Java Map Entry
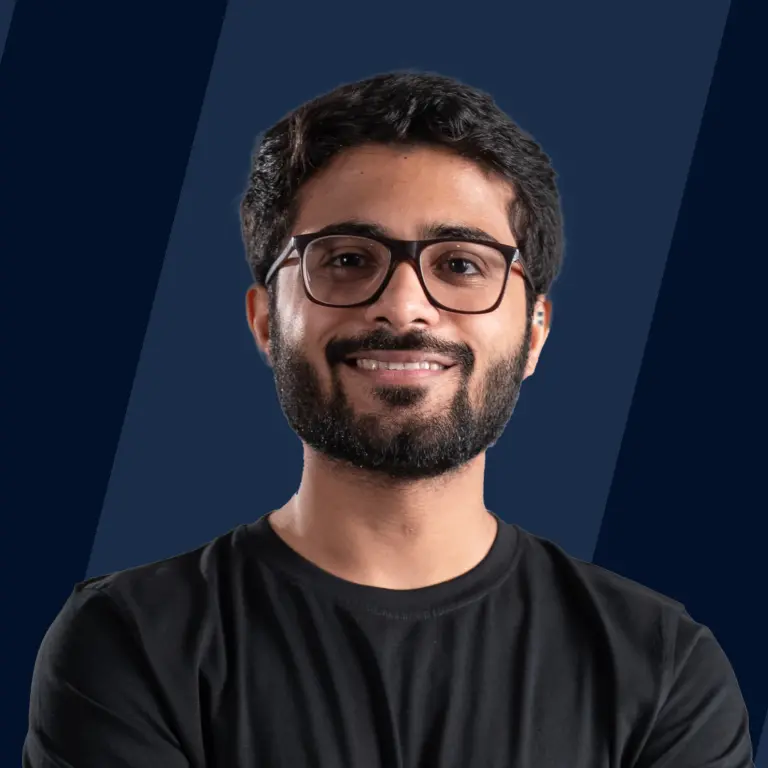
Overview
It is common knowledge that if you want to store your data in a linear fashion or by index, you can do that with the help of arrays in Java, but what if you want to store your data in key-value pairs? This is where the Java Map interface comes to our rescue.
A map in Java is a data structure that stores your data in key-value pairs. Here, each key in a map is unique and serves as an index for easy identification and retrieval of data. On the other hand, the entity associated with a particular key is called a "value," and the map stores these values with their referencing keys. A few things that are important to note here are that while each key in a map has to be unique, values can be repeated, which means multiple keys can have the same values. This type of mapping is often also referred to as "one-to-one" mapping. Also, maps in Java only take in objects and not primitives, so both keys and values that are added to a map need to be objects. Once we've stored a key-value pair inside a map, we can get the value associated with a particular key by using the .get() method, which takes in a key and returns the associated value.
Now, each key value tuple that is added to a map is referred to as an "entry" (i.e., a key, along with its associated value). The Map. The entry interface in Java provides a way to access these entries in the map, and once we can access them, we can easily modify them according to our needs.
So in this article, we are going to learn everything about the Java Map.entry interface, all the classes that implement the interface, all the methods that are declared in the interface, and how to use it in combination with another very useful method called .entrySet(). Along the way, we will also look at several examples that will help us better understand the concept. So without wasting any more time, let us start learning.
Introduction to Java Interface Map.Entry
Before we move ahead, let us consider a scenario. Let us assume we have to store the maximum marks scored by a student in five subjects. you can make an array of size 5 and can then store the 5 maximum marks in that array as shown in the below java code
But something seems missing. How would anyone know which index in the array corresponds to any specific subject? A person would have to have prior knowledge of how indexes of the array related to the subjects. Wouldn't it be great if we could store the name of the subject and its corresponding marks together inside a single data structure? Well, we can make a Pair class that stores the name of the subject along with corresponding marks and then make an array of that Pair class. But that is complicating things too much. There must be a better way, and there is. This is where the Java map interface comes into play. It allows you to store a key and its corresponding value together inside a map. So if we use a map in the above example instead of an array, we can store the subject name and its corresponding marks as a single entry. Let's look at a Java program that uses a map instead of an array to store the maximum marks of a student in 5 subjects.
As you can see from the above code, it is much more logical to store the data in a map if your data has to be stored in key-value pairs. Each key along with its corresponding value is known as an entry in a map, and to access these entries and modify them, we use the Java map Entry interface.
All Known Implementing Classes
Sr. No. | Class Name |
---|---|
1 | AbstractMap.SimpleEntry |
2 | AbstractMap.SimpleImmutableEntry |
Enclosing Interface
The Map interface is the enclosing interface of the Java map.entry interface.
