Java Stream map()
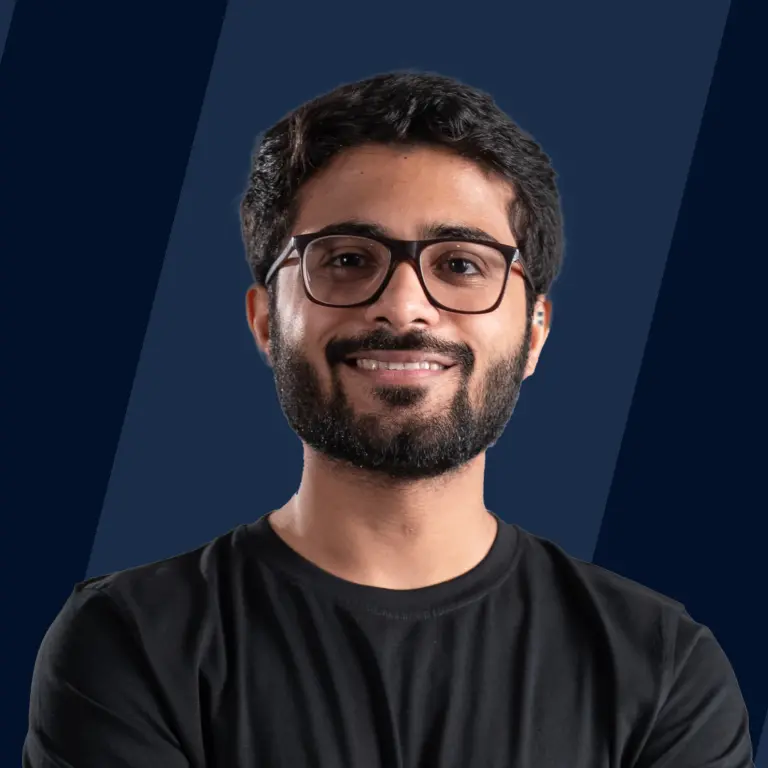
Overview
The map() method in Java Stream is a functional operation that transforms each element in a stream by applying a specified function to it. The map() method returns a new stream consisting of the transformed elements.
The map() method can be used to perform a wide range of transformations on a stream of objects. For example, it can be used to:
- Convert a stream of objects of one type to a stream of objects of another type, or
- Extract a specific property or field from each object in a stream.
Syntax of Java Stream map()
The syntax of the Java Stream map() method is as follows -
where,
R - It denotes the element type of the new Stream.
? super T - It represents that the original Stream should have the values that are a superclass of type T.
? extends R - It allows the method taken by map to be declared as returning a subtype of R
mapper - It is the stateless and non-interfering method that gets applied to each element of the Stream and produces a new Stream of values.
Parameters of Java Stream map()
The map() method accepts a parameter of type method<? super T,? extends R> which is a mapper Function that takes the values of type T and returns a value of type R. The T and R types refer to generic types.
An example of a mapper method can be Integer.toString(int a) that takes a value of type integer and converts and returns a value of type String in Java.
Therefore, you can pass any method, maybe shortcut code using the lambda expressions or a proper method. The key point is that the method’s argument type will be T and the resulting values will be of type R.
Return Value of Java Stream map()
The return type of the Java Stream map() method is <R> Stream<R>. It returns a stream of type R that has the same type as the mapper method you passed to the map() method. The Java Stream map() method basically is used to take an input Stream<X> and return an output Stream<Y> by applying a certain mapper Function to it.
Exceptions of Java Stream map()
The exceptions can occur in any piece of Java code and we need to handle these exceptions for maintaining the smooth processing of our program. Any type of exception can occur in the Java Stream map() method or any lambda methods as well therefore, they must be handled in advance.
Let us discuss one of the exceptions and how to handle it using the try-catch block in Java-
Output:
Explanation:
- The above program throws an ArithmeticException because the Java stream map() method contains a method as a parameter in which the stream element gets divided by zero.
- To handle such unexpected situations, we use the try-catch block in Java.
We basically extract the method that is passed to the map() method as a parameter and then apply the try block on it to avoid any exceptions. Let us have a look at it.
Output:
Explanation:
Now, the error that caused the program to stop will not occur because we have handled it using the try catch block.
Example
Let us now see an example of the Java Stream map() to increase all the values of the Stream by 2. Below is the code example for the same.
Output:
Explanation:
- In the above program, we have firstly created a list of array elements which are then passed to the add() method.
- Inside the add() method, the list is converted to a stream of elements, and the map() method is applied to it.
- The map() method takes the stream values one by one and performs the specific method on the element to increase its value by 2. After which the collector gathers the resultant elements again in a list which is returned to the caller method.
What is Java Stream map()?
Java Stream map() method is an intermediate operation that takes up the input stream elements one by one and returns an output stream of elements by applying a specific mapper function to each of the input stream elements.
The Java 8 Stream's map() method simply takes a stream of type X and returns another stream of type Y by applying the mapper function on the input stream elements and producing new stream elements of another type.
The mapper method used as a parameter in the map() method is a stateless method as it does not store the values of the previously processed elements. The mapped stream is closed after all of its elements are processed and placed in the resultant stream.
More Examples
Let us now discuss some more examples of the Java stream's map() method.
Example 1
In the first code example, we will be multiplying each input stream element by the number 5 and then print the resultant output stream elements.
Output:
Example 2
Now let us another example of the Java stream's map() method to convert all the uppercase letters to lowercase letters. Below is the code example for the same.
Output:
Explanation:
- In this example, we have taken all the stream elements one by one and converted them to the lower case using the mapper function of the map() method.
- Further, the collector collects the output stream elements in a new list.
Example 3
Now, let us consider one more example of the Java stream's map() method to extract the first 3 characters of a string in Java.
Output:
Explanation:
- In this example, we have used the substring() method in the map's mapper function to extract the first three characters from each of the strings in the list.
- After which the collector gathers them in the resultant output list.
Conclusion
- The Java Stream map() method is used to return a new stream of objects.
- The map() method takes a mapper function as an arguments and applies this mapper function to each element of the input stream and produces a resultant output stream.
- The Java Stream map() method is an intermediate operation.
- The mapper method in the map() method is a stateless method as it does not store the values of the already processed stream elements.
- The mapped stream gets closed after all its elements are placed in a new output stream.