Java Timer Class
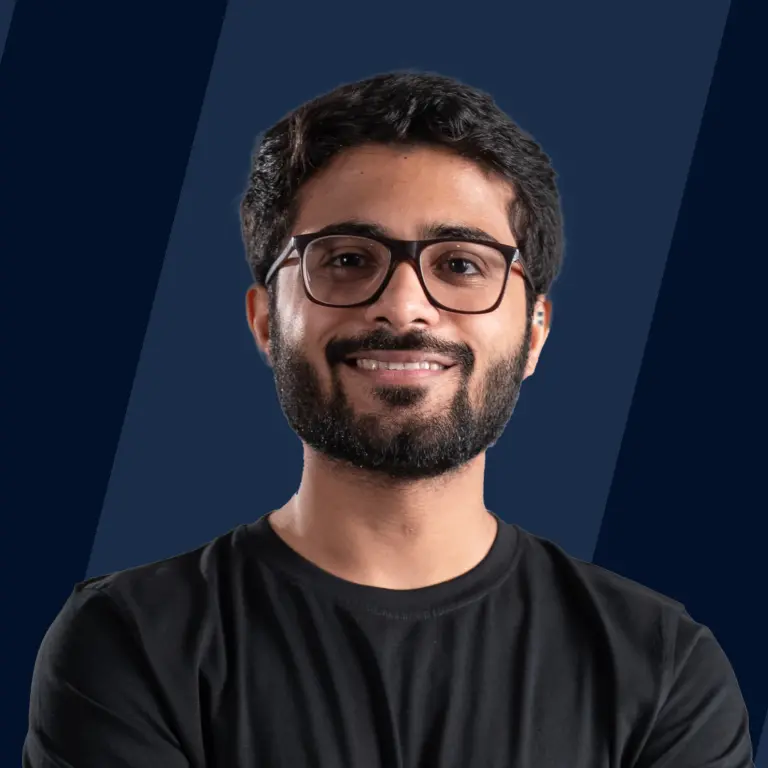
Overview
In this article, we are going to learn about Java timer classes. Before getting started with the topic let us have a short overview of the Java timer class
Java Timer Class : In Java, whenever we are required to schedule a task or run a block of code after some regular instant of time, we use this Java Timer Class. Using the Timer class, we can run or schedule the task for once, or for a repeated number of executions. Each timer object has a background thread attached to it that is in charge of the execution of all the tasks of a timer object.
So let us now begin with the main agenda of our article, Java Timer Class.
Introduction to Java Timer Class
Java Timer class is a class in Java, which belongs to the Java.util package. and we import it as Java.util.Timer. In Java, whenever we are required to schedule a task or run a block of code after some regular instant of time, we use this Java Timer Class. Using the Timer class, we can run or schedule the task for once, or for a repeated number of executions. Each timer object has a background thread attached to it that is in charge of the execution of all the tasks of a timer object. Java Timer class is multi-threaded, that is, a single Java Timer class object can be shared by multiple threads, thereby making it thread-safe. All the scheduled tasks of a Java Timer class are stored in the binary heap data structure.
Important Notes:
- Java Timer class object is multi-threaded, and a single Java Timer class object can be shared by multiple threads.
- Java Timer class object is thread-safety.
- All the scheduled tasks of a Java Timer class are stored in the binary heap data structure.
Declaration
Let us now see, how we can declare a Timer class in Java.
Syntax:
We can create an object of the timer class as follows:
Constructors of Java Timer
Certain varieties of parameterized constructors are provided by Java, for the Timer class object. Let us discuss them in detail.
Constructors | Description |
---|---|
Timer() | This is the default constructor of the Timer class, which does not contain any parameter, and will create an object of the Timer class. |
Timer(boolean isDaemon) | This is the parameterized constructor of the Timer class, which consist of a boolean variable as a parameter. It basically creates a new Timer object whose connected thread may be identified to run as a daemon. Basically, Daemon is a background process that does little jobs like garbage collection and has a low priority. |
Timer(String name) | This is the parameterized constructor of the Timer class, which consist of a String variable as a parameter. It basically creates a new Timer object whose connected thread has its own specified name. |
Timer(String name, boolean isDaemon) | This is the parameterized constructor of the Timer class, which consists of two parameters of String and boolean type. It basically creates a new Timer object whose connected thread will have its own specified name, and may be identified to run as a daemon. |
Constructor Detail
Let us discuss the constructors of the Timer class in Java in detail.
Timer(): This is the default constructor of the Timer class, which does not contain any parameter, and will create an object of the Timer class.
Syntax:
Timer(boolean isDaemon): This is the parameterized constructor of the Timer class, which consists of a boolean variable as a parameter. It basically creates a new Timer object whose connected thread may be identified to run as a daemon. Basically, Daemon is a background process that does little jobs like garbage collection and has a low priority.
