Java Tuple
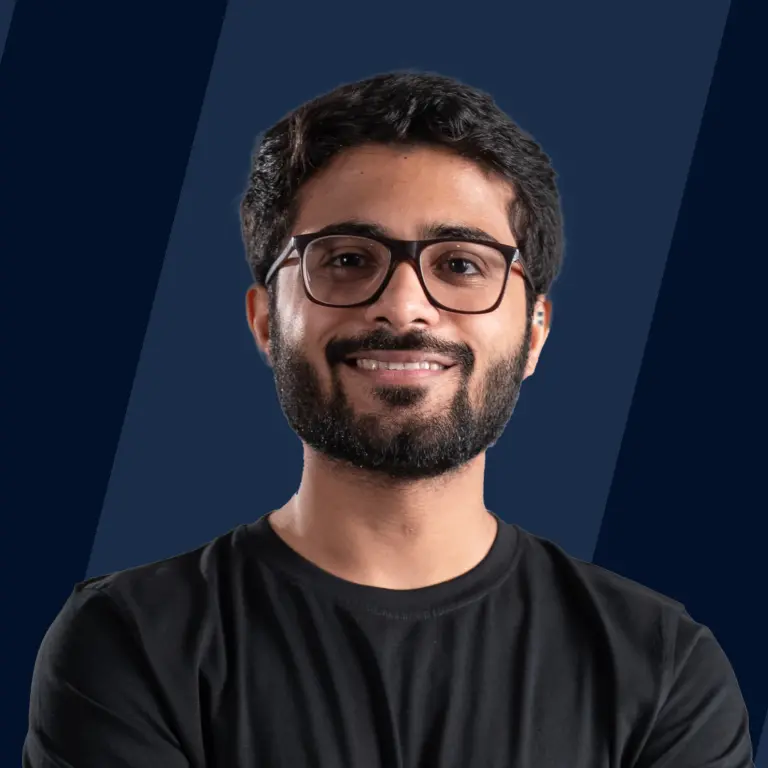
Overview
A Tuple is another data structure that can store objects of different types. These elements may or may not be related to each other. The meaning of the word tuple also sounds similar - "data structure consisting of multiple parts". Tuples come into the picture to overcome the design issues of Arrays and Lists in Java which we only store homogeneous data, and when we require to assign values only once (data should remain unmodified). Now let's explore the Java Tuple class in more detail.
Introduction to Java Tuple Class
Java does not have a built-in Tuple type, but we can use a simple 3rd-party library called org.javatuples. It offers various classes, functions, and data structures to work with Tuples. The Java Tuple class provided by the library is an ordered collection of heterogeneous objects. A byte array stores a Tuple in Java as an object. The comma-separated values are stored and enclosed inside []. Example:
Declaration
The declaration, as of javatuples version 1.2, is:
The Java Tuple class is an abstract base class for all the different tuple classes provided by the library.
Characters of Java Tuple
Tuple Java class has the following characteristics:
- Type Safe
- Iterable
- Immutable
- Serializable
- Implements the Comparable interface
- Implements the toString(), equals() & hashCode() methods.
Java Tuple Subclasses
There are 10 different Java Tuple classes offered which depend on the number of elements allowed (one to ten). All of them are the subclass of the main Java Tuple abstract classes. These are depicted in the following table:
Size of Tuple | Tuple Class Name | General Example |
---|---|---|
One Element | Unit | Unit<A> |
Two Elements | Pair | Pair<A,B> |
Three Elements | Triplet | Triplet<A,B,C> |
Four Elements | Quartet | Quartet<A,B,C,D> |
Five Elements | Quintet | Quintet<A,B,C,D,E> |
Six Elements | Sextet | Sextet<A,B,C,D,E,F> |
Seven Elements | Septet | Septet<A,B,C,D,E,F,G> |
Eight Elements | Octet | Octet<A,B,C,D,E,F,G,H> |
Nine Elements | Ennead | Ennead<A,B,C,D,E,F,G,H,I> |
Ten Elements | Decade | Decade<A,B,C,D,E,F,G,H,I,J> |
Apart from these, there are two more classes, KeyValue<A,B> and LabelValue<A,B>. These are similar to Pair<A,B> but are different in terms of semantics.
