Convert Java long to int
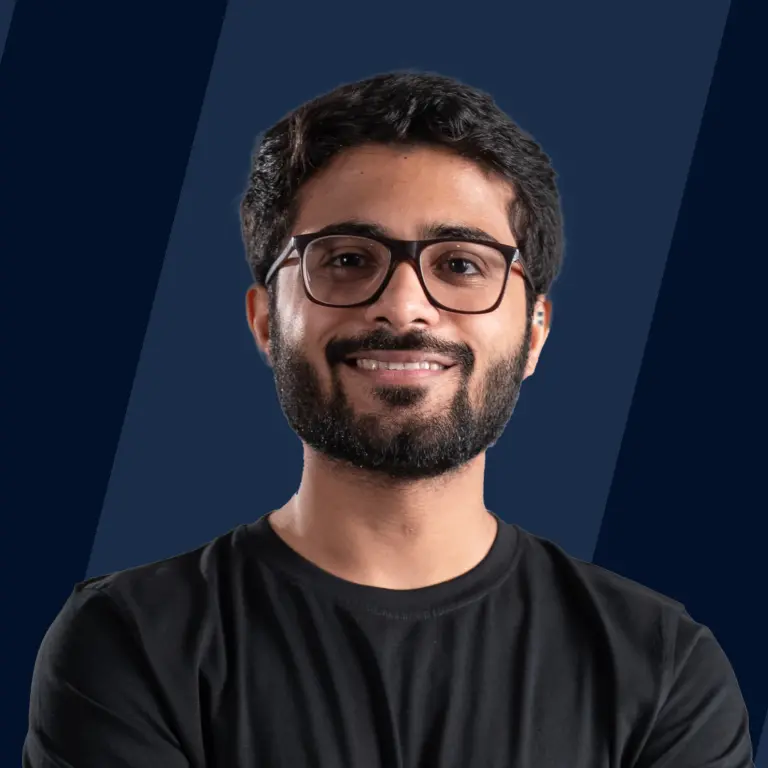
In this post, we'll outline the process of converting long to int in Java and provide some sample code. We describe how to convert Java long to int using type-casting as it is one of the most popular methods for changing data types, and we cover many additional approaches we may use for the same purpose utilizing the methods and functions offered by Java 8.
Here, we'll go through how to convert both a Long object and a Long primitive type into an int.
Introduction
Long and int are both considered primitive data types in Java, as you are presumably already aware. They are not class objects, thus. Primitive types like int and long are stored in Stack memory, whereas all objects and arrays are kept in heap space.
Since long and int are both integer types, they are somewhat compatible.
Let's explore different ways we can convert java long to int.
1. Converting Java Long to Int by Type-Casting
The Java application allots 64 bits for a long type, however, the long type's range is between -263 and 263 - 1. In addition, the int type takes up 32 bits and allows for the insertion of values between -231 and 231 - 1. As a result, any integer type can be incorporated into a long type. When working with primitives in Java, the translation from a smaller type to a larger type happens automatically. It is sometimes referred to as narrowing.
Here, we're giving a long variable an int. Everything went without a hitch; a smaller one fits quite smoothly in a bigger range, and we only lose memory space.
Now let's get back to the main task of this article — Java long to int conversion. Something bigger doesn't always fit inside something smaller, which is the difficulty. Therefore, in this case, Java cannot automatically convert "smaller" to "larger." Let's see what happens when we follow the preceding example oppositely.
Output:
As you can see, the Java compiler throws an error.
Now, you may wonder how we can convert long to int differently than when we convert from int to long.
For this operation, typecasting is used. In this case, it's known as narrowing type conversion.
You must explicitly state the type you want to cast your value to narrow a variable.
Syntax/Declaration:
The typecast operator () in Java is used to accomplish typecasting (datatype).
The data type that we wish to convert the source data type to is called target datatype.
Here is an example in Java where we will attempt to convert one "small" long number to an integer (which should fit) along with two "large" long numbers that are outside the range of an integer.
