Java Output Formatting
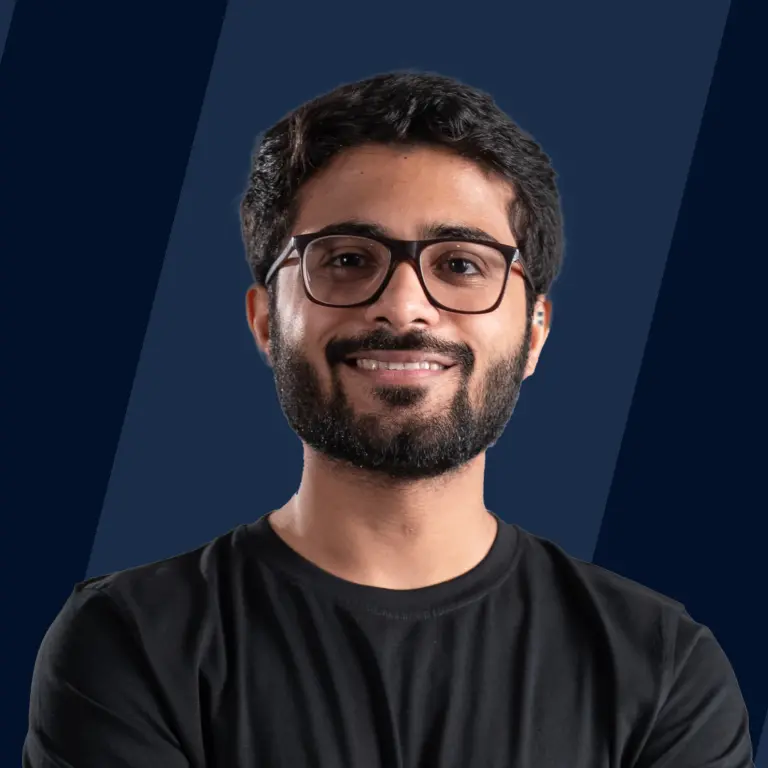
Learn about Java Output Programming, where code comes alive! Have you ever wondered how Java programmes interact with you? It is via their product. Java Output Programming focuses on showing outcomes, whether they be simple "Hello, World!" or complicated data structures. Imagine your code as a storyteller, telling its plot via the console. This introduction will take you on a journey through the interesting world of commands and meaningful results. Join the quest to decipher Java's language and let your code say volumes. Prepare to discover the magic beneath your terminal as we dig into the succinct world of Java Output Programming!
Formatting Using Java printf()
In Java programming, accuracy and readability are critical. The printf() function is a flexible formatter that may raise your output to a professional level. In this lesson, we'll look at the complexities of formatting using Java's printf() function, with a focus on numeric formatting, decimal numbers, and Boolean values.
For Number Formatting
When working with numerical data, it is critical to explain it clearly and concisely. The printf() function lets you choose the width and accuracy of your output. For example, imagine you wish to show the numeric variable count with a minimum width of 5 characters. You may accomplish this using the following snippet:
In this example, %5d indicates that the integer should be formatted with a minimum width of 5 characters. Adjust the number accordingly to meet your specific requirements.
Formatting Decimal Numbers
Precision becomes even more important when working with decimal numbers. Java's printf() provides a dependable solution for formatting floating-point values. Let's say you have a double variable price representing a monetary value, and you want to display it with two decimal places:
Here, %.2f signifies that the floating-point number should be formatted with two digits after the decimal point. The %f is a placeholder for a floating-point number, and you can adjust the precision as needed.
For those instances where you want to include a thousands separator for better readability, you can employ the %, flag:
The %,.2f format ensures that the large number is displayed with two decimal places and includes a comma as a thousands separator.
For Boolean Formatting
Java's printf() is not limited to numeric values; it can also handle Boolean values with ease. Suppose you have a Boolean variable isJavaFun indicating whether Java is fun or not:
In this case, the %b format specifier is used to represent a Boolean value. It automatically converts the Boolean into a human-readable "true" or "false".
For String Formatting
Let's start learning with the most common use case – string formatting. With printf(), you can inject values into strings with style. Take this snippet, for example:
Here, %s and %d act as placeholders for the string and integer values, respectively. The result? A neatly formatted string that dynamically incorporates the variables.
For Char Formatting
Moving on with the char formatting, it's a breeze with printf(). Need to display a character with specific formatting? Consider the following example:
The %c specifier is your ticket to formatted char output. Simple, yet effective.
For Date and Time Formatting
Java's printf() isn't just for basic types – it's also a wizard at handling dates and times. Let's say you want to display the current date and time:
Here, %tF and %tT provide placeholders for the date and time components, respectively. The result is a nicely formatted display of the current date and time.
Format a Table
Now, let's start by formatting data in a table format. This is very useful when presenting information methodically. Check out this example.
In this example, we utilized format specifiers such as %-15s to specify a left-justified string with a width of 15 characters. The result is a table with cleanly aligned columns.
Java's printf() function is a flexible tool that allows you to format output with ease. Whether you're working with strings, characters, dates, times, or even creating tables, printf() is an intuitive solution. As you begin your Java programming adventure, keep this formatting superpower in mind; it might be the hidden weapon you need to make your output stand out.
Formatting Using the DecimalFormat Class
In programming, precision and presentation are synonymous. When dealing with numerical data in Java, the DecimalFormat class emerges as a valuable tool for formatting numbers with style. In this section, we'll look at how to use DecimalFormat to accomplish precision formatting in Java, guaranteeing that your numeric outputs are both correct and visually pleasing.