Constructors Detail
The generic version of the constructor Java Tuple class is given below. You just need to replace the TupleName with the tuple of appropriate size and parameters.
Example:
Methods of Java Tuple
Most methods of Tuple in Java are public and final except for the getSize() which is public and abstract. These are depicted in the below table:
Method | Syntax | Description | Time Complexity |
---|---|---|---|
contains() | public final boolean contains(Object value) | It checks if the tuple has a specific element or not. | O(n) |
containsAll() | public final boolean containsAll(Collection<?> collection) | It returns true if this tuple contains all of the elements of the specified collection (List/Array). | O(m*n) |
equals() | public final boolean equals(Object obj) | Overrides the equals() method of the Object class. | O(n) |
getSize() | public abstract int getSize() | It returns the size of the tuple. | O(1) |
getValue() | public final Object getValue(int pos) | Get the value at a specific position in the tuple. This method has to return the object, so using it you will lose the type-safety you get with the getValueX() methods. | O(1) |
hashCode() | public final int hashCode() | It returns a hash code for the string. It overrides the hashCode() method of the Object class. | O(1) |
indexOf() | public final int indexOf(Object value) | It returns the index of the first occurrence of the value passed if found, otherwise, return -1. | O(n) |
iterator() | public final Iterator<Object> iterator() | It returns an iterator over the elements in this tuple in the proper sequence. | O(1) |
lastIndexOf() | public final int lastIndexOf(Object value) | It returns the last index of the last occurrence of the specified value if found, otherwise, returns -1. | O(n) |
toArray() | public final Object[] toArray() | It converts the tuple into an array. | O(1) |
toString() | public final String toString() | It returns a string representation of the object. Overrides the toString() method of the Object class. | O(n) |
toList() | public final List<Object> toList() | It converts the tuple into a list. | O(n) |
- n: Tuple's Size
- m: Collection's Size
Methods Detail
The methods of the Java Tuple class discussed above are common to all Tuple subclasses. However, there are more methods associated with subclasses. Those methods are described (along with the ones above):
1. Java Tuple add() Method
add() is used to add value to an existing tuple. This results in a new tuple with one more valuable than the previous one. It happens because Java Tuples are immutable. For instance, adding a value to Triplet transforms it into a Quartet tuple. Decade being the highest class available does not support it.
Syntax:
Parameters:
- n: Number of values as specified by the Tuple class
- types: Type of the values passed
- values: Values passed
Return Value: Returns the object of an appropriate Java Tuple subclass based on the number of values passed as arguments.
2. Java Tuple addAtX() Method
addAtX() method adds a value, at an index X to an existing tuple. Similar to the add() method, adding another value results in a new tuple. It can be used with any Java Tuple subclass except for the Decade class.
Syntax:
Parameters:
- X: Index at which the values will be inserted
- n: Number of values as specified by the Tuple class
- types: Type of the values passed
- values: Values passed
Return Value: Returns the object of an appropriate Java Tuple subclass based on the number of values passed as arguments. The object contains n number of values inserted at index X.
3. Java Tuple compareTo() Method
The compareTo() method is used to compare the order of a tuple object with another tuple object passed in the parameter. It can be used with any Tuple subclass.
Syntax:
Parameters:
- tuple1: Tuple object 1
- tuple2: Tuple object 2 to be compared with tuple1
Return Value: Returns the result of compareTo() for the 1st different element present in the passed object. The value can be negative, 0, or positive.
4. Java Tuple contains() Method
contains() method is used to check for the presence of a value in the given Tuple. It can be used with any Tuple class object.
Syntax:
Parameters: value: The value whose presence is to be checked within the Tuple object.
Return Value: Returns true if the value passed as parameter is present in the Tuple object otherwise, returns false.
5. Java Tuple containsAll() Method
The containsAll() method is used to check the presence of a collection of values in a Tuple. It can be used with any Tuple class object.
Syntax:
Parameters:
- X: Data type of values
- value1, value2, ..., valueN: The variable number of values passed as an argument
- values: Array of data type X
Return Value: Returns true if the values are present. Otherwise, returns false.