Now, storing a bigger value (long value) in a small container (int variable) can have its own consequences, as you can imagine. Let's say we hold ourselves responsible for that, just for the sake of this article.
The compiler carries out the conversion when it notices an explicit indication of a narrower type. Thus, we obtain the following output:
Output:
We certainly anticipated seeing 16000, but why is there a negative number in -2147483648? What does this 1410065408 imply exactly?
Now, remember the consequences of storing a bigger number (long value) in a small container (int variable), as we talked about earlier? This is one of those.
The truth is that these numbers were kept in a long variable, which takes up 64 bits, as we all recall. These numbers are being attempted to be stored in an int variable, which can only hold 32 bits. Typically, the first 32 bits of the long integer will be represented by zeros and ones in these 32 cells, and the remaining bits will just be ignored. Therefore, the bits that do not fit are simply eliminated if the original number cannot be fitted into 32 bits.
We only have the right number of 16000 because it uses less than 32 bits as a result.
Let's look at another example of typecasting in Java.
Java
Output:
As you can see, we are using the type casting operator to store a value of type long in the intnum variable, which was originally of type int.
You must've noticed we've used l at the end of long values in some examples like this.
Wonder why is that?
Assigning the letter l when declaring a variable of type long and specifying its value is preferable because doing so will be useful when working with integers outside the int range.
2. Converting Java Long to Int Using toIntExact() Method
The toIntExact() function is one of the various ways to convert java long to int.
Syntax:
Here, value (of type long) is a parameter to our toIntExact() method. It returns the input argument (of type long) as an int.
Now, what if the result overflows int? We get an ArithmeticException error in such cases.
Let's now see a Java program to see this in action.
java program
Output:
3. Converting Java Long to Int Using intValue() Method of the Long Wrapper Class
We can also use the Long Wrapper class in Java to convert Java longs to ints using the intValue() method.
Now, what is the long wrapper class again? Allow us to give you a brief description of it.
The wrapper class in Java provides a technique for converting a primitive into an object and an object into a primitive.
Autoboxing and unpacking features automatically turn primitives into objects and objects into primitives since J2SE 5.0. Autoboxing and unpacking are the automatic transformations of a primitive into an object.
A value of the primitive type long is enclosed in an object by the Long class. One field of the type of length can be found in an object of type Long.
This class also includes other constants and methods essential for working with longs and several methods for converting a long to a String and a String to a long.
Let's look at some long-class constructors while we're at it.
Long class Constructors
- Long(long value) - creates a brand-new Long object with the provided long argument as its representation.
- Long(String s) - creates a Long object with a fresh allocation representing the long value specified by the String parameter.
Let's wrap this up (pun intended 😁) with an example of Java
If you'd love to learn more about wrapper classes in Java, you're in luck! We already have an informative, fun article you can check out here.
Getting back to our topic, let's see how we can use intValue() method of Long Wrapper class in Java to convert java long to int
Syntax:
As you can see here, we have no parameters to this method. The return value, however, is numerical after it undergoes the whole process of being converted from an object initially.
Let's look at this example in Java
Output:
The intValue() method transforms a longnum object of type long into an int primitive type.
4. Converting Java Long to Int with Casting Values
Casting value is one of the most popular methods of type conversion in Java. Let's see an example in Java.
Output:
As we can see the returned value was of type int in the output.
5. Converting Java Long to Int Using BigDecimal
Operations on double numbers for arithmetic, scale management, rounding, comparison, format conversion, and hashing are provided by the BigDecimal class. With a little time complexity, it can handle very big and very small floating point numbers with high precision.
We can also carry out this conversion by using the BigDecimal class in Java. Let's have a look at this example.
Output:
Here, we've used the intValueExact() method for converting long to int.
int intValueExact(): transforms this BigDecimal into an int while looking for any lost data.
6. Converting Java long to int Using Guava
Many of Google's fundamental libraries are contained in Guava, an open-source, Java-based library used in many of Google's projects. It makes optimal coding practises easier to implement and reduces coding errors. It offers convenience functions for basic support, caching, concurrency, common annotations, string processing, input/output, and validation.
