Java Class PrintWriter
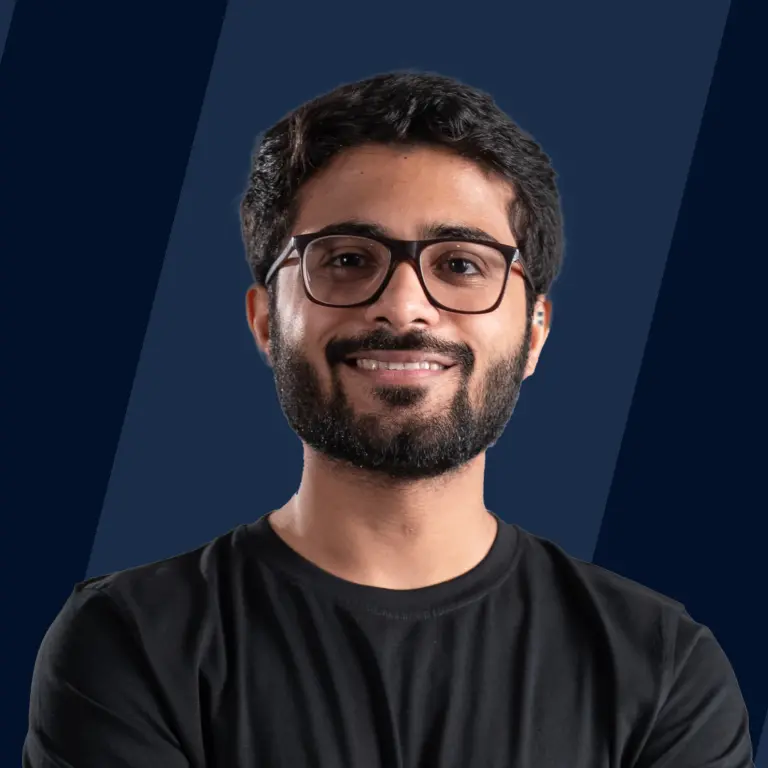
Overview
The Writer class is implemented by the Java PrintWriter class. The formatted representation of objects is printed using it to the text output stream. To mix text and numbers while creating reports or other documents, the Java PrintWriter can be helpful. Except for the methods for writing raw bytes, the PrintWriter class has all the same methods as the PrintStream class. The PrintWriter is a subclass of Writer and is used to write text.
Introduction to Java Class PrintWriter
The formatted representation of objects can be printed to the text output stream using the Java PrintWriter class. The java.io.PrintWriter package is defined by the built-in class known as PrintWriter. A superclass of the PrintWriter is the Writer class. The PrintWriter class makes it possible to write formatted objects to the base Writer class, such as writing int, double, long, and other primitive objects or data as text rather than as bytes values. Except for the methods that write raw bytes, the PrintWriter class implements all print methods as the PrintStream. It is helpful to create reports that combine text and numbers because the PrintWriter class was designed to compose the text.
Why use PrintWriter if other OutputStreams are Available?
The most common practice to print data on the console is by using the System.out.print method. However, it is easier to customize the format as per the specified Locale (regional standards) while publishing global applications using the PrintWriter object. We can look into using Locale according to your system later in this post. To learn about formats in java click here.
Another benefit of using PrintWriter is that you may instruct it to automatically flush its data after each println() operation. (However, closing the stream when you are done with it is still a wise precaution.)
Working of PrintWriter
PrintWriter transforms the raw data (int, float, char, etc.) into the text format, unlike other writers. The formatted data is then written to the writer.
Additionally, no input or output exceptions are thrown by the PrintWriter class. To identify any errors in it, we must utilize the checkError() method.
Note: Auto flushing is a feature of the PrintWriter class. This means that if one of the println() or printf() methods is called, it forces the writer to send all data to the destination.
Creating a PrintWriter
Using another Writer
Code Explanation
-
We have created a print writer that will write data to the file that the FileWriter represents.
-
The optional parameter autoFlush defines whether or not to use automatic flushing.
Using other Output Streams
Code Explanation
-
We have created a print writer that will write data to the file that the FileOutputStream represents.
-
The optional parameter autoFlush defines whether or not to use automatic flushing.
Using Filename
Code Explanation
- We have created a PrintWriter Object that will write data to the file that we have given.
- The optional parameter autoFlush defines whether or not to use automatic flushing.
Note: In each of the above scenarios, the PrintWriter uses a standard character encoding to send data to the file. The character encoding (UTF8 or UTF16) can also be specified.