Syntax:
Timer(String name): This is the parameterized constructor of the Timer class, which consist of a String variable as a parameter. It basically creates a new Timer object whose connected thread has its own specified name.
Syntax:
Parameters:
name - String parameter, It basically creates a new Timer object whose connected thread has its own specified name.
Timer(String name, boolean isDaemon): This is the parameterized constructor of the Timer class, which consists of two parameters of String and boolean type. It basically creates a new Timer object whose connected thread will have its own specified name, and may be identified to run as a daemon.
Syntax:
Parameters:
- name - String parameter, It basically creates a new Timer object whose connected thread has its own specified name.
- isDaemon - Boolean parameter, to check whether the task is to be run as a daemon.
Basically, Daemon is a background process that does little jobs like garbage collection and has a low priority.
Methods of Java Timer
Methods | Description |
---|---|
void cancel() | The void cancel() method ceases the timer, dropping any currently scheduled task. This method has no return type. |
int purge() | The int purge() method cleans all the discarded tasks from this timer's task queue. This method is of integer type. |
void schedule(TimerTask task, Date time) | This void schedule(TimerTask task, Date time) method takes two parameters, one is the task, and another is the time. It basically, schedules the specified task for execution at the specified time. This method has no return type. |
void schedule(TimerTask task, Date firstTime, long period) | This void schedule(TimerTask task, Date firstTime, long period) method takes three parameters, the task, the firstTime, and the time period. It basically, arranges for the provided job to be executed repeatedly with a defined delay, starting at the supplied time. |
void schedule(TimerTask task, long delay) | This void schedule(TimerTask task, long delay) method takes two parameters, one is the task, and another is the delay time. It basically, schedules the specified task for execution after the specified delay. This method has no return type. |
void schedule(TimerTask task, long delay, long period) | This void schedule(TimerTask task, long delay, long period) method takes three parameters, the task, the delay time, and the time period. It basically, arranges for the provided job to be executed repeatedly with a defined delay, starting after the supplied delay. |
void scheduleAtFixedRate(TimerTask task, Date firstTime, long period) | This void scheduleAtFixedRate(TimerTask task, Date firstTime, long period) method takes three parameters, the task, the firstTime and the time period. It basically, arranges for the provided job to be executed repeatedly at a predetermined pace, starting at the stated time. |
void scheduleAtFixedRate(TimerTask task, long delay, long period) | This void scheduleAtFixedRate(TimerTask task, long delay, long period) method takes three parameters, the task, the delay time, and the time period. It basically, Schedules the specified task for repeated fixed-rate execution, beginning after the specified delay. |
Methods Detail
Let us discuss all the methods of the Timer class in Java, in detail along with their syntax, and code.
void cancel(): The void cancel() method ceases the timer, dropping any currently scheduled task. This method has no return type.
Syntax:
int purge(): The int purge() method cleans all the discarded tasks from this timer's task queue. This method is of integer type.
Syntax:
void schedule(TimerTask task, Date time): This void schedule(TimerTask task, Date time) method takes two parameters, one is the task, and another is the time. It basically, schedules the specified task for execution at the specified time. This method has no return type.
Syntax:
void schedule(TimerTask task, Date firstTime, long period): This void schedule(TimerTask task, Date firstTime, long period) method takes three parameters, the task, the firstTime, and the time period. It basically, arranges for the provided job to be executed repeatedly with a defined delay, starting at the supplied time.
Syntax:
Code:
Output:
Explanation:
- In the above code, firstly we are importing the Timer and the TimerTask Class from the Java.util package.
- Inside the main method we are creating a new object of the Timer class timer.
- Then we are creating an object of the Helper class, which is inheriting the TimerTask class. The Helper class is basically performing some tasks. The above code consists of a run() method, which is printing some values.
- Then we are calling the schedule method which consists of three parameters. The first parameter is the task, then the firstTime at which task is to be executed, then the time in milliseconds between successive task executions.
- We can observe how the task is been printed in a time gap of 5000 milliseconds.
void schedule(TimerTask task, long delay): This void schedule(TimerTask task, long delay) method takes two parameters, one is the task, and another is the delay time. It basically, schedules the specified task for execution after the specified delay. This method has no return type.
Syntax:
void schedule(TimerTask task, long delay, long period): This void schedule(TimerTask task, long delay, long period) method takes three parameters, the task, the delay time, and the time period. It basically, arranges for the provided job to be executed repeatedly with a defined delay, starting after the supplied delay.
Syntax:
void scheduleAtFixedRate(TimerTask task, Date firstTime, long period): This void scheduleAtFixedRate(TimerTask task, Date firstTime, long period) method takes three parameters, the task, the firstTime, and the time period. It basically, arranges for the provided job to be executed repeatedly at a predetermined pace, starting at the stated time.