Declaration
declaration of the Java map.entry interface looks something like this.
As we have already discussed, a map stores the data in key-value pairs, and each key-value pair is known as an entry on Java. but we can't iterate in a map as we do in an array. There is now indexing for an entry in a map, so there is no direct way to access all the entries except the map.entrySet() method, which returns a collection-like view of a map whose elements are of type Map.Entry. The only way to get a reference to the entries in a map is by iterating over the collection view of the map that is returned by the map.entrySet().
One important thing to note here is that each Map.entry object that is part of the set that is returned by the map.entrySet() method exits only for the duration of the iteration which in simpler terms means that once the iterator returns a Map.entry object and if the map that we are working on is modified, the behavior of the previously returned entry is completely undefined.
Before things start to get a little confusing, let us look at a simple java program to understand the working of the entrySet() method and the Map.entry objects.
Output:
As you can see in the above code, we are creating a map in which the key is of the integer type and the associating value is of the string type. Then we are inserting the entries inside the map with the help of the Java map.put() method. Now comes the interesting part, we are using the map.entrySet() method that will return us the set of Map.Entry objects will represent entries inside our map. Moving ahead, we are creating an iterator over this set so that we can iterate through all the entries, and finally, we are printing the key and value in each Map.Entry object. We are extracting the key and its corresponding value with the help of the .getKey() and .getvalue() methods, respectively, of the java map entry Interface.
Now, that was a lot of new information that we just unraveled, but don't worry, we will look at each of the methods that are declared by the java map entry interface and also look at some examples that will help us to better understand this concept.
Method Summary of Java Interface Map.Entry
we saw a few of the methods that are declared in the java map entry interface. in this section, we will look at all the methods that are declared on the Map.Entry interface.
All of the declared methods
Sr. No. | Modifier and Type | Method name | Explanation |
---|---|---|---|
1 | static <K extends Comparable<? super K>,VComparator<Map.Entry<K, V>> | comparingByKey() | This method returns a comparator that is used to compare Map.Entry objects in natural order on key. |
2 | static <K,V> Comparator<Map.Entry<K, V>> | comparingByKey(Comparator<? super K> cmp) | This method returns a comparator that is used to compare Map.Entry objects by the key using the given Comparator. |
3 | static <K,V extends Comparable<? super V>> Comparator<Map.Entry<K, V>> | comparingByValue() | This method returns a comparator that is used to compare Map.Entry objects in the natural order of the values. |
4 | static <K,V> Comparator<Map.Entry<K, V>> | comparingByValue(Comparator<? super V> cmp) | This method returns a comparator that is used to compare Map.Entry objects by value using the given Comparator. |
5 | boolean | equals(Object o) | This method is used to Compare the concerning object with this entry for equality. |
6 | K | getKey() | This method returns the key which is corresponding to this entry. |
7 | V | getValue() | This method returns the value which is concerning to this entry. |
8 | int | hashCode() | This method gives us the hash code value for this map entry. |
9 | V | setValue(V value) | This method replaces the value concerning this entry with the specified value (optional operation). |
Time Complexity:
All the Methods of the Java Map.The entry interface works in O(1) time.
Static Methods
Here you can see the method of the java Map.Entry interfaces are static. Static methods are those methods that belong to the class itself and not any instance of the class. You don't have to create an object of the class to access static methods of that class, you can directly access them by using the class name. to learn more about static methods, click here.
Sr. No. | Modifier and Type | Method name | Explanation |
---|---|---|---|
1 | static <K extends Comparable<? super K>,VComparator<Map.Entry<K, V>> | comparingByKey() | This method returns a comparator that is used to compare Map.Entry objects in natural order on key. |
2 | static <K,V> Comparator<Map.Entry<K, V>> | comparingByKey(Comparator<? super K> cmp) | This method returns a comparator that is used to compare Map.Entry objects by the key using the given Comparator. |
3 | static <K,V extends Comparable<? super V>> Comparator<Map.Entry<K, V>> | comparingByValue() | This method returns a comparator that is used to compare Map.Entry objects in the natural order of the values. |
4 | static <K,V> Comparator<Map.Entry<K, V>> | comparingByValue(Comparator<? super V> cmp) | This method returns a comparator that is used to compare Map.Entry objects by value using the given Comparator. |
Instance Methods
Instance methods are the methods that require an object of the class to be accessed. Click here to learn more about instance methods.
