endsWith() in Java
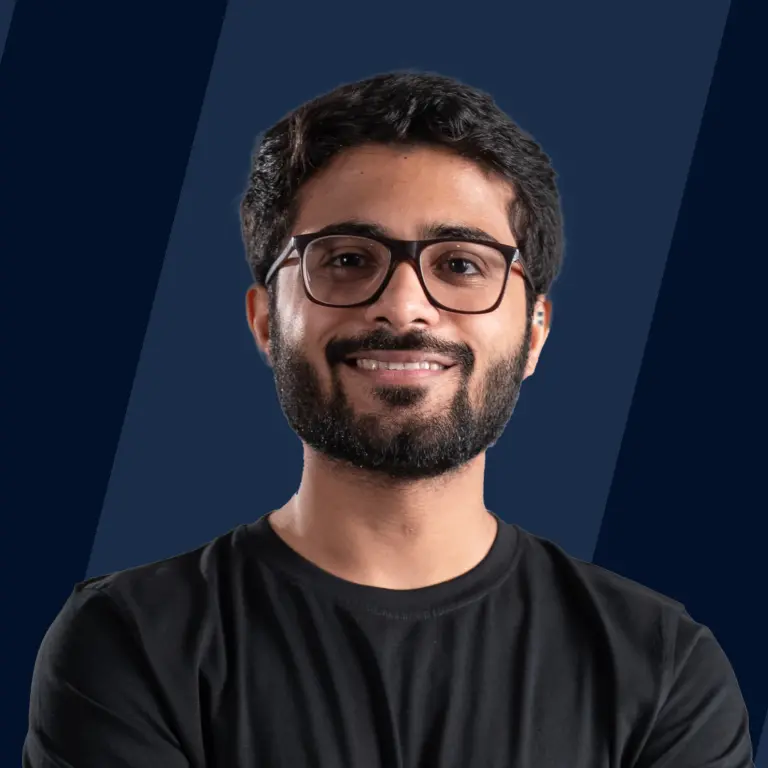
Overview
The Java String endsWith() method is used to check if a string ends with a given suffix or not. If the string ends with a given suffix, it returns true, else it returns false. endsWith() method internally uses the startsWith() method to find the result.
Syntax of endsWith() in Java
The method signature is given below:
Syntax of the endsWith() method is:
Here, "str1" is an object of the String class.
Parameters of endsWith() in Java
endsWith() method takes only one parameter, the suffix(string), and checks whether a string ends with the suffix or not.
Return Value of endsWith() in Java
Return Type: boolean
The function returns true or false as per the following conditions:
Return Value | Condition |
---|---|
false | if the string does not end with the given suffix |
true | if the string ends with the given suffix or the suffix is an empty string |
Exceptions of endsWith() in Java
- endsWith() method throws "NullPointerException" when null is passed as a suffix string.
- endsWith() method throws the error "incompatible types" if we pass an integer or character as a suffix string.
Example of endsWith() in Java
We’ll see an example of the endsWith() method where we'll check if the given string ends with 'for' or 'Academy'.
Output
Code Explanation:
- string.endsWith("for") returns false to variable bool1 as the string does not end with "for".
- string.endsWith("Academy") returns true to variable bool2 as the string ends with "Academy".
Note: The endsWith() method takes care of the case sensitiveness of the characters present in a string.
What is endsWith() in Java String?
endsWith() is a method present in String class of java.lang package. It checks if a string ends with the given suffix or not and returns a boolean value accordingly. We will deep dive to see how endsWith() actually works. Internal code for endsWith() method in jdk8 is given below:
- endsWith(suffix) method calls internally startsWith(prefix,offset) method.
- endsWith(suffix) passes specified string suffix as prefix and length of the input string - length of suffix string as the offset.
- startsWith(prefix, offset) method checks if this string starts with the specified prefix beginning a specified index (offset).
Internal code for startsWith() method in jdk8:
- Since endsWith() directly uses startsWith(), so the time complexity is O(N) due to the one simple while loop in startsWith() method.
For example:
input_string = java-script and input_string.length = 11
suffix_string: script and suffix_string.length = 6
It searches in the input_string from a given position(or index 5 in this case) till the end for the suffix_string. If found, then returns true, else false.
Note:
-
Since validatePopular(String email) method is a static function, we can access it directly without making an object.
-
Since the endsWith() method throws NullPointerException if the suffix is null, we should use a try-catch block to handle this.
More Examples
Example 1: Java endsWith() - checking Domain
We have an array of domain names. We have to check if the domain names end with a given substring.
Output
Example 2: String endsWith() with if Statement
In this part we'll help an artificial intelligence enthusiast filter websites with domain names ending with "ai" from a list of domain names.
Output
Code explanation:
- We have a String array of domain names, out of which we have to print those domain names that end with "ai".
- We loop through the array and use string.endsWith("ai") to check if the domain name ends with "ai", if it does, we print that domain name.
Example 3: Validating a Popular Domain
We'll see a practical application of endsWith() by building a function that checks if an email has a popular domain name with .com at the end.
Output
Code Explanation:
- We have a list of popular email id domains stored in string array comEmailEndings in validatePopular(String email) method.
- When we pass an email id from function to the validatePopular(String email) method, the validatePopular(String email) method checks if the email ends with popular domains names or not using endswith() function.
- Since the endswith() function can throw exceptions, it is a good practice to use try-catch block in the program.
- The above code can be extended to build a function that checks if an email is valid or not, but for that we'll need to have all domain names and some additional information.
Conclusion
- Java String endsWith() method checks whether a string ends with a given suffix or not.
- If the string ends with a given suffix, the endsWith(String suffix) method returns true else false.
- endsWith(String suffix) method throws NullPointerException when the suffix string is null.
- endsWith(String suffix) method throws the error “incompatible types” when the suffix variable is passed as a character or a numeric data type and not a string data type.
- endsWith(String suffix) method internally uses startsWith(String prefix, int offset) method to find the result.