Syntax:
void scheduleAtFixedRate(TimerTask task, long delay, long period): This void scheduleAtFixedRate(TimerTask task, long delay, long period) method takes three parameters, the task, the delay time, and the time period. It basically, Schedules the specified task for repeated fixed-rate execution, beginning after the specified delay.
Syntax:
Code:
Output:
Explanation:
- In the above code, firstly we are importing the Timer and the TimerTask Class from the Java.util package.
- Inside the main method we are creating a new object of the Timer class timer.
- Then we are creating an object of the Helper class, which is inheriting the TimerTask class. The Helper class is basically performing some tasks. The above code consists of a run() method, which is printing some values.
- Then we are creating the instance of the date object for fixed-rate execution.
- Then we are calling the scheduleAtFixedRate method which consists of three parameters. The first parameter is the task, then the delay which will delay in milliseconds before the task is to be executed, then the time in milliseconds between successive task executions.
- Then we are running the synchronized(obj) thread block.
- Inside the synchronized(obj) thread block, we are making the main thread wait, using the wait() method.
- Then once the timer has scheduled the task 4 times, the main thread resumes and terminates the timer.
- Then we are purging to remove all canceled tasks from the timers task queue.
Java Timer Class Examples
Let us discuss some examples of Java Timer class.
1. Java Timer schedule() Method
Let us now look at the example of the Java Timer schedule() method, for more clear understanding.
Abstract:
Here in this code, we will see how Java Timer consists of the feature of scheduling any specified task for repeated execution with a fixed delay, with the task having some specified start time.
Code:
Output:
Explanation:
- In the above code, firstly we are importing the Timer and the TimerTask Class from the Java.util package.
- Inside the main method we are creating a new object of the Timer class timer.
- Then we created an instance of the Helper class which is inheriting the TimerTask class. Inside the Helper class, we have initialized a variable using which we will check the number of times the task is executed.
- Using the run() method of the Helper class we are printing the number of times the task is executed.
- Then in the main method, we have used the void schedule(TimerTask task, Date firstTime, long period) method, which consists of three parameters. The first parameter is the task, then the firstTime at which task is to be executed, then the time in milliseconds between successive task executions.
- Using the schedule() method we can execute the run() method as many times as we want.
2. Java Timer cancel() Method
Let us now look at the example of the Java Timer cancel() method, for more clear understanding.
Abstract:
Here in this code, we will see how Java Timer consists of the cancel() method, which is used to cease the timer, dropping any currently scheduled task.
Code:
Output:
Explanation:
- In the above code, firstly we are importing the Timer and the TimerTask Class from the Java.util package.
- Inside the main method we are creating a new object of the Timer class timer.
- Then we created an instance of the TimerTask class. Inside the TimerTask class, we are calling the run() method to carry out the action of the task.
- Inside the run() method, we are running a loop, we can observe, the loop will keep executing, even after the first "Stop calling" statement, which is encountered when the loop is reached 3.
3. Java Timer purge() Method
Let us now look at the example of the Java purge() method, for more clear understanding.
Abstract:
Here in this code, we will see how the Java Timer purge() method cleans all the discarded tasks from this timer's task queue.
Code:
Output:
Explanation:
- In the above code, firstly we are importing the Timer and the TimerTask Class from the Java.util package.
- Inside the main method we are creating a new object of the Timer class timer.
- Then we created an instance of the TimerTask class. Inside the TimerTask class, we are calling the run() method to carry out the action of the task.
- Inside the run() method, we are running a loop, we can observe, the loop will keep executing, and just after the cancel() method, a break statement is given. Due to this, the coop will break when i become 3.
- Now, when we come out of the loop, we are returning the number of tasks which is been removed from the queue. For that, we are using the purge() method, which will clean all the discarded tasks from this timer's task queue.
Conclusion
In this article, we learned about how to get the Java Timer class. Let us recap the points we discussed throughout the article:
- In Java, whenever we are required to schedule a task or run a block of code after some regular instant of time, we use this Java Timer Class.
- Java Timer class object is multi-threaded, and a single Java Timer class object can be shared by multiple threads.
- Java Timer class object is thread-safety.
- All the scheduled tasks of a Java Timer class are stored in the binary heap data structure.
- Certain varieties of parameterized constructors are provided by Java, for the Timer class object.
- Timer(), Timer(boolean isDaemon), Timer(String name), Timer(String name, boolean isDaemon) are different constructors of Java Timer class.
- Different methods of Timer class in Java are, void cancel(), int purge(), void schedule(TimerTask task, Date time), void schedule(TimerTask task, Date firstTime, long period), void schedule(TimerTask task, long delay), void schedule(TimerTask task, long delay, long period), void scheduleAtFixedRate(TimerTask task, Date firstTime, long period), void scheduleAtFixedRate(TimerTask task, long delay, long period). We have discussed them in detail.
- We have also seen some examples of the Java Timer class.