Sr. No. | Modifier and Type | Method name | Explanation |
---|---|---|---|
1 | boolean | equals(Object o) | This method is used to Compare the concerning object with this entry for equality. |
2 | K | getKey() | This method returns the key which is corresponding to this entry. |
3 | V | getValue() | This method returns the value which is concerning to this entry. |
4 | int | hashCode() | This method gives us the hash code value for this map entry. |
5 | V | setValue(V value) | This method replaces the value concerning this entry with the specified value (optional operation). |
Abstract Methods
Abstract methods are methods with signatures but without any body of content. The body for abstract methods is provided in the implementing subclasses. Click here to learn more about Abstract methods.
Sr. No. | Modifier and Type | Method name | Explanation |
---|---|---|---|
1 | boolean | equals(Object o) | This method is used to Compare the concerning object with this entry for equality. |
2 | K | getKey() | This method returns the key which is corresponding to this entry. |
3 | V | getValue() | This method returns the value which is concerning to this entry. |
4 | int | hashCode() | This method gives us the hash code value for this map entry. |
5 | V | setValue(V value) | This method replaces the value concerning this entry with the specified value (optional operation). |
Default Methods
Default methods were introduced in Java 8. Before Java 8, only Abstract methods were allowed inside an interface which made it difficult to add new methods to the interface as the new methods will then be required to be declared in the implementing subclasses. Default methods allow us to create methods with the body in an interface. Click here to learn more about default methods in java.
Sr. No. | Modifier and Type | Method name | Explanation |
---|---|---|---|
1 | static <K extends Comparable<? super K>,VComparator<Map.Entry<K, V>> | comparingByKey() | This method returns a comparator that is used to compare Map.Entry objects in natural order on key. |
2 | static <K,V> Comparator<Map.Entry<K, V>> | comparingByKey(Comparator<? super K> cmp) | This method returns a comparator that is used to compare Map.Entry objects by the key using the given Comparator. |
3 | static <K,V extends Comparable<? super V>> Comparator<Map.Entry<K, V>> | comparingByValue() | This method returns a comparator that is used to compare Map.Entry objects in the natural order of the values. |
4 | static <K,V> Comparator<Map.Entry<K, V>> | comparingByValue(Comparator<? super V> cmp) | This method returns a comparator that is used to compare Map.Entry objects by value using the given Comparator. |
Method Detail of Java Interface Map.Entry
Now we will look at some of the above-mentioned methods in detail.
1. getKey()
This method returns the Key value of the Map.entry object and it throws the IllegalStateException. f the entry has been removed from the backing map, implementations may, but are not required to, throw this exception.
2. getValue()
This method returns the value corresponding to an entry. but if the entry has been removed from the map by using the remove operation of the iterator, the result of this method is undefined. Just like the getKey method, this method also throws the IllegalStateException and if the entry has been removed from the backing map, implementations may, but are not required to, throw this exception.
3. setValue()
This method replaces the specified value with the value corresponding to this entry (optional operation). (Writes through to the map.) If the mapping has already been removed from the map (via the iterator's remove operation), the behavior of this call is undefined.
Parameters:
Value - New value to be stored in this entry
Returns: An old value corresponding to the entry Throws:
UnsupportedOperationException - If the backing map does not support the put operation.
ClassCastException - If the class of the specified value prevents it from being stored in the backing map.
NullPointerException - If the backing map does not allow null values and the specified value is null
IllegalArgumentException - If some property of this value prevents it from being stored in the backing map.
IllegalStateException - If the entry has been removed from the backing map.
4.equals()
This technique Checks for equality between the specified object and this entry. If the given object is also a map entry and the two entries represent the same mapping, this function returns true. More formally, two entries e1 and e2 represent the same mapping if (e1.getKey()==null ? e2.getKey()==null : e1.getKey().equals(e2.getKey())) && (e1.getValue()==null ? e2.getValue()==null : e1.getValue().equals(e2.getValue()))
This ensures that the equals method works correctly across different Map implementations of the Map.Entry interface.
the declaration of this method overrides the .equals() method in the Object class.
5. hashCode()
This method returns the value of a map entry's hash code. The hash code for a given entry is defined as follows.
(e.getKey()==null ? 0 : e.getKey().hashCode()) ^ (e.getValue()==null ? 0 : e.getValue().hashCode())
This ensures that e1.equals(e2) implies e1.hashCode()==e2.hashCode() for any two Entries e1 and e2, as required by the Object.hashCode general contract.
This method also overrides the .hashcode() method defined in the object class.
6. comparingByKey
Declared as : static <K extends Comparable<? super K>,V> Comparator<Map.Entry<K,V>> comparingByKey()
This method Returns a comparator that compares Map.Entry in natural order on key. When comparing an entry with a null key, the returned comparator is serializable and throws a NullPointerException.
