Convert Java String to Character Array
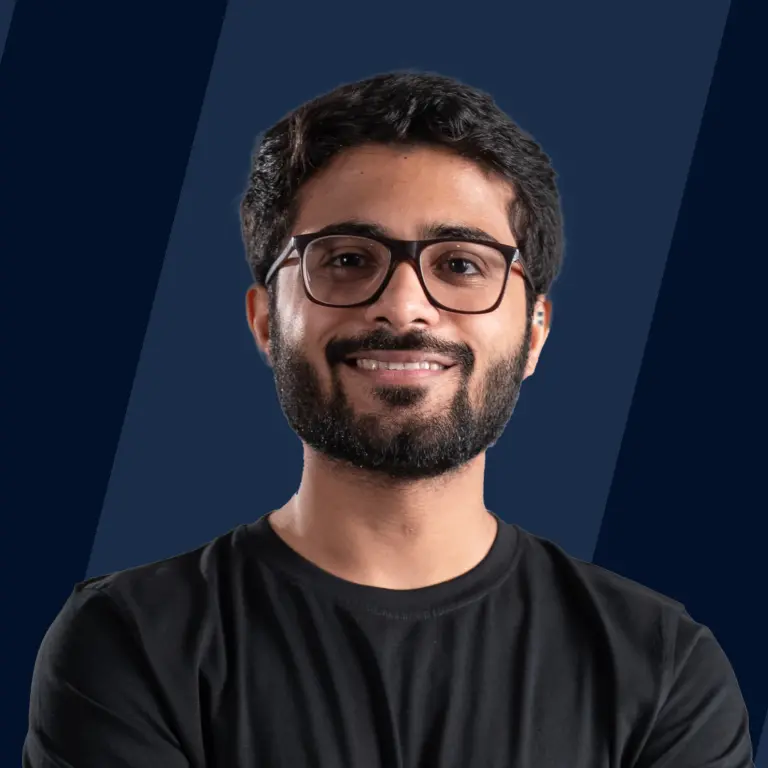
Overview
In this article, we are going to learn about how we can convert Java String to Character Array. Before getting started with the topic, let us get a short overview of what is a String and character array in Java.
String in Java : Basically, a String in Java is an object, which consists of a series or collection of character values in it. Usually, when we want to store any text in Java, we use the String data structure to store it. For instance, if we want to store "Hello World", we will store it in a String type variable String str = "Hello World".
Character array in Java : Basically, a character array is a data structure, which is used to store characters in it. It is similar to an array of char data types. For instance, if we want to store 'H', 'e', 'l', 'l', 'o', we will store the in an array of character type char[] c = {'H', 'e', 'l', 'l', 'o'}.
So let us now begin with the main agenda of our article, Convert Java String to Character Array.
Introduction
In Java, as we know we can store any text element in a String data type variable, in many cases, we are required to get all the characters of that String. Now, we can get characters at any index of the String by just calling the charAt(index) method. However, something in cases like if we want to replace any character from the String, we can't simply use the charAt(index) method, and it will throw an error. For instance, we have a String str = "Hello World", now if we want to replace the 0th index character 'H', and if we are using the charAt(index) method to replace the character at index 0, it will throw an error.
charAt() method : String class consist of many methods, charAt() is one of them. It usually returns the character at any specific index of the String.
Code :
Output:
Explanation :
- In the above code, we have stored the text "Hello World", inside a String type variable.
- We are replacing the 0th index character from the String text with some other character using the charAt() method.
- We can observe, it throws an unexpected type of error. Hence, we cannot replace characters using the charAt() method.
So to solve and successfully execute problems like the above, we need some data structures, and that is character array, we usually convert our String data to a character array, where we can store the character of the string, and then we can replace it. Let us now solve the above problem, using a character array this time, and see what will be our output.
Code:
Output:
Explanation:
- In the above code, we have stored the text "Hello World", inside a String type variable.
- Then, we are creating a char array of string length using the length() method, in which we will store all the characters of the String.
- We are storing the characters of the String using for loop.
- Then, we are replacing the 0th index character from the char array with some other character.
- Then we are again converting the char array to String, and printing the String value.
- We can observe the change in the 0th index character of the String. Hence we have achieved replacing of characters of a String using a char array.
