Java Math cos()
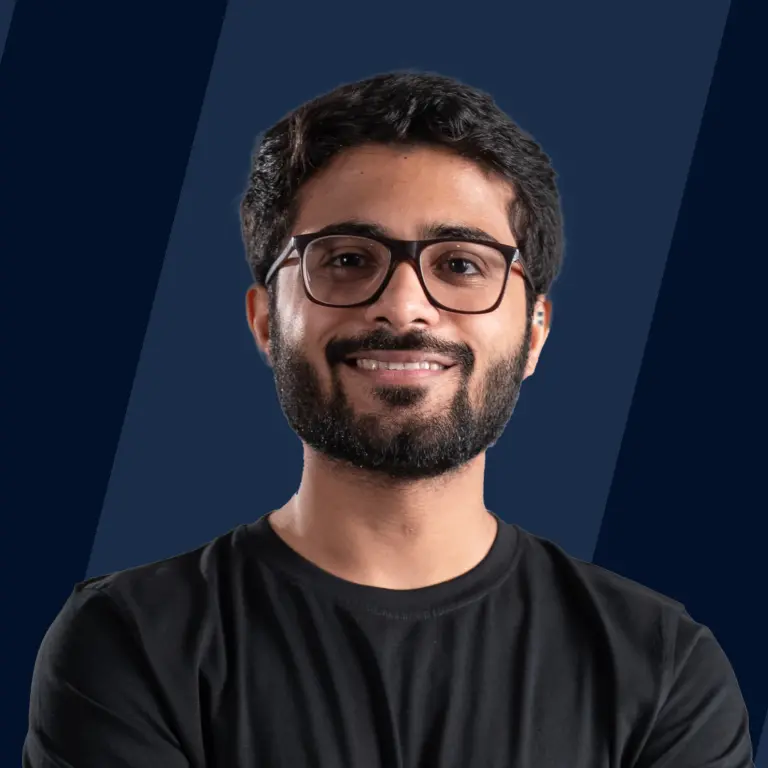
The cos() method in Java calculates the cosine of an angle. It accepts a single argument, the angle in radians, and returns a double value representing the cosine of the angle.
- If the input is NaN (Not-a-Number):
- The method returns NaN.
- If the input is positive infinity or negative infinity:
- The method returns NaN.
- If the input is positive zero (+0.0):
- The method returns 1.0.
- If the input is negative zero (-0.0):
- The method returns 1.0.
- For all other finite input values:
- The method returns the cosine value between -1 and 1, depending on the input angle.
Syntax of Math cos() in Java
The syntax of the cos() method is:
The method signature looks like this:
Here, cos() is a static method. So, we are accessing the method using the class name Math.
Learn more about static methods in Java here.
Parameters of Math cos() in Java
The cos() method accepts a single parameter angle, measured in radians, whose trigonometric cosine will be returned.
Return Value of Math cos() in Java
Return type : double
Math cos() method returns the trigonometric cosine of the specified angle It also returns NaN if the specified angle is NaN or infinity.
Exceptions of Math cos() in Java
The Math.cos() method in Java does not throw any exceptions.
Example
Let's see an implementation of Math.cos() method in Java
Output
Explanation:
The angles 30 and 45 degrees are first converted to radians using the Math.toRadians() method. The Math.cos() method is then used to calculate the cosine values of the angles, which are subsequently printed to the console as 0.8660254037844387 and 0.7071067811865476, respectively.
What is Math cos() in Java?
The Math.cos() method returns a double value between -1 and 1, representing the angle's cosine.
Because cos() is a static method of Math so, you always use it as Math.cos(), rather than as a method of a Math object you created.
Let's see an implementation of java.lang.Math.cos() in Java
Output
More Examples
Below is a Java code that demonstrates using the cos() method of Math class.
Output:
The Java example source code up top demonstrates the use of the Math class's cos() method. We only request user input and parse it using the Scanner class.
Since the console result was obtained using the nextLine() method and the return data type was String, we converted it to a double using Double.parseDouble().
Because the cos() method only accepts double method arguments, we must first convert it to double.
We also used Math.toRadians() to convert the data to radians, which is the necessary method argument, after converting it into double.
Example 1: Using Math cos() with Double.MAX_VALUE
Output:
where, Double.MAX_VALUE is a constant holding the largest positive finite value of type double
The value is stored in a'; it is then converted to radians using the Math. radians ()function and stored inb; we then use the cos()method onb` to obtain its cosine value.
Example 2: Using Math cos() with Double.POSITIVE_INFINITY
Let’s see the implemenation of cos() with Double.POSITIVE_INFINITY in Java
Output
where Double.POSITIVE_INFINITY is a constant holding the positive infinity of type double.
We then use the cos() method to obtain its result. Since If the argument is an infinity, then Math.cos() returns NaN as a result.
Example 3: Using Math cos() with Double.NEGATIVE_INFINITY
Let’s see the implementation of cos() with Double.NEGATIVE_INFINITY in Java.
Output
This is the constant holding the negative infinity of type double. We've used the cos() method to obtain its result. Since If the argument is an infinity, then Math.cos() returns NaN.
Example 4: Using Math cos() with Double.NaN
Let’s see the cos() implementation with Double.NaN in Java.
Output
Since the argument is NaN, then Math.cos() returns NaN.
Conclusion
- The java.lang.Math.cos() function returns the trigonometric cosine of an angle. The computed result should be within 1 unit in the last place of the actual result. The returned value will fall within the range of [-1, 1].
- As per the official documentation, the cos() method of Math expects the angle to be specified in radians. It calculates and returns the cosine of the given angle.
- In Java, the Double class provides special constants such as Double.NaN, Double.POSITIVE_INFINITY, and Double.NEGATIVE_INFINITY. When the Math.cos() method encounters an argument that is NaN or infinity, the result of the cos() function will be NaN.