7. comparingByValue
Declared as: static <K, V extends Comparable<? super V>> Comparator<Map.Entry<K,V>> comparingByValue()
This method Returns a comparator that compares Map.Entry in natural order on value. When comparing entries with null values, the returned comparator is serializable and throws a NullPointerException.
8. comparingByKey
Declared as: static <K,V> Comparator<Map.Entry<K,V>> comparingByKey(Comparator<? super K> cmp)
This method Returns a comparator that compares Map.Entry by key using the given Comparator. If the specified comparator is also serializable, the returned comparator is serializable.
9. comparingByValue
Declared as: static <K,V> Comparator<Map.Entry<K,V>> comparingByValue(Comparator<? super V> cmp)
This method Returns a comparator that compares Map.Entry by value using the given Comparator. If the specified comparator is serializable, the returned comparator is also serializable.
Java Interface Map.Entry Examples
Ok, so that was a lot of new information that we got till now. Let us see some of the above-mentioned methods and interfaces in action by going through some simple yet interesting examples that use the java Map.Entry interface. Let us start.
Example 1
let us start by looking at a very simple java Program that uses the entryset() method and the getKey() and getvalue() methods of the java Map.Entry objects.
Output:
In this example, we are creating a map of String as a Key type and double as a value type. Then we are entering the values in the map and create a Set of entries of the type Map.Entry with the help of the .entrySet() method. We are then using an iterator i to loop over all the entries in the map and print the keys and values of each entry with the help of the getKey() and getvalue() methods of the Map.Entry object.
Example 2
Ok, so we saw a simple java program that uses the java Map.Entry objects and the java .entrySet() method. But let us move ahead and look at some important Map.Entry methods in action. In this example, we are going to see the Map.Entry.comparingByKey() method of the Map.Entry interface.
Output:
So in this example, we are creating a map of Integer as the key type and String as a Value type. then we are entering the key value pairs or entries into the map and create a set of map entries of the type Map.Entry by using the .entrySet() method.
Then we are sorting the map by the sorted() method which takes in a comparator basis on which the entries in the map will be sorted. here we are using the Map.Entry.comaringByKey() method which returns a comparator that compares the Map.Entry objects by the natural order of the key. Then we are finally printing the keys and values in each entry using the forEach() method.
As you can see in the output, Map entries are sorted by the natural order of the key.
Example 3
In the last example, we saw how we can sort the entries in our map by using the .comparingByKey() method of the java Map.Entry interface. This method returns a comparator that compares the key values in the entries by their natural ordering. But what if we want to sort the entries in the reverse ordering of the key? Well, let us look at a java program that shows us how to do so.
Output:
This java program is very similar to the code that we saw in the last example, the only difference being that while sorting the entries using the Map.Entry.comparingByKey() method, we are also passing a parameter to the method called Comparator.reverseOrder(). This method reverses the natural ordering of the comparison.
Example 4
Ok, so in the last 2 examples, we have seen how we can sort the entries using the .comparingByKey() method. but we can also sort the entries based on the values corresponding to those keys. Java Map.The entry interface has a special method for that called .comapringByValue(). Let us look at a java program to see how that method works.
Output:
Just like in previous examples, here also we are creating a map of Integer as the key type and String as a value type and entering key value pairs into the map. Then we are sorting the map by using the .sorted() method that we discussed in the previous example. The only difference is that here at the place of the comparator, we are passing the .comparingbyValue() method of the Map.Entry interface which returns a comparator that compares the Map entries by natural ordering of the "Value" elements. Finally, we are printing the key value pairs by using the .forEach() method.
As you can see in the output, the entries of the map are now sorted in the natural ordering.
Example 5
Just like we saw with the .comparingByKey() method that returns a comparator that compares the entries on basis of the natural ordering of the key, we can reverse the ordering by using the Comparator.reverseOrder() method. We can use the same method with the .comparingByValue() method. Let us look at a java program that does that.
Output:
In this java program, we are first creating a map with Integer as the key type and String as the Value type. Then we are entering values into the map by using the java map.put() method. Then we are creating a set of Map.Entry objects by using the map.enrtySet() method. We are sorting the Entries on the natural ordering of the Values by using the Map.Entry.comparingByValue() method and then reversing the order by using the Comparater.reverseOrder() method.
Conclusion
- Java maps are used to store data in key-value pairs
- In a map, each key is unique whereas multiple keys can have the same corresponding value
- Each key along with its corresponding value together is known as an entry in java.
- Map.entry along with the .entrySet() method is used to get access to these entries and iterate over them which we cannot do with traditional looping methods.
- Several methods are declared by the java Map.entry interface not only helps us to get both the key and the value for a specific entry but also allows us to perform several comparative operations over them.
Thank You for Reading!