From the above examples, we got a clear idea that converting a String to a character array is required many times in our code. Now, in Java, there are many ways by which we can convert our String to a character array. Let us discuss them in detail.
Java Program to Convert String to Char Array Using a Naive Approach via Loops
Let us begin with the brute force approach of converting a String to a char array. In this approach, we will be converting our String to a char array with the help of loops. Let us discuss this approach.
Intution :
- Firstly we will store a text in a variable of String type.
- Then we will create a char array with the length same as the String length.
- Then we will run a loop starting from 0 till `String's length.
- Inside the loop we will store the characters of the String in the character array.
Code:
Output:
Explanation:
- In the above code, we have stored the text "Hello World", inside a String type variable.
- Then, we are creating a char array of string length using the length() method, in which we will store all the characters of the String.
- Then we are copying character by character into an array using for loop.
- Then we are printing the elements of an array using for loop.
Time complexity:
Space Complexity:
Java Program to Convert String to Char Array Using toCharArray() Instance Method
Let us now look at another approach to converting a String to a char array. In this approach, we will be using the toCharArray() method for converting our String to a char array. Let us discuss the intuition of this approach.
Note : This toCharArray() method is very important as in most interviews an approach is seen mostly solved using this method.
Intution :
- Firstly we will store a text in a variable of String type.
- Then we will create a char array with the length the same as the `String's length.
- Then we will store the array in the char array which we will be getting from the toCharArray() method.
- Then we will print the char array.
Code:
Output:
Explanation:
- In the above code, we have stored the text "Hello World", inside a String type variable.
- Then, we are creating a char array of string length and store the array returned by the toCharArray() method
- Then we are printing the elements of an array using for loop.
Time complexity:
Space Complexity:
Java Program to Convert String to Char Array Using charAt() Instance Method
Let us now look at another approach to converting a String to a char array. In this approach, we will be using the charAt() instance method for converting our String to a char array. Let us discuss the intuition of this approach.
Intution :
- Firstly we will store a text in a variable of String type.
- Then we will create a char array with the length the same as the `String's length.
- Then we will run a loop starting from 0 till `String's length.
- Inside the loop, firstly we will get the index-wise characters of the String, using the charAt() method.
- Then we will store the characters of the String in the character array.
- Then we will print the character array.
Code:
Output:
Explanation:
- In the above code, we have stored the text "Hello World", inside a String type variable.
- Then, we are creating a char array of string length using the length() method, in which we will store all the characters of the String.
- Then we are copying character by character, which we are fetching by the charAt() method, into an array using for loop.
- Then we are printing the elements of an array using for loop.
Time complexity:
Space Complexity:
Java Program to Convert String to Char Array Using Java 8 Stream
In Java 8, we have the Stream API, before getting started with how we can convert our String to char array, let us discuss about what is a Stream API in Java.
Stream in Java : To process the collections of objects, we use the Stream API in Java. A stream is a collection of objects that can support several operations and be pipelined to create the desired outcome.
Now, char Stram is not provided by Java 8, however, when dealing with String, using the String.chars() method we can get the IntStream (integer type Stream) of characters.
After getting the IntStream of characters, we need to convert the IntStream to Stream<Character>. We can usually achieve this by creating char using the lambda function ch -> (char) ch inside the mapToObj(), which will then automatically get converted into characters.
In the end, we can use the toArray() method to convert the Stream into a newly allocated character array.
As we understood what Stream API is and how we can use it to convert our String to a char array, let us now look at the code, for more clear understanding.
Code:
Output:
Explanation:
- In the above code, firstly we are importing the classes from the Java.util package.
- Then inside the main method, we are storing the custom input String text, inside a String type variable.
- Then we are creating a character array of string length, and inside which we are storing the returned character array value, which we are getting after calling the function toCharacterArray(str).
- Inside the toCharacterArray(str) function, we are sending the String value as the parameter, and the return type of the function is a character array.
- Inside the toCharacterArray(str) function, firstly we are checking whether the String value is null or not. If the value is null, we will return a null value.
- Then, we are calling the chars() method (str.chars()), to get our IntStream.
- After getting the IntStream of characters, we need to convert the IntStream to Stream<Character>. We can usually achieve this by creating char using the lambda function ch -> (char) ch inside the mapToObj(), which will then automatically get converted into characters.
- Then we can use the toArray() method to convert the Stream into a newly allocated character array.
