Java Program to Merge Two Lists
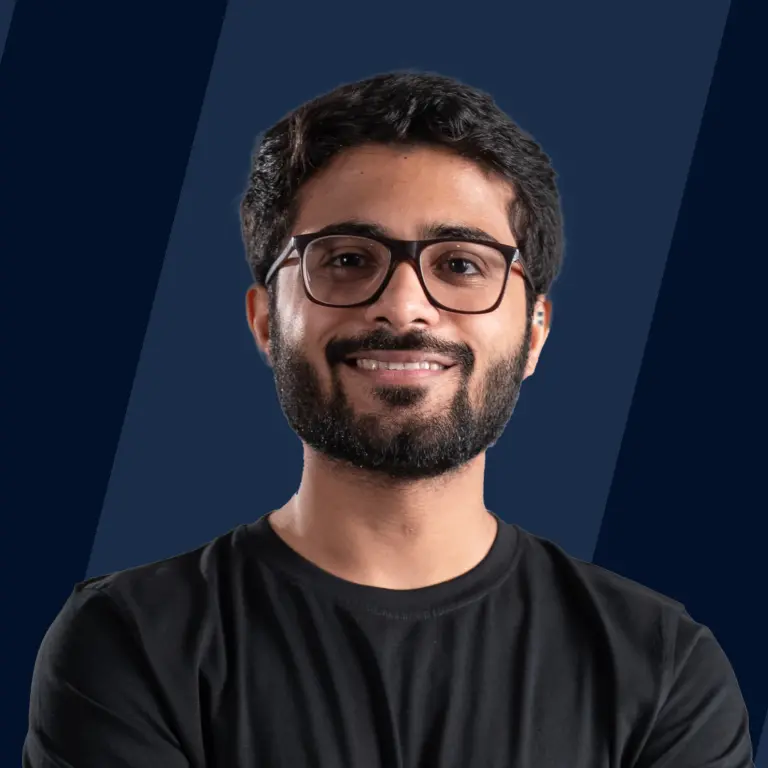
Overview
We use lists frequently throughout Java and in many different contexts. Merging two lists is an important idea, and there are different techniques to do this process.
Introduction
You should be knowledgeable about the following Java programming concepts to understand this article. Let's discuss them briefly
1. Java ArrayList
The ArrayList class is a resizable array, which can be found in the `java.util package.
Now you must be wondering how it is any different from the built-in array in Java.
In Java, a built-in array and an ArrayList differ in that an array's size cannot be changed; instead, a new array must be created if you want to add or remove entries from an existing one. While an ArrayList can have any number of elements added or removed at any time
Syntax
Learn more about ArrayList in Java here
2. Java List
Java's list feature makes it possible to keep an organized collection. It includes index-based techniques for adding, updating, deleting, and searching components. Duplicate elements are also possible. The list can also contain null elements.
The Collection interface is inherited by the List interface, which may be found in the `java.util package. It is the interface's ListIterator factory.
Syntax:
While ArrayList is the implementation class of List, List is an interface
The List interface is implemented by the ArrayList and LinkedList classes. Let's look at a few samples for the List:
To put it briefly, you can make a List of any kind. The type is specified by the classes ArrayList<T> and LinkedList<T> T here stands for type.
Learn more about List in Java here
3. Linked List
Similar to the ArrayList, the LinkedList class is a collection that can hold numerous items of the same type.
Because both classes implement the List interface, the LinkedList class and the ArrayList class have all of the same methods. This indicates that you can edit, add, delete, and clear the list in the same manner.
Although they can both be used in the same way, the ArrayList and LinkedList classes are constructed very differently.
Containers are how the LinkedList organizes its elements. Each container contains a connection to the next container in the list, and the list itself has a link to the first container.
A new container is created, the element is added to the list, and the new container is linked to one of the existing containers.
A LinkedList is used to manipulate data, whereas an ArrayList is used to store and access data
The LinkedList offers numerous ways to do specific operations more quickly:
Method | Description |
---|---|
addFirst() | Adds an item to the beginning of the list. |
addLast() | Add an item to the end of the list |
removeFirst() | Remove an item from the beginning of the list. |
removeLast() | Remove an item from the end of the list |
getFirst() | Get the item at the beginning of the list |
getLast() | Get the item at the end of the list |
Learn more about Linked list in Java here
Some prerequisites for running the codes discussed in this article in Java are:
- Download and Install the following Apache Commons Collections library here
- Go to your project's properties right away.
- Decide on the Java Build Path.
- Select Libraries from the Java Build Path menu.
- Choose Classpath under libraries.
- Next, open the external JARs section and add the JAR executable file that you received from the provided URL.
Merge Two Lists Using addAll()
This function adds all the members of the specified list in the same order as the original list by passing the name of the list as an argument.
Syntax
- Create a new empty list (concatenated_list)
- Concatenate the two lists you've been given, list1 and list2, using the addAll() method.
Following the aforementioned processes, both of the lists are now present in our empty list
Let's now see how we can implement it in Java for ArrayList
Output
Two lists named list1 (for prime) and list2 (for even) in the above example are created using
Here, we've added every element from prime and even to the newly created list called merged_list using Java's ArrayList addAll() method
Let's now see how we can implement the same in Java for Linked List
Output
Here, we've firstly created two lists L1 and L2
Then, these two lists are merged using the addAll() method
Let's look at the time and space complexity of addAll()
Time Complexity: , where n is the size of the collection to be added. Space Complexity: , where n is the size of the collection to be added.
Merge Two Lists Using The Stream Class
This method accepts two streams as arguments and joins them together to form a new stream. The second list is then appended to the first list.
Learn more about the stream in Java here
Syntax
Let's see how we can merge two lists in Java using the stream class
Output
After creating two lists prime and even as,
We then convert both prime and even list into stream
The two lists were combined in the mentioned example using the Stream class
Here we've used three functions of stream:
- stream() - this function the given list into a stream.
- concat() - this function takes two streams as parameters and returns the concatenated one.
- collect(Collectors.toList()) - this function converts the stream into a list
The merged stream is then converted into a list using collect(Collectors.toList()) function
Merge Two Lists Using Union()
The command returns a new concatenated list after receiving two list arguments. The second list is then appended to the first list.
Syntax
Let's see how we can merge two lists in Java using union()
Output
list1 and list2 are created first using
These two lists were concatenated using the ListUtils.union() method in a newly created list called concatenated_list
Conclusion
- addAll() method to merge two lists in Java to add all the members of the specified list in the same order as the original list by passing the name of the list as an argument.
- stream class in java can be used for merging two lists, it accepts two streams as arguments and joins them together to form a new stream. The second list is then appended to the first list.
- We can merge two lists in Java using the union() method. It returns a new concatenated list after receiving two list arguments. The second list is then appended to the first list.
- The merged stream is then converted into a list using collect(Collectors.toList()) function.
- We've used three functions in stream to merge two lists in this article: stream(), Concat(), collect(Collectors.toList()).