Java Stream.reduce()
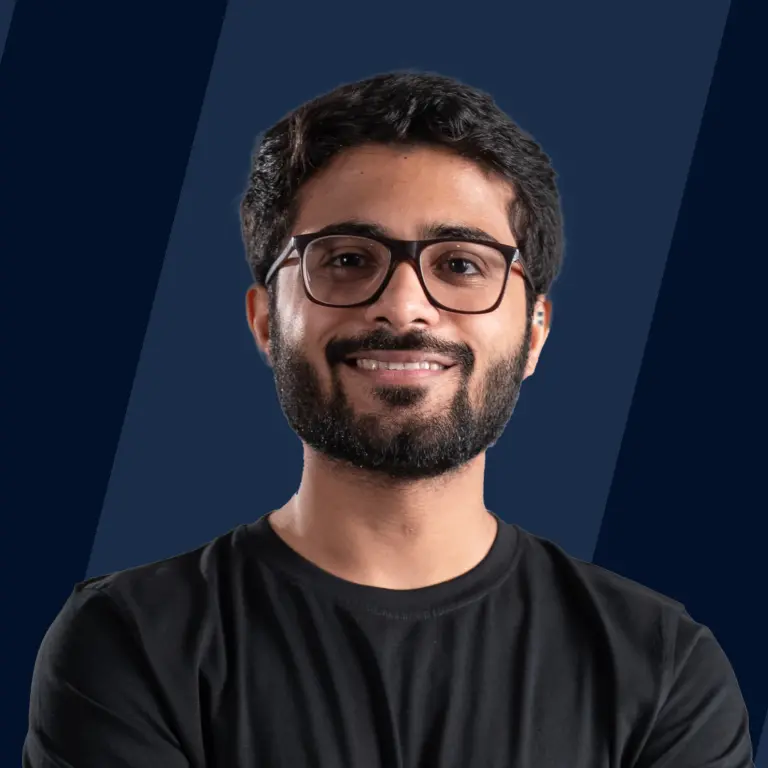
Overview
The Stream API facilitates the use of intermediate, reduction as well as terminal operations on a list of primitive or non-primitive data types. Java Stream reduce() method is the one that provides the stream reduction operation that fetches one single result against a list of elements by processing each of the list elements with some combining operation provided by the user.
Syntax of Java Stream reduce()
Here, T is the type for the identity, and accumulator is a BinaryOperator that accepts two values.
However, if the identity is not assigned initially, there will be no default or initial value, so the reduce() method returns an Optional.
Parameters of Java Stream reduce()
In general, Java Stream reduce() comprises two parameters: the identity and the accumulator.
The identity element is either the initial value of the stream reduction or it can also be the default value if there are no elements present in the stream.
The accumulator is a function that takes up two parameters as well:
- A partial result of the reduction that has been produced by processing the elements of the list so far.
- The next element that has to be processed in the list.
Return Value of Java Stream reduce()
The return value of the Java Stream reduce() will be of type T or it can be an Optional as the result may or may not be empty. For Optional, the isPresent() method returns true or false depending on whether there is a non-null value.
Example
Let's take up an example to find out the sum of all even numbers from a given list of integers. Without the Java stream reduce() method, there would be a long implementation of a for loop that iterates the elements of the list to check whether they are even and then adds them up.
Output:
The reduce() method would create a stream of objects and simply iterates each element where its implementation can be defined in a concise way.
Here, the above example needs a check whether a number is even or odd. So, the lambda expression comprises two arguments; the partial sum till the previous number and the current number to check and add to the sum, if it's even.
The lambda expression in the above example would be:
Since the sum returned would be an integer, we'll store the resultant value in an integer variable. The entire implementation for the reduce() method is:
Code
Output:
What is Java Stream reduce()?
In the case of functional programming, the concept of folding operates on a collection of elements to produce a single result value based on the type of operation a user puts in. The reduce() method exhibits the folding implementation.
The Java Stream reduce() method offers the stream reduction operation that obtains a single result from a list of elements by putting each element into a user-provided operation.
More Examples
The above example demonstrates the use of the Java stream reduce() on the list of integers. Likewise, we could perform the same on the list of strings as well.
Output:
In this example, we have provided the identity as an empty string, which will be combined with each of the strings in the list as the final outcome.
Now, we'll look at an example to find the maximum and minimum of all the numbers present in the list. Now, a check can be provided with the help of a ternary operator in this manner:
Integer.MIN_VALUE is the identity here, so it will be considered as the initial value in this case.
The same thing can be done easily with the max() method present in the Integer class. In this, we don't have to write the implementation for the greatest element with the lambda expression.
Code
Output:
Now, let us create a custom class so that we can see how to reduce method can be applied to a list of objects instead of the primitive data types.
We'll have an example to calculate the total expense from a list of objects that have an itemName, price, and the number of units bought.
Given below is the custom class implementation along with the parameterized constructor.
Also, the list of the objects will be defined in the following manner:
Here, in this case, we'll use a map() to first calculate the total price of each of the items based on their units bought and this will create a map of the total cost for each item. Then, we would apply reduce() method to it to find the total double value, i.e., the totalExpense.
Moreover, the result can be empty if there's no item purchased, so let the result be Optional. When the result is an Optional, we must not define the identity and the first value will be considered by default.
Code
Output:
Conclusion
- The Java Stream reduce() method offers the stream reduction operation that obtains a single result from a list of elements by putting each element into a user-provided operation.
- In the case of functional programming, the concept of folding operates on a collection of elements to produce a single result value based on the type of operation a user puts in. The reduce() method exhibits the folding implementation.
- Java Stream reduce() comprises two parameters: the identity and the accumulator.
- The identity element is either the initial value of the stream reduction or it can also be the default value if there are no elements present in the stream
- The accumulator is a function that takes up two parameters as well:
- A partial result of the reduction that has been produced by processing the elements of the list so far.
- The next element that has to be processed in the list.