- At the end we are returning the character array from the toCharacterArray(str) function.
- Then we are printing the elements of an array using for loop.
There is another method, using which we can get the IntStream, and that is String.codePoints(). Let us look at an example of how we can do this.
Code:
Output:
Explanation:
- In the above code, firstly we are importing the classes from Java.util package.
- Then inside the main method, we are storing the custom input String text, inside a String type variable.
- Then we are creating a character array of string length, and inside which we are storing the returned character array value, which we are getting after calling the function toCharacterArray(str).
- Inside the toCharacterArray(str) function, we are sending the String value as the parameter, and the return type of the function is a character array.
- Inside the toCharacterArray(str) function, firstly we are checking whether the String value is null or not. If the value is null, we will return a null value.
- Then, we are calling the codePoints() method (str.codePoints()), to get our IntStream.
- After getting the IntStream of characters, we need to convert the IntStream to Stream<Character>. We can usually achieve this by creating char using the lambda function ch -> (char) ch inside the mapToObj(), which will then automatically get converted into characters.
- Then we can use the toArray() method to convert the Stream into a newly allocated character array.
- At the end we are returning the character array from the toCharacterArray(str) function.
- Then we are printing the elements of an array using for loop.
Time complexity:
Space Complexity:
Java Program to Convert String to Char Array Using .getChars() Method
There is another, bit more modified approach to convert the String value to a char array, and that is by using the .getChars() method. Let us look at the syntax of this method first, and discuss it in detail.
Syntax:
Parameters:
There is a total of four parameters inside our getChars() method.
- srcBegin : It is the beginning index from where we want our characters of the String to get converted into a character array. It is of integer type.
- srcEnd : It is the ending index till where we want our character of the String to get converted into a character array. It is of integer type.
- dst : It is the destination character array, where we want to store the characters of the String. It is of char array type.
- desBegin : It is the starting index, from where the dst array should store the characters of the String. It is of integer type.
Return Type:
The getChars method, does not return any value, that is, the return type of this method is void.
When we want, instead of all the characters, only a subset of the String to get converted into the character array, we use this getChar() method. Please keep in mind that, if we want only a single character from the String, we can use the charAt(index) method, to get the char value at the specified position in the String.
Now, let us look at the code of how we can use the getChar() method to convert the String to a char array, for more clear understanding.
Code:
Output:
Explanation:
- In the above code, firstly we are importing the classes from the Java.util package.
- Then inside the main method, we are storing the custom input String text, inside a String type variable.
- Then we are creating a character array, and here it is not necessary to make it of length equal to String length. We can just make it big enough to fit the characters of the String we want to convert into a character array.
- Then we are copying the "World" substring from the string to the char array. We have used the getChar() method, in which we have sent the starting index of the "World" substring, then the ending index of the "World" substring, then the destination array, and lastly the starting index, from where it should store inside the destination array, as the parameter.
- Then we are printing the elements of the char array using for loop.
Time complexity:
Space Complexity:
Conclusion
In this article, we learned about how to get convert Java String to character Array in java. Let us recap the points we discussed throughout the article:
- String in Java is an object, which consists of a series or collection of character values in it.
- Character array is a data structure, which is used to store characters in it. It is similar to an array of char data types.
- In Java there are many ways by which we can convert our String to a character array.
- The naive approach of converting a String to a char array is by using a for a loop.
- Another approach to converting a String to a char array is using the toCharArray() method.
- Another approach to converting a String to a char array is using the charAt() instance method.
- Another approach to converting a String to a char array is using the Java 8 Stream API.
- To process the collections of objects, we use the Stream API in Java. A stream is a collection of objects that can support several operations and be pipelined to create the desired outcome.
- Now, char Stram is not provided by Java 8, however, when dealing with String, we can use both the String.chars(), and String.codePoints() methods to get the IntStream (integer type Stream) of characters.
- After getting the IntStream of characters, we need to convert the IntStream to Stream<Character>. We can usually achieve this by creating char using the lambda function ch -> (char) ch inside the mapToObj(), which will then automatically get converted into characters.
- At the end we can use the toArray() method to convert the Stream into a newly allocated character array.
- There is another, bit more modified approach to convert the String value to a char array, and that is by using the .getChars() method.
- When we want, instead of all the characters, only a subset of the String to get converted into the character array, we use this getChar() method.