Thread.sleep() in Java
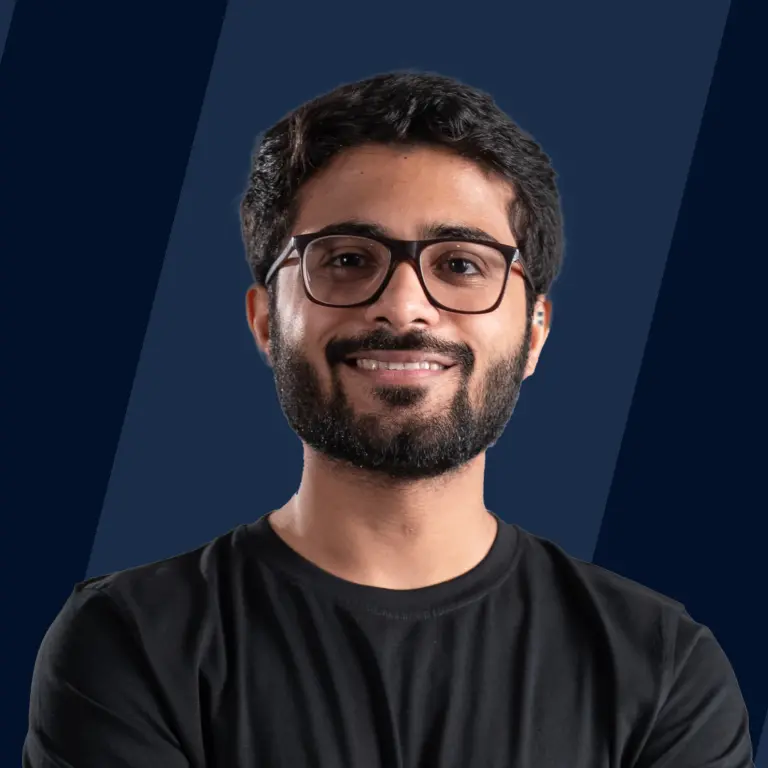
Overview
The Thread class is present in the java.lang package of Java and represents a thread of execution in a program. It contains the sleep() method, which is overloaded twice, with the first variant taking one argument and the second taking two. These methods allow us to pause the execution of the current thread for a specific duration of time. Once the specified time has elapsed, the thread resumes its execution from where it left off.
Syntax of Thread.sleep() in Java
We overload the sleep() function in Java. We call the corresponding function based on the number of parameters during the function call.
Parameters of Thread.sleep() in Java
The sleep method takes two arguments based on the syntax of the function (as discussed above) :
millis : It is the millisecond's duration of time for which the thread has to sleep.
nanos : It is the nanosecond's duration of time for which the thread has to sleep. Its value lies between to .
Return Value of Thread.sleep() in Java
Return Type : void
Thread.sleep() in Java does not return any value. Its return type is void.
Exceptions of Thread.sleep() in Java (If applicable)
The Thread.sleep() method always stops the current thread execution. When the current thread in sleep gets interrupted by another thread, it throws an InterruptedException. When the specified sleep time during the function call is negative, it throws an IllegalArguementException.
Example
Let us look at a simple program where we will use the Thread.sleep() in Java to pause the main thread execution for 2 seconds.
When you run the above code, it will sleep for 2 seconds and then print the sleep time in milliseconds to the console. The output will look something like this:
The output shows that the Thread.sleep() method causes the current thread to sleep for the specified number of milliseconds (in this case, 2000 milliseconds or 2 seconds). The System.currentTimeMillis() method is used to calculate the sleep time by subtracting the start time from the end time. The output confirms that the Thread.sleep() method successfully paused the execution of the current thread for the specified amount of time.
What is Thread.sleep() in Java?
The Thread.sleep() method is used to temporarily pause the execution of the current thread. It does so by putting the thread in a wait state for a specific duration of time, with the help of a thread scheduler. When the given sleep time is completed, the thread state is changed back to runnable, where it waits for the CPU to resume its execution. The actual time a thread sleeps depends on the system timer and the thread scheduler, which is a part of the operating system. In a quiet system, the sleep time is usually close to the specified time. However, in a busy system, the sleep time may vary more than the specified time. It's important to note that the current thread does not lose monitor and locks during thread sleep.
It is an efficient way of making processor time available for other threads in the application running on the system computer. It enables the computer to process multiple things at the same time.
More Examples
Using Thread.Sleep() Method For Main Thread
In the above example, we have written a Java program to stop the main thread execution using the Thread.sleep() method. We have used a for loop to print the number from 1 to 5 and simultaneously put the thread to sleep for 1 sec (1000 ms) each time the loop runs. If the program throws an exception, it runs the statement inside catch.
Output
Using Thread.Sleep() Method for Custom Thread
In the above example, we have written a Java program to stop the custom thread execution using the Thread.sleep() method. We have written a Scaler subclass that inherits the attributes and method from the Thread superclass. We have used a for loop to print the number from 1 to 5 and simultaneously put the thread to sleep for 1 sec (1000 ms) each time the loop runs. If the program throws an exception, it runs the statement inside catch.
Output
IllegalArguementException When Sleep Time is Negative
In the above example, we have written a Java program to show the working of an IllegalArgumentException using the Thread.sleep() method. We have used a for loop to print the number from 1 to 5. It simultaneously puts the thread to sleep for -100ms each time the loop runs. Since the value of the time argument in the sleep method cannot be negative, the program gives an IllegalArgumentException. If the program throws an IllegalArgumentException, it runs the statement inside catch.
Output
Conclusion
- The sleep() function is a method of the Thread class in the Java.lang package of Java.
- The Thread.sleep() function stops the current thread execution until a specified time.
- The time for which the thread stops gets passed as a parameter to the sleep function.
- The value of the parameter is in milliseconds and nanoseconds.
- The return type of sleep function is void.
- When a negative value of sleep time gets passed as the argument, it throws an IllegalArguementException.
- It throws an InterruptedException when another thread interrupts the current thread in sleep.