The DecimalFormat class in the java.text package provides a versatile technique for formatting decimal integers. Its flexibility stems from its ability to customise the presentation of numbers, such as adjusting the number of decimal places, selecting grouping separators, and handling currency symbols.
Syntax:
Let's start with learning the basic syntax of creating a DecimalFormat object:
Here, pattern is a string that defines the format you want for your numbers. It can include various placeholders, such as #, 0, and ,.
Setting Decimal Places:
One typical need is to limit the number of decimal places shown. The # sign in the pattern denotes an optional digit, whereas the 0 represents an obligatory digit. For example:
This pattern ensures that only up to two decimal places are displayed, rounding as necessary.
Handling Grouping Separators:
To enhance readability, you might want to include grouping separators like commas for thousands. The , symbol in the pattern accomplishes this:
This pattern adds commas for thousands and ensures up to two decimal places are displayed.
Dealing with Currency Symbols:
When dealing with monetary values, having currency symbols is essential. The pattern uses the ¤ symbol to represent the currency sign:
This pattern adds the currency symbol, commas for thousands, and two decimal places for precision.
Applying Formatting:
Now that you've set up your DecimalFormat object, applying it to format numbers is a breeze:
This snippet demonstrates how to format the myNumber variable according to the specified DecimalFormat pattern.
Understanding the DecimalFormat class in Java allows you to portray numerical data with precision and flair. Whether you're working with financial data, scientific computations, or any other numerical output, knowing how to use DecimalFormat ensures that your numbers make sense and look beautiful. So, plunge into the realm of DecimalFormat and improve the visual attractiveness of your Java apps!
Formatting Dates and Parsing Using SimpleDateFormat Class
Managing dates is a typical problem in Java programming. Whether you're showing dates to users or processing user input, a good understanding of date formatting and parsing is required. The SimpleDateFormat class is an important tool in Java for performing these operations. In this post, we'll look at SimpleDateFormat and how it may help you interact with dates in your Java applications.
Understanding SimpleDateFormat
The SimpleDateFormat class in Java allows you to format and parse dates based on a pattern. It defines the date format using a combination of letters and symbols. For example, yyyy-MM-dd indicates the year, month, and day in a common format.
Date Formatting with SimpleDateFormat
Let's start by learning how to format dates with SimpleDateFormat. To do this, create an instance of SimpleDateFormat and provide the required date pattern. Here is a simple example:
In this example, we create a SimpleDateFormat instance with the pattern yyyy-MM-dd HH:mm:ss and use it to format the current date and time. The resulting formatted date is then printed to the console.
Date Parsing with SimpleDateFormat
On the other side, parsing involves the conversion of a date string into a Date object. Here's how you can achieve this using SimpleDateFormat:
In this example, we define a SimpleDateFormat instance with the pattern yyyy-MM-dd and use it to parse a date string. The parsed Date object is then shown on the terminal. It is important to handle ParseException, which may occur if the input string does not fit the required pattern.
Understanding the Common Format Symbols in SimpleDateFormat patterns is key. For example:
Mastering date formatting and parsing in Java using the SimpleDateFormat class allows you to perform date-related activities more effectively. Whether you're working with user inputs, database records, or showing dates in your application's user interface, understanding SimpleDateFormat is essential. With this understanding, you may easily traverse the complex world of dates in your Java programs.
Java String Format Specifiers
String Format: Specifiers are placeholders within a string that determine the format of different data types. They function as blueprints, instructing Java to display or handle certain values in a string. Common syntax for a specifier is %[flags][width][.precision] specifier.
Let's break down the components:
- Percent Sign (%):
This signals the beginning of a format specifier. - Flags:
Optional indicators that modify the output format. For example, the - flag left-aligns the result. - Width:
Specifies the minimum width of the output. It ensures uniformity in the displayed data. - Precision:
Applicable for floating-point numbers. It indicates the number of digits to display after the decimal point. - Specifier:
Denotes the data type to be formatted. For instance, %d is used for integers, %f for floating-point numbers, and %s for strings.
Let us now look at some of the most commonly used specifiers.
%d - Integer
Output:
%f - Floating-point
Output:
%s - String:
Output:
Let us now look at some additional tips.
Combining Specifiers
You can use multiple specifiers in a single format of the string to display multiple values.
Output:
Order Matters:
Ensure that the specifiers in the format string are in the same order as the associated variables.
In Java programming, learning String Format Specifiers gives you a powerful tool for customizing data presentation. Whether you're creating user-friendly output or dealing with difficult data formatting duties, understanding these specifiers allows you to improve the precision and readability of your Java programs. Now, armed with this information, you may bring a new level of clarity to your Java string formatting!
Conclusion
- Precision is important when formatting Java output. Whether you're working with floating-point numbers or dates, preserving accuracy ensures that the output is both true and intelligible. Pay attention to the amount of decimal places or the date format to transmit information correctly.
- Well-formatted output improves the readability of your program. The use of indentation, line breaks, and uniform spacing makes the output more accessible and comprehensible. A clear and organized display of information is critical for both developers and end users.
- Java includes a variety of formatting options, allowing developers to tailor output to specific requirements. Use formatting symbols, width specifiers, and flags to customize the way data is displayed. This amount of customization guarantees that your output presents information.
- Java output formatting follows locale-specific norms. This is especially important when working with data that differs due to regional or cultural variances, such as date and time formats or numerical representations. Being locale-aware improves your application's worldwide adaptability and usability.
- Properly handling exceptions and mistakes in Java output formatting is important. Prepare for problems that may develop during formatting operations, such as mismatched data types or erroneous format specifiers. Robust error handling guarantees that your program handles unexpected events graciously, resulting in a more consistent user experience.