Java Math sin()
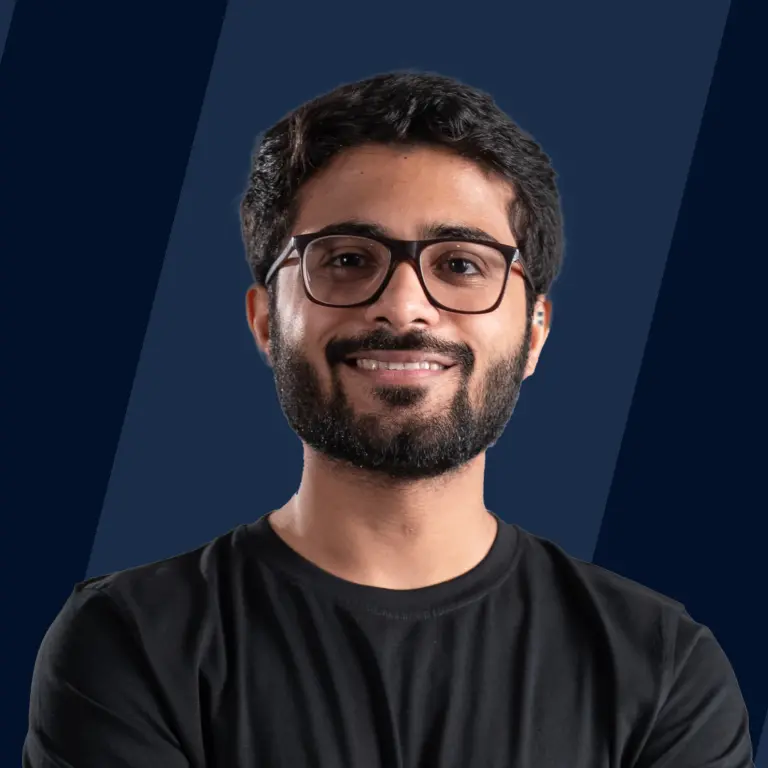
The built-in java.lang.Math.sin() function returns the sine value of the argument supplied. The argument is expected to be in radians. If the argument is NaN (Not-a-Number) or infinity, the function will return NaN as the outcome. If the argument is zero, the function will return 0 with the same sign as the argument. The value returned by the function will always be between -1 and 1, representing the range of the sine function.
Syntax of Math sin() in Java
The Java Math sin() returns the trigonometric sine of the specified angle.
The syntax of the sin() method is:
Here, sin() is a static method. Hence, we are accessing the method using the class name, Math
Learn more about static methods in Java here
Parameters of Math sin() in Java
The sin() method takes a single parameter, an angle, measured in radians, whose trigonometric sine will be returned.
Return Value of Math sin() in Java
The sin() method in Java returns the trigonometric sine of the specified angle. It returns NaN if the specified angle is NaN or infinity.
If the argument is zero, however, then the result of the sin() method is also zero with the same sign as the argument.
Example
Let's now see an example in Java, in which we will simply showcase the working of the Math.sin() method of java.lang package method
Output
Because the sin() method only accepts double method arguments, we must first convert it to double. We also used Math.toRadians() to convert the data to radians, which is the necessary method argument, after converting it into double.
What is Math sin() in Java?
The trigonometric sine of an angle is returned by the Java method sin() (angle should be in radians). If the argument is NaN or an infinity, the result will also be NaN; if the argument is zero, the result will be zero with the same sign as the argument.
In the example below, the sin() method is used to determine an angle's trigonometric sine.
The output of the above code will be:
More Examples
Now, Let's see how we can implement sin function on an ArrayList in Java
Here, we've declared a double-type array list and determined each element's sine values.
Output
Example 1: Using Math sin() with Double.MAX_VALUE
Output:
Where Double.MAX_VALUE is a constant holding the largest positive finite value of type double
The value is stored in a, value is then converted in radians using the Math.toRadians() function and is then stored in b, we then use the sin() method upon b to obtain their sine value.
Example 2: Using Math sin() with Double.POSITIVE_INFINITY
Output:
Where Double.POSITIVE_INFINITY is a constant holding the positive infinity of type double, we can use the sin() method to obtain its result. If the argument is an infinity, then Math.sin() returns NaN as a result.
Example 3: Using Math sin() with Double.NEGATIVE_INFINITY
Output:
The code demonstrates the use of the Math.sin() method to calculate the sine of a negative infinity value and prints the result.
Example 4: Using Math sin() with Double.NaN
In the following example, we pass the Double.NaN as an argument to the sin() method. As per the function's definition, sin() should return the NaN value.
Output
Since the argument is NaN (not a number), then Math.sin() returns NaN.
Conclusion
The Math.sin() method in Java returns the sine value of an input angle in radians.
- If the input angle is a positive finite number, the method returns the sine value between -1 and 1.
- If the input angle is positive infinity (Double.POSITIVE_INFINITY) or negative infinity (Double.NEGATIVE_INFINITY), the method returns NaN (Not-a-Number).
- If the input angle is NaN (Not-a-Number) or exceeds the range of a double value, the method also returns NaN.
- The method returns the corresponding zero value for the input angle of positive zero (+0.0) or negative zero (-0.0).
- The method returns exact values for some specific angles such as 0, π/6, π/4, π/3, π/2, etc., while for other angles, it provides an approximation based on the mathematical trigonometric function.
- The returned values are of the double data type.