Java Scanner next() Method
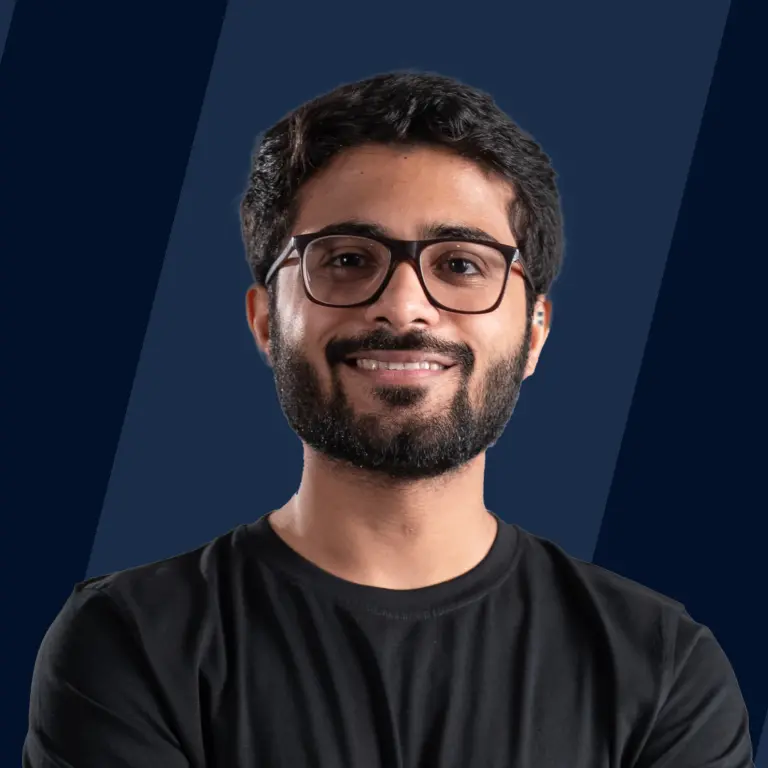
Overview
The Scanner class in java.util package consists of the next() method which takes the input from the user. This input is stored in the form of a string. The next() method of the Scanner class is used to find and return the next token.
Syntax of Java Scanner next()
The Java Scanner next() can be applied in 3 different ways-
Java Scanner next() method
This method is used to get the next complete token from the scanner. This method does not contain any parameters.
Syntax:
Java Scanner next(String pattern) method
This method is used to return the next token if it matches the pattern constructed by the specific string.
Syntax:
Java Scanner next(Pattern pattern) method
This method is used to return the next token if it matches the pattern.
Syntax:
Parameters of Java Scanner next()
Parameters that can be taken as an input-
- String pattern- The data type is String and the parameter is a pattern. This provides a string specifying a pattern for the scan/token.
- Pattern pattern- The data type is Pattern and the parameter is a pattern. This provides a pattern to scan the specific string that satisfies the pattern.
Return Value of Java Scanner next()
The Java Scanner next() method returns a String in the form of the next token.
Exceptions of Java Scanner next()
There are two exceptions associated with Java Scanner next() method-
- NoSuchElementException- This exception is thrown when there is no token found.
- IllegalStateException- This exception is thrown when the Scanner is closed first before the invocation is completed.
Example
Let us understand in short how we can take input from the user using the Scanner next().
- First import the java.util package as it contains the Scanner class which has various methods.
- Then in the main function, we create an object of the Scanner class and then read the string. The user will enter the string which will be read in the form of tokens.
Output-
Explanation:
Here, we have created an object of the Scanner class. Then we have asked the user to enter his/her name and that value is read and stored using Java Scanner next method. The value is then displayed.
What is Java Scanner next()?
The Scanner class in java.util package consists of the next() method which takes the input from the user. This input is stored in the form of a string. The next() method of the Scanner class is used to find and return the next token. It only takes in the first token entered by the user.
If the user enters a string along with the spaces, the scanner next() method takes in the first token till the first space, and the latter part is not considered. The scanner considers the part till it encounters a space.
Example-
Here only the first word(i.e., Program) is stored.
For taking the entire line as the input, we use the Scanner nextLine() method.
More Examples
Example 1
Let us verify the fact written above that the next() method takes in the first word only.
Code-
Output-
Explanation:
- As you can see, the first word was only considered by the Scanner next() method.
- In the program above, same steps are followed to create an object of the Scanner class. Then the user is asked to enter his/her name and that value is read and stored using Java Scanner next() method.
- Here the user is entering a name along with spaces, but it only reads the first word. To read the entire line once, nextLine() method need to be used.
Conclusion
- The Scanner class in java.util package consists of the next() method which takes the input from the user. This input is stored in the form of a string.
- The next() method of the Scanner class is used to find and return the next token.
- The next() method of Scanner class is overloaded with three signatures:
- next()
- next(String pattern)
- next(Pattern pattern)
- The return type is a string.
- It has 2 exceptions associated with it- NoSuchElementException and IllegalStateException.