- Download Guava Archive
Download the latest version of Guava jar file from guava-18.0.jar. We have downloaded guava-18.0.jar and copied it into C:\>Guava folder.
OS | Archive name |
---|---|
Windows | guava-18.0.jar |
Linux | guava-18.0.jar |
Mac | guava-18.0.jar |
- Set Guava Environment
Set the Guava_HOME environment variable to the base directory location on your computer where the Guava jar is kept. On various Operating Systems, let's assume we've extracted guava-18.0.jar into the Guava folder as shown below.
OS | Output |
---|---|
Windows | Set the environment variable Guava_HOME to C:\Guava |
Linux | export Guava_HOME=/usr/local/Guava |
Mac | export Guava_HOME=/Library/Guava |
- Set CLASSPATH Variable
Set the Guava jar location as the CLASSPATH environment variable's starting point. The following assumes that you have placed guava-18.0.jar in the Guava folder on various Operating Systems.
OS | Output |
---|---|
Windows | Set the environment variable CLASSPATH to %CLASSPATH%;%Guava_HOME%\guava-18.0.jar;.; |
Linux | export CLASSPATH=export CLASSPATH=$CLASSPATH:$Guava_HOME/guava-18.0.jar:. |
Mac | export CLASSPATH=$CLASSPATH:$Guava_HOME/guava-18.0.jar:. |
Now let's see how we can do type conversion using Google Guava's Ints class:
Additionally, Google Guava's Ints class provides a saturatedCast method:
7. Converting Java Long to Int with Integer Upper and Under Bounds
Not to mention, it's important to always remember that an integer value has an upper bound and a lower bound. Integer.MAX VALUE and Integer.MIN VALUE offer these boundaries. Depending on the methodology and procedure, the outcomes change for values outside of the specified limitations.
We'll test the scenario in which an int value is unable to store the long value in the following line of code:
When using direct cast, lambda, or boxing values, a negative value is produced. In this situation, the return value is wrapped in a negative number since the long value exceeds Integer. ' MAX Result The outcome value is positive if the long value is less than Integer. ' MIN VALUE.
Nevertheless, three techniques discussed in this article might result in various sorts of exceptions:
A ArithmeticException is the outcome of the first and second. A IllegalArgumentException is raised in the latter case. Ints.checkedCast then determines whether the integer is within its range.
Last but not least, the saturatedCast method from Guava examines the integer upper and lower bounds first, and if the provided number is more or smaller than them, returns the limit value:
Java Programming Examples on Long to Int Conversion
Let's look at some examples on long to int coversion in Java
Example 1: Computing Area of Rectangle
In this example, we will compute a rectangle's area and convert it to an integer value.
Output:
Here using the intValue() method we've printed out the area of rectangle (which was computed by the operations of two long value variables) of the type int.
Example 2: Find the Value of Series of Numbers
In this example, after converting the integers to equivalent int values, we determine the sum of the numbers.
Output:
Similarly here, we've converted the end result into an int using the intValue() method.
Example 3: Find the Volume of Cube
In this example, we're determining the volume of a cube that was previously saved as a long number and changing it to an int value.
Output:
Here, we convert the long value to int using the type-casting operator and print out the respective result.
Example 4: Compute the Simple Interest
In this illustration, the principal is preserved as a long variable and used to calculate the simple interest. At the end of the time period, we also calculate the overall amount of money collected.
Output:
Here the volume of the cube is computed using operators of type long and the end result is printed of type int .
Conclusion
- The typecast operator in Java is used to accomplish typecasting (datatype).
- int intValueExact() method transforms this BigDecimal into an int while looking for any lost data.
- The intValue() method transforms a longnum object of type long into an int primitive type.
- Wrapper class provides a technique for converting a primitive into an object and an object into a primitive.
- When typing casting, a programmer uses the casting operator to change one data type into another during the programme's creation. It is also known as a narrowing conversion.