Java Tokens
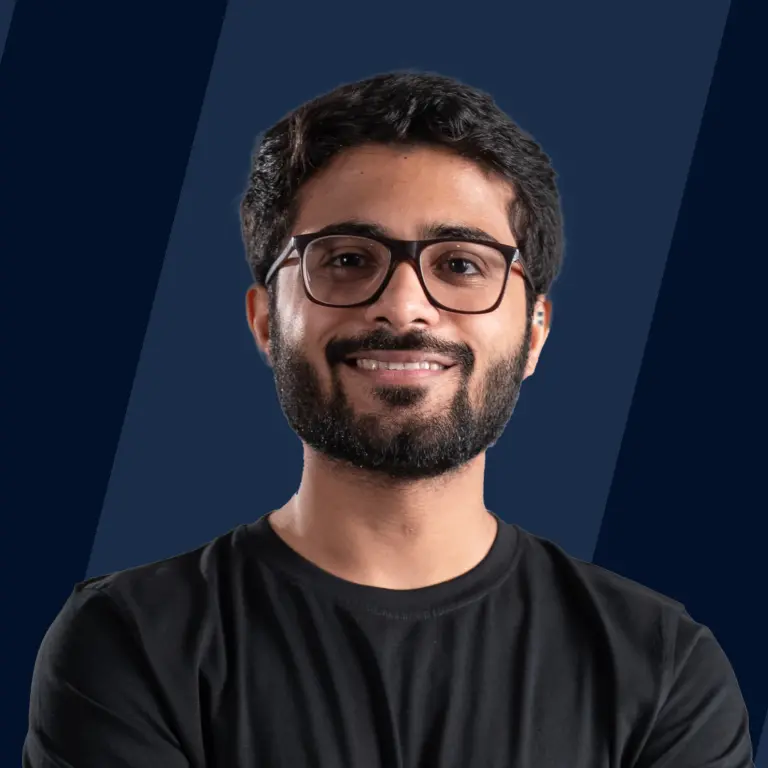
Overview
Java tokens are like the building blocks of a secret code that computers understand. They're tiny parts of a program, such as words, symbols, and numbers, that together create instructions for the computer to follow. Imagine each token as a special word in a secret language. There are important words (keywords) that tell the computer what to do, names (identifiers) that give things unique titles, numbers (literals) for counting, and signs (operators) that do math or make decisions. Spaces and punctuation marks (separators) help organize the tokens, just like spaces between words in sentences.
What are Tokens in Java?
In Java, tokens are the smallest units of a program's source code. Here's a breakdown of the different types of tokens in Java:
-
Keywords:
Keywords are reserved words that have specific meanings and functionalities within the Java language. Examples include class, public, static, void, if, else, while, and many others.
-
Identifiers:
Identifiers are names given to various program elements such as classes, methods, variables, and labels. They are used to uniquely identify these elements within the code. Identifiers must follow certain naming rules, like starting with a letter, dollar sign, or underscore, and then followed by letters, digits, underscores, or dollar signs.
-
Literals:
Literals are constant values that appear directly in the code. They represent values such as numbers, characters, strings, and boolean values. Examples include 123, 'a', "Hello, world!", and true.
-
Operators:
Operators are symbols used to perform various operations on variables and values. They include arithmetic operators (+, -, *, /, %), assignment operators (=, +=, -=), comparison operators (==, !=, <, >, <=, >=), logical operators (&&, ||, !), and more.
-
Separators:
Separators are symbols used to separate different parts of the code and define the structure of the program. Common separators include parentheses (), braces {}, square brackets [], commas ,, semicolons ;, periods ., and more.
Keywords
A Java keyword is a reserved word in the Java programming language that holds a predefined meaning and functionality. These words are part of the language's syntax and have specific purposes, directing the compiler to perform certain actions or operations. Keywords cannot be used as identifiers (such as variable names or class names) and play a crucial role in defining the structure, behavior, and flow of Java programs. For more detailed learning of the topic, you can follow What is Keyword in Java?
Identifiers
In Java programming, an identifier is like a special name you give to different things in your code, such as names for your classes, functions, or pieces of information. It's like labeling stuff in your room so you can find them easily. These names help you understand what each thing does without having to look at the details. But there are rules for making these names. They have to start with a letter, underscore (_), or dollar sign ($). After that, you can use letters, numbers, underscores, or dollar signs. Also, the names you use need to match exactly, even if you change the size of the letters. By picking good names that follow these rules, your code becomes clearer, and it's easier for everyone to work together on the same project. For more detailed learning of the topic, you can follow Identifiers in Java with Examples
Constants
Here's a detailed explanation of constants in Java:
-
Declaring Constants:
To declare a constant in Java, the final modifier is used to indicate that the value of the variable cannot be changed once it is assigned. Constants can be declared in various scopes, including class-level (static constants) or within methods (local constants). Example of a class-level constant:
Example of a local constant within a method:
-
Naming Conventions for Constants:
Constants are often named using uppercase letters with underscores between words. This convention improves readability and makes it clear that the value is a constant. Example constant names:
- PI
- MAX_VALUE
- DEFAULT_TIMEOUT
- ERROR_MESSAGE
-
Advantages of Constants:
- Readability: Constants provide meaningful names for values, making the code easier to understand.
