it is a child class of LinkageError.
It signals that an unexpected exception has occurred in a static initializer. java.lang.ExceptionInInitializerError is thrown to indicate that an exception occurred during the evaluation of a static initializer or the initializer for a static variable.
Constructors of java.lang.ExceptionInInitializerError
Constructor | Description |
---|---|
public ExceptionInInitializerError() | Constructs an ExceptionInInitializerError with null as its detail message string and with no saved throwable object. |
public ExceptionInInitializerError(String s) | Constructs an ExceptionInInitializerError with the specified detail message string. |
public ExceptionInInitializerError(Throwable thrown) | Constructs a new ExceptionInInitializerError class by saving a reference to the Throwable object thrown for later retrieval by the getException() method. |
Constructors in Detail
Constructs an ExceptionInInitializerError with null as its detail message string and with no saved throwable object. A detail message is a String that describes this particular exception.
Constructs a new ExceptionInInitializerError class by saving a reference to the Throwable object thrown for later retrieval by the getException() method. The detail message string is set to null.
Parameters: thrown : The exception thrown
Constructs an ExceptionInInitializerError with the specified detail message string. A detail message is a String that describes this particular exception. The detail message string is saved for later retrieval by the Throwable.getMessage() method. There is no saved throwable object.
Parameters: s : The detail message
Methods of java.lang.ExceptionInInitializerError
Method | Syntax | Description |
---|---|---|
getCause() | public Throwable getCause() | Returns the cause of this error (the exception that occurred during a static initialization that caused this error to be created). |
getException() | public Throwable getException() | Returns the exception that occurred during a static initialization that caused this error to be created. |
Methods in Detail
a. getCause() Method
It returns the exception that occurred during a static initialization that caused this error to be created. This method predates the general-purpose exception chaining facility. The Throwable.getCause() method is now the preferred means of obtaining this information.
Return Value: The saved throwable object of this ExceptionInInitializerError, or null if this ExceptionInInitializerError has no saved throwable object.
b. getException() Method
It overrides the Throwable.getCause() method and returns the cause of this error (the exception that occurred during a static initialization that caused this error to be created).
Return Value: The cause of this error or null if the cause is nonexistent or unknown.
java.lang.ExceptionInInitializerError Examples
The following examples display the outputs received on running java FileName after compiling using javac FileName.java command.
1. Unchecked Exception during Static Variable Initialization
Output:
Explanation: The above example shows how an unchecked exception (dividing the number 10 by resulting in ArithmeticException) during static variable assignment, results in java.lang.ExceptionInInitializerError error.
2. Unchecked Exception inside Static Initializer Block
a. Example Illustrating NullPointerException
Output:
Explanation: The example illustrates another unchecked exception that leads to java.lang.ExceptionInInitializerError. Here, we declare a static string variable s but do not initialize it. In the static block, we try to get the length of the uninitialized string variable, which results in NullPointerException, which is wrapped inside the instance of ExceptionInInitializerError and thrown by JVM.
b. Example Illustrating IndexOutOfBoundsException
Output:
Explanation: This example is similar to the previous one. But, here, we create a new empty ArrayList of strings and store its reference within a static variable. We create another static variable to hold the list's first item. We initialize the variable inside the static initializer block by fetching the first item from the list. But, since the list is empty, an IndexOutOfBoundsException is thrown by JVM in runtime wrapped inside the java.lang.ExceptionInInitializerError instance.
3. Checked Exception inside Static Initializer Block
Java doesn't allow throwing checked exceptions from a static block. However, one can handle those exceptions using a try-catch block and wrap them inside the ExceptionInInitializerError instance. It is a good practice as we follow the design principles of the Java language.
Output: i. If the ScalerTopics.txt is available
ii. If the ScalerTopics.txt is not available
Explanation: In this example, we declare a static FileInputStream variable instantiated inside the static initialization block. A file named "ScalerTopics.txt" may or may not exist. Hence, the constructor for FileInputStream possibly throws a checked exception - FileNotFoundException. But such checked exceptions are not allowed inside static blocks; therefore, one might get a compile-time error if the exception is unhandled. Hence, we handle it using try-catch and throw that exception, indirectly, wrapped inside an instance of java.lang.ExceptionInInitializerError. By this, we also conform ourselves to follow the design principles.
Causes of java.lang.ExceptionInInitializerError
The ExceptionInInitializerError indicates that an unexpected exception has occurred while:
- Evaluation of a static initializer block
- Initialization of a static variable
By default, the unexpected exception occurred is an unchecked exception. The unchecked exceptions are also called runtime exceptions in Java.
How to Resolve java.lang.ExceptionInInitializerError?
The resolution of java.lang.ExceptionInInitializer can be done by ensuring that:
- Static initializer of a class doesn't throw any unchecked exception (runtime exception).
- Initialization of a static variable in a class doesn't throw any unchecked exception (runtime exception).
For example, let's resolve an error thrown in the first example to see point 1 in action:
Output:
Explanation: The resolution is straightforward. In Example1, the java.lang.ExceptionInInitializerError is thrown because of an ArithmeticException caused due to division by zero. So, we change the divisor to some number other than zero during the initialization of variable s.
Let's explore another example for resolution of error thrown in example 2 to see point 2 in action:
Output:
Explanation: In Example2, the string variable took a null value as default because we didn't initialize it. Next, we invoked the string's length method, which was not applicable for null values (causing NullPointerException). Hence, we have to initialize the variable s with an actual string variable to resolve java.lang.ExceptionInInitializerError that originated inside the static initializer block.
Conclusion
- The static variables are initialized at the time of declaration or in a static initializer block.
- The evaluation of static variables takes place during the class loading process. But runtime exceptions can occur. It is the situation when the JVM throws a java.lang.ExceptionInInitializerError.
- ExceptionInInitializerError is a child class of LinkageError. That's why the JVM doesn't load a class that throws this error.
- JVM wraps the runtime exception (thrown in static variable declaration or static initializer block) inside an instance of ExceptionInInitializerError so that a reference to original exception is maintained.
- Sometimes, the error can result in NoClassDefFoundError. It confuses programmers that the required class is not loaded due to some other reasons.
- Various unchecked exceptions like NullPointerException, ArithmeticException, IndexOutOfBoundsException, etc may lead to java.lang.ExceptionInInitializerError.
- Java doesn't allow unchecked exceptions (like FileNotFoundException) inside the static initializer blocks. One may perform error handling by employing a try-catch block and throwing an ExceptionInInitializerError, which wraps the original exception. It is a clean and recommended way that follows Java's design principles.
- Unexpected exceptions may occur in evaluation of static initializer blocks or initialization of static variables. These are the two causes.
- One can resolve the java.lang.ExceptionInInitializerError by ensuring that the initialization of static variables or evaluation of static initializer blocks doesn't throw any runtime exception.