6. Java Tuple equals() Method
equals() method is used for checking if a Tuple is equal to another Tuple given as a parameter. It can be used with any tuple subclass.
Syntax:
Parameters:
- TupleObj1: Tuple class object, eg: Unit, Pair, etc
- TupleObj2: Another Tuple object to be checked if it is equal
Return Value: Returns true if both Tuple objects are equal otherwise, returns false.
7. Java Tuple fromCollection() Method
The fromCollection() method is used to instantiate a Tuple object in an elegant way in terms of semantics. A collection of values is given as parameters. It is a static method.
Syntax:
Parameters:
- X: Data of values in the collection
- collection: Collection of values from which the Tuple class object is to be prepared
- TupleSubclass: It can be Unit, Pair, etc
Return value: The appropriate Java Tuple Subclass object with the values present in the collection.
8. Java Tuple fromIterable() Method
The fromIterable() method is used to instantiate a Tuple object in an elegant way in terms of semantics. The values of iterable are given as parameters. It is a static method.
Syntax:
Parameters:
- X: Data type of values in iterable
- iterable: The iterable of values from which the tuple object is to be constructed
Return Value: An appropriate Tuple subclass object
9. Java Tuple getSize() Method
The getSize() method is used to get the size (number of values) of the tuple object. It can be used with any tuple object.
Syntax:
Return Value: Returns an integer value signifying the number of elements.
10. Java Tuple getLabel() Method
The getLabel() method is used to obtain the label from the LabelValue class object. It is used only with the LabelValue class object. The Label returned ensures tupe-safety.
Syntax:
Return Value: Returns a Label (element present at the index 0 of the LabelValue object) of the associated type.
11. Java Tuple getValue() Method
The getValue() method is used to obtain the value of the Tuple object present at the index passed as a parameter. It can be used any Tuple class object.
Syntax:
Parameters: pos: Index of the value to be fetched
Return Value: Returns an Object value that is present at the index pos.
12. Java Tuple getValueX() Method
getValueX() is used to obtain the value present at index X of the Tuple object. It can be used with any Tuple object. The value is of type of Xth element, i.e., it maintains the type-safety, unlike getValue(X).
Syntax:
Parameters:
- A: Type of Xth element
- X: Index of value to be fetched, the value of X lies between 0 to TupleObjSize - 1.
Return Value: Returns value present at Xth index, with its associated type, of the Tuple object.
13. Java Tuple getKey() Method
getKey() method is used to get the key from the KeyValue class object. It can only be used with KeyValue class objects. It ensures the type-safety of the returned key.
Syntax:
Return Value: Returns a key present at the index 0 of the KeyValue class object.
14. Java Tuple setKey() Method
setKey() method is used to set the key of KeyValue class object and can only be used with it.
Syntax:
Return Value: Returns another KeyValue class object with a key as the element passed in the parameter. The value is taken from the previous KeyValue class object.
15. Java Tuple setLabel() Method
setLabel() method is used to set the label of the LabelValue class object and can only be used with it.
Syntax:
Return Value: Returns another LabelValue class object with a label as the element passed in the parameter. The value is taken from the previous LabelValue object.
16. Java Tuple setValue() Method
setValue() is used to set the value of the LabelValue or KeyValue object and can only be used with them.
Syntax:
Return Value: Returns a new LabelValue or KeyValue object with a specified value but the previous key.
17. Java Tuple setAtX() Method
The setAtX() method is used to modify the value in the existing tuple present at index X. A new tuple is returned due to immutability.
Syntax:
Return Value: A new Tuple object with a modified value at the Xth index.
18. Java Tuple removeFromX() Method
removeFromX() is used to remove a value (at Xth index) from an existing tuple. The tuple size decreases from n to n-1, i.e., a new tuple with one value less is obtained.
Syntax:
Return Value: Returns a new Tuple object with a size one less than the existing tuple by removing the element at index X.
19. Java Tuple indexOf() Method
The indexOf() method is inherited from the Tuple class and is used to find the index for a value passed in the parameter. It can check for all types of values.