- Maintainability: By centralizing constant values, changes can be made in one place, reducing the risk of errors.
- Compile-Time Checks: Constants are resolved at compile time, allowing the compiler to catch typos or invalid values.
- Preventing Accidental Changes: Marking values as constants prevents accidental modification, ensuring data integrity.
-
Usage of Constants:
Constants are commonly used for values that are used repeatedly throughout the codebase, such as mathematical constants (Math.PI), configuration values, error codes, and more. Example of using a constant in code:
For more detailed learning of the topic, you can follow Constant in Java with Examples
Special Symbols
Here's an overview of some commonly used special symbols in Java:
-
Parentheses () and Braces {}:
- Parentheses are used to enclose method arguments, expressions, and control flow conditions.
- Braces define code blocks, such as method bodies, loops, and class definitions.
-
Square Brackets []:
Used for declaring arrays and accessing elements within arrays.
-
Semicolon ;:
Used to terminate statements in Java.
Each complete statement must end with a semicolon.
-
Comma ,:
Used to separate items in lists, such as method arguments or array elements.
-
Period .:
Used for accessing members of a class or package, such as methods and fields.
-
Assignment Operator =:
Used to assign a value to a variable.
For example, int x = 10; assigns the value 10 to the variable x.
-
Arithmetic Operators +, -, *, /, %:
Used for basic mathematical operations like addition, subtraction, multiplication, division, and modulus.
-
Comparison Operators ==, !=, <, >, <=, >=:
Used to compare values and return boolean results.
-
Logical Operators &&, ||, !:
Used for combining and negating boolean expressions.
Operators
Here's an overview of the main categories of operators in Java:
- Arithmetic Operators:
- +: Addition
- -: Subtraction
- *: Multiplication
- /: Division
- %: Modulus (remainder of division)
- Assignment Operators:
- =: Assigns a value to a variable.
- +=, -=, *=, /=, %=: Compound assignment operators that combine an operation with assignment.
- Increment and Decrement Operators:
- ++: Increments a variable by 1.
- --: Decrements a variable by 1.
- Comparison Operators:
- ==: Equal to
- !=: Not equal to
- <: Less than
- >: Greater than
- <=: Less than or equal to
- >=: Greater than or equal to
- Logical Operators:
- &&: Logical AND
- ||: Logical OR
- !: Logical NOT
- Bitwise Operators:
- &: Bitwise AND
- |: Bitwise OR
- ^: Bitwise XOR (exclusive OR)
- ~: Bitwise NOT (complement)
- <<: Left shift
- >>: Right shift (with sign extension)
- >>>: Right shift (without sign extension)
- Conditional (Ternary) Operator:
- ? :: Evaluates a boolean expression and returns one of two values depending on the result.
- Instanceof Operator:
- instanceof: Checks if an object is an instance of a particular class or interface.
- Type Cast Operator:
- (type) expression: Converts the result of an expression to the specified data type.
- Bitwise Shift Operators:
- <<: Left shift
- >>: Right shift with sign extension
- >>>: Right shift without sign extension
- Unary Operators:
- +: Positive value (usually redundant)
- -: Negation
- ~: Bitwise complement
- !: Logical NOT
- ++: Increment
- --: Decrement
For more detailed learning of the topic, you can follow Operators in Java with Examples
FAQs
Q. What are Java tokens?
A. Java tokens are the smallest units of a program, including keywords, identifiers, literals, operators, and separators.
Q. Why is proper tokenization important in Java?
A. Proper tokenization ensures adherence to syntax rules and enables the compiler to understand code structure.
Q. Are Java keywords considered tokens?
A. Yes, Java keywords are recognized as distinct tokens that direct the compiler's understanding of code intent.
Conclusion
- Foundation of Java Code: Java tokens are the building blocks of a Java program, encompassing keywords, identifiers, literals, operators, and separators. They define the syntax and semantics of the language.
- Compiler Understanding: Tokenization is the process of breaking down source code into tokens, facilitating the compiler's understanding of the code's structure and meaning during compilation.
- Role in Syntax and Semantics: The precise arrangement and usage of tokens determine a program's functionality and behavior. Correctly structured tokens ensure adherence to the language's syntax rules and enable successful compilation.
- Keyword Importance: Keywords are reserved words that have predefined functionalities in Java. They guide the compiler to perform specific actions and provide consistency in code interpretation.
- Coding Proficiency: Mastery of tokens is fundamental for writing readable, maintainable, and functional Java code. Proper usage of keywords, identifiers, operators, and other tokens leads to well-structured programs and efficient development.