Java Map.put()
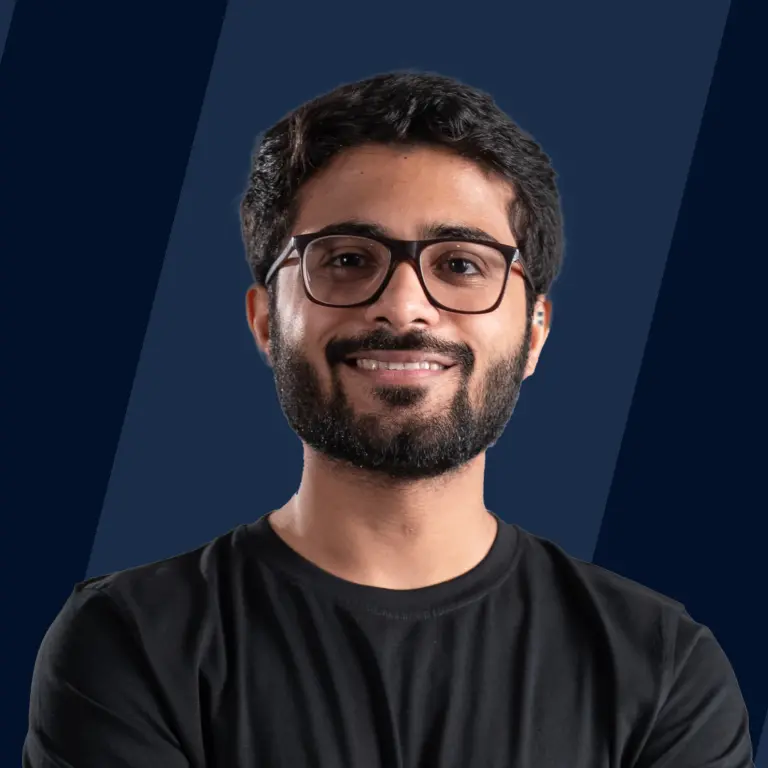
A hash map in Java is a data structure that stores data in key-value pairs. We can insert values with a unique key and get those values back using those unique keys.
To insert data in a HashMap, you can use the Java HashMap put() method. It takes in two parameters: the unique key and the value to be inserted, and it adds them to your Map.
What is a HashMap?
The Hashmap class is part of the Java Collections framework and it provides the functionality of storing key/value pairs. In HashMap, keys are used as unique identifiers to associate each value on the map.
What is Java Map put()?
HashMap put() is a Java method that inserts a key-value pair to the hashmap. It adds the specified value against the specified key inside the hashmap. This method adds the value in O(1), which is constant time.
Time Complexity: O(1)
Example:
Output:
Explanation:
As you can see, we insert integer numbers corresponding to some characters using the Java HashMap put() method and then print the map.
Syntax for Java HashMap put()
The syntax for the java HashMap put() method this
Here, map is an object of the HashMap class in Java, and put() is a method for inserting key-value pairs into the hashmap.
Parameters for Java HashMap Put Method
Java map put method takes in 2 parameters
- Key k -> identifiers to which values are going to be mapped
- Value v -> actual value that is mapped to a particular key
Return Value of Java HashMap put()
We can have 2 cases regarding the return value of the java map put() method.
- Key already Present: If the key we are trying to add is already present inside the map, then that put() method replaces the previously associated value with that key with the new value inside the map and returns the previous value.
- Key NOT Present: If the key we are trying to add is not already present inside the map, then the put() method adds the new key-value pair inside the map and returns null.
If the key is already present inside the map and the value associated with it is null, then put() method will also return null.
Examples
Now, let's look at some examples to understand how the Java HashMap put() method works.
Example 1: Adding values using java put() method
Output:
Example 2: Inserting elements with duplicate keys
Output:
Explanation:
- In this example, we are again creating a hashmap class object named map and adding the student name and roll number using the HashMap put() method.
- As you can see, when an existing key is updated, the put() method returns the previously mapped value.
In this line, we are trying to add a new roll number for the key "prem", which already exists in the hashmap. By doing this we are replacing the old value with the new value.
Conclusion
- Java HashMap put() is a method that is used to add key/value pairs inside a hashmap.
- It takes in 2 parameters
- Key for assigning values
- Value assigned to that key
- Java HashMap put() method returns the previously stored value for the key if it was already present in the hashmap. Otherwise, it adds the key/value and returns null.