All Implemented Interfaces
- Closeable
- Flushable
- Appendable
- AutoCloseable
Field Summary of Java Class PrintWriter
Modifier and Type | Field and Description |
---|---|
protected Writer | Field |
Fields Inherited from Class java.io.Writer
Modifier and Type | Field and Description |
---|---|
protected Object | The thing that synchronizes this stream's operations. |
Constructor Summary of Java Class PrintWriter
Constructor | Description |
---|---|
PrintWriter(File file) | creates a new PrintWriter using the provided file but without automatic line flushing. |
PrintWriter(File file, String csn) | creates a new PrintWriter with the supplied file and charset but without automatic line flushing. |
PrintWriter(OutputStream out) | creates a new PrintWriter from an existing OutputStream without performing automatic line flushing. |
PrintWriter(OutputStream out, boolean autoFlush) | converts an existing OutputStream into a new PrintWriter. |
PrintWriter(String fileName) | creates a new PrintWriter with the specified file name but without automatic line flushing. |
PrintWriter(String fileName, String csn) | Creates a new PrintWriter, without automatic line flushing, with the specified file name and charset. |
PrintWriter(Writer out) | new PrintWriter is created without automatic line flushing. |
PrintWriter(Writer out, boolean autoFlush) | Creates new PrintWriter. |
Method Summary of Java Class PrintWriter
Constructor | Description |
---|---|
PrintWriter append(char c) | The specified character is added to this writer. |
PrintWriter append(CharSequence csq) | adds the desired character string to this author |
PrintWriter append(CharSequence csq, int start, int end) | adds a subsequence of the desired character sequence to this writer. |
boolean checkError() | checks the stream's error condition and flushes it if it is not closed. |
protected void clearError() | removes this stream's error state. |
void close() | Closes the stream and releases any related system resources. |
void flush() | Flushes stream. |
PrintWriter format(Locale l, String format, Object... args) | uses the provided format string and parameters to write a formatted string for this writer. |
PrintWriter format(String format, Object... args) | Using the format string and parameters supplied, write a formatted text to this writer. |
void print(boolean b) | print boolean value. |
void print(char c) | print character. |
void print(char[] s) | an array of characters are printed. |
void print(double d) | prints a floating-point number with double precision. |
void print(float f) | a floating-point number is printed.. |
void print(int i) | print an integer. |
void print(long l) | print a long int. |
void print(Object obj) | print an object |
void print(String s) | print the string. |
PrintWriter printf(Locale l, String format, Object... args) | An easy way to use the format string and parameters supplied to write a formatted string to this writer. |
PrintWriter printf(String format, Object... args) | An easy way to write a formatted string using the given format string and parameters to this writer. |
void println() | writes the line separator string to end the current line. |
void println(boolean x) | a boolean value is printed before the line is ended. |
void println(char x) | terminates the line after printing a character. |
void println(char[] x) | prints a range of characters before cutting the line. |
void println(double x) | ends the line after printing a double-precision floating-point number. |
void println(float x) | a floating-point number is printed before the line is ended. |
void println(int x) | terminates the line after printing an integer. |
void println(long x) | terminates the line after printing a long integer. |
void println(Object x) | terminates the line after printing an Object. |
void println(String x) | terminates the line after printing a String. |
protected void setError() | shows that an error has happened. |
void write(char[] buf) | a array of characters are written. |
void write(char[] buf, int off, int len) | Writes a portion of a array of characters. |
void write(int c) | writes one character at a time. |
void write(String s) | a string is written. |
void write(String s, int off, int len) | a piece of a string is written. |
Java Class PrintWriter Examples
Example 1
In this example, we are going to find how to write to console and text file using the printwriter class in java.
Output
test.txt
Code Explanation:
In the first part, we have printed data to the console using printwriter. We have passed the object of System.out to the constructor for writing on the console. write() method of the printwriter class is used to write to the console. After printing, we used the flush() and clear() methods to flush and close the stream, respectively.
In the Second part of code, we have written data into the text file. We have created a printwriter that will write data to the test.txt file by passing the file name to the constructor. clear() methods to flush and close the stream, respectively.
Example 2
In this example we are going to check how to print different data type to console
Output
Code Explanation:
In the above code, we have used different PrintWriter methods to print different data types to the console.
Conclusion
- We can write formatted data to an underlying Writer using the Java PrintWriter class java.io.PrintWriter.
- The java.io.PrintWriter package is defined by the built-in class known as PrintWriter.
- A superclass of the PrintWriter is the Writer class.
- It is easier to customize the format as per the specified Locale (regional standards) while publishing global applications using the PrintWriter object.
- PrintWriter class provides different methods, constructors, and fields.