Syntax:
Return Value: Returns an integer which is the first index of the value specified, if found otherwise, returns -1.
20. Java Tuple lastIndexOf() Method
The lastIndexOf() method is also inherited from the Tuple class and is used to find the last index of the value passed in the parameter. It can check for all types of values.
Syntax:
Return Value: Returns ans integer which is the last index of the value specified, if found otherwise, returns -1.
21. Java Tuple toArray() method
The toArray() method, inherited from the Tuple class, converts the values in the Tuple object into an Object type array. It can be used with any Tuple object.
Syntax:
Return Value: Returns an array of an Object type that contains the tuple values.
22. Java Tuple toString() Method
The toString() method, inherited from the Tuple class, converts the Tuple object values into a string.
Syntax:
Return Value: Returns string value that contains comma-separated tuple object values.
23. Java Tuple hashCode() Method
The hashCode() method gives the hash code for the Tuple object. It is always the same, given the object doesn't change. JVM generates a unique Hash code at object creation time. It is used to perform operations within hashing algorithms and data structures like Hashtable, HashMap, etc. Unique code also enables searching the object.
Syntax:
Return Value: Returns an integer value representing the hash code value for the tuple object.
Implementation of Tuple in Java
org.javatuples provides a simplified way of creating a tuple. You can create a tuple object corresponding to the desired size.
Example:
Output:
Explanation: The above example shows how the tuples are implemented in Java. Here, we create a Quartet tuple using the constructor of the corresponding class to store a Car's details. On printing to the console, comma-separated values are printed enclosed inside [].
Java Tuple Operations
The next sections discuss the various operations supported by Tuple in Java using examples.
a. Creating a Tuple
There are three different ways to create a tuple:
By Using the with() Method
Syntax:
Example:
Output:
By Using Constructor
Syntax:
Example:
Output:
By Using Collection
The javatuples library supports two ways to create a tuple from the collection, either by using the fromCollection() method or the fromArray() method. You may use any of them depending on the requirement. Please note that the number of items in the collection should match the type of tuple that you want to create.
Syntax:
Example:
Output:
b. Getting Values
There are two ways to fetch the values from a tuple:
i) Java Tuple getValueX() method
Every Java Tuple class has a getValueX() method for fetching the values from tuples. X specifies the order of the element inside the tuple. It has a value like an index of an array.
Example:
Output:
Explanation: The above example uses getValueX() methods of Triplet object. These methods are type-safe and hence, no casting is required. We directly store the values present at 0th, 1st, and 2nd places into variables of suitable types.
ii) Java getValue(int index) Method
This method is an alternative to the previous method. It accepts a zero-based position of the element to be fetched. But the drawback with this method is that it is not type-safe and explicit casting is required.
Example:
Output:
Explanation: As shown in this example, when we use the getValue(int index) method we are not ensured of the type-safety. So we need to explicitly cast each value to its proper type.
c. Setting Values using Java Tuple setAtX() Methods
Once created, Java tuples are non-modifiable as they are immutable. To overcome this problem at a certain level, the library provides another class of methods, commonly denoted as setAtX() methods. This method will create a copy of the existing but with newly added value at the given index.
Example:
Outptut:
Explanation: In the above example, we use a setAt1(val) method of Pair to use an existing pair tuple object but modify the 1st index of the previous tuple and return a new tuple with values 1, 5. This operation does not modify the original tuple because they are immutable.
d. Adding Elements
You can easily add new elements to the tuples but this will return a new tuple of one higher-order due to their immutability. The two ways to add elements are described below:
i) At the end of the Tuple using the Java Tuple add() method
Example:
Output:
Explanation: This example also shows the immutable nature of tuple in Java. On adding a new element, a new tuple of higher order is created, i.e., on adding a new element to Pair we get a Triplet in return. We can also add one tuple to another as it is shown above.
ii) At Specific Index using Java Tuple addAtX() method
It is a useful method to add new elements to an existing tuple at a specified position given by X, where X is a zero-based position.
Example:
Output:
Explanation: The addAt1() method used here inserts a new string at index 1 and shifts all other elements to their right. After the operation, a new Java tuple is returned which consists of four elements (Quartet).
e. Removing Elements
To remove elements from a tuple you can use the removeFromX() method. X is the same as discussed above.
Example:
Output:
Explanation: We create a Triplet of strings to demonstrate the remove operations at different indices. The first call to the removeFrom0() method removes the string present at index 0 which is "First" and pPair is obtained with two strings. The second call to removeFrom1() removes the string at index 1 and again another Pair is obtained, this time different from the previous one.
f. Iterate over Tuple
The Java Tuple can be iterated upon (similar to Collections or Arrays) because they implement the Iterable interface.
Example:
Output:
Explanation: The for-each loop is used here in a similar way to how it is used for Collections or Arrays, except for the fact that every time the type of value changes. So we use a variable of Object type to hold the value in the current iteration.
h. Searching in Tuple
i) Java tuples contains() and containsAll() Methods
- The contains() method checks for the presence of an element in the tuple and returns a boolean value.
Example:
Output:
Explanation: The contains() method returns true or false depending upon the presence of value in the Triplet tuple. The "a" and "c" are present, therefore it returns true, except for the "d" as it is not available in the Tuple.
- The containsAll() method checks whether all elements of a collection are present in the tuple or not.
Example:
Output:
Explanation: The Triplet created in the above example contains three integers (1, 2, and 3). After checking with the first list, the method returns false because 1 and 2 are present but 4 is absent. The check with the second list returns true because all the elements of list2 are present in the Triplet tuple.
ii) Java tuples indexOf() and lastIndexOf() Methods
These methods are used to return the first index and last index of a specified element in the tuple, respectively.
Example:
Output:
More Examples of Java Tuple
Converting Tuples to Arrays or Lists
The toArray() & toList() methods of the Java Tuple class can be used to convert them into Array & List, respectively. The methods return an Array or a List of Object type even though all elements of Tuple may contain elements of the same data type.
Output:
Explanation: Notice in the example that even though elements of the same data type are present but still an Object type Array and List are returned.
Tuple Vs. List/Array
Tuple | List |
---|---|
It is a set of comma-separated values enclosed inside parantheses | It is a set of comma-separated values enclosed inside square brackets |
Optional to include Parentheses | Mandatory to include Square brackets |
Immutable in nature | Mutable in nature |
Less memory required | More memory required |
Less number of factory methods | More factory methods available |
Has a fixed length | Has a variable length |
It can store heterogeneous data | It can store homogeneous data |
Faster as compared to lists | Slower as compared to tuples. |
Represented as t = (1, 2, 3, 4, 5) | Represented as l = [1, 2, 3, 4, 5] |
Conclusion
- Java Tuple is a data structure that can store heterogeneous data which should be assigned data only once. The data may or may not be related to each other.
- Tuples are immutable, type-safe, iterable, comparable and serializable.
- Java doesn't support tuples by default. Therefore, a 3rd-party library javatuples is used.
- Tuple is an abstract class and it has ten most common Java Tuple subclasses whose sizes range from 1 (Unit) to 10 (Decade). Apart from these, the two more subclasses are KeyValue and LabelValue.
- There are mainly two ways to create a tuple: using a constructor or through a more convenient static factory method with(). They can also be created using a collection directly via the fromArray() or fromCollection() method.
- Every modifying operation on a Java Tuple class does not actually modify the original tuple but returns a newly created one because they are immutable.
- Java Tuple class offers two get-value methods: the getValueX() method (type-safe) and the getValue(int index) method (not type-safe and explicit casting required).
- Setting values in Java Tuples is done through a setAtX() method, and X is the index.
- New elements can be added using add() and addAtX().
- Tuples in Java also offers you to remove the elements at certains positions using the removeAtX() method.
- The tuples can also be iterated in the same manner as Collections or Arrays are iterated.
- The searching methods of Java tuples are contains(), containsAll(), indexOf(), lastIndexOf().
- Tuples are introduced in order to overcome the design issues with Arrays and Collections, i.e., a tuple in Java allows heterogeneous data to be stored which is not possible in a standard way with Arrays & Collections.