java.util.Date Class
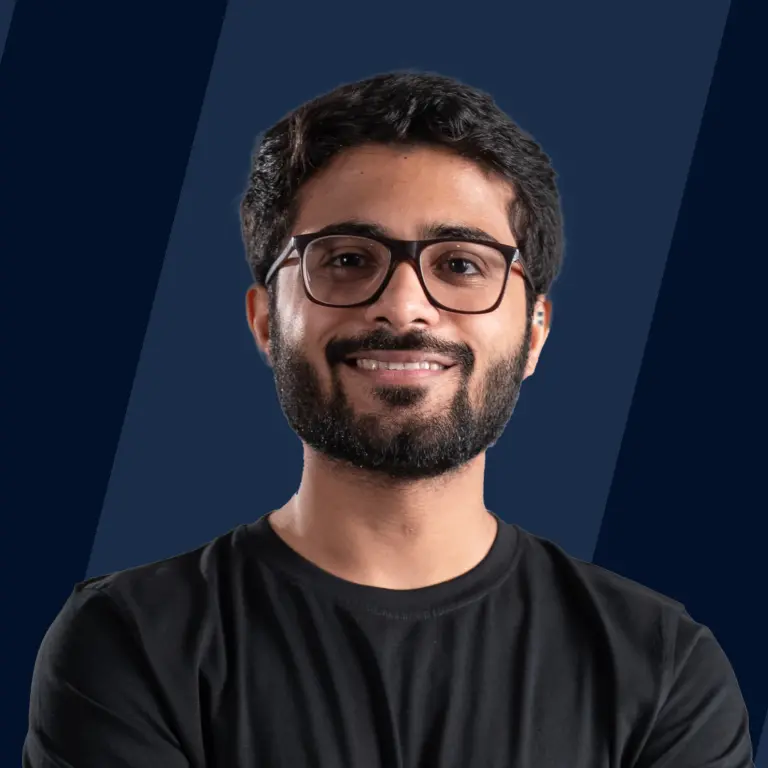
Overview
To represent date and time in Java, we use the java util date class. The java. util. The Date class is a class in java. util package. It contains methods and constructors to add dates and times in our code. The java util Date class is used to represent a particular moment in time, with millisecond precision since the
00:00:00 GMT (the epoch time).
Introduction to java. util.Date
While writing a certain program, we might have to include the current date or time or a specific instant in time with millisecond precision. A Date class is provided to incorporate this into our Java program. The java.util.Date is a class that is inside java. util package. The java util date class provides us with many methods and constructors to include date and time. We need to import the java.util.date class to include its methods in our code.
The Java util date class represents a particular moment in time with millisecond precision elapsed since 1st January 1970 00:00:00 GMT.
Declaration
The most common way to declare the java util date class is as follows-
We have used the Date() constructor which is the most basic constructor of java. util.Date class. We will dive deep into different constructors in the upcoming section.
Constructors of java. util.Date
The java. util. Date constructors have primarily two constructors. There are a few more constructors but they are now deprecated and were before Java 8.
No. | Constructor | Description |
---|---|---|
1. | Date() | It is used to create a date object to represent the current date and time. |
2. | Date(long milliseconds) | It is used to create a date object for the given milliseconds since January 1, 1970, 00:00:00 GMT. |
Constructors Detail
Now that we know the constructors along with their usage, let us look at the examples.
Examples:-
1) Creating a date object using Date()
This will create an object with the time set to the current time measured to the nearest millisecond and the date set to the current date.
2) Creating a date object using Date(long milliseconds)
Methods of java. util.Date
As you must be aware that all the classes in Java are derived from the Object class in Java which is the base class for all the classes. So the methods in the Object class are inherited by the Date class. The Date class has its distinct methods as well. We will discuss them in depth.
No. | Method | Description |
---|---|---|
1. | boolean after(Date date) | This method is used to return if the current date is after the given date. |
2. | boolean before(Date date) | This method is used to return if the current date is before the given date. |
3. | int compareTo(Date date) | This method is used to compare the current date with the given date. |
4. | boolean equals(Date date) | This method is used to check whether two dates are equal or not based on their millisecond difference. |
5. | long getTime() | This method is used to return the time represented by this date object. |
6. | void setTime(long time) | This method is used to set the current date and time to the given date and time. |
7. | String toString() | This method is used to convert this date object to a String-type object. |
8. | int hashCode() | This method is used to generate a hash code for the given object. |
9. | Object clone() | This method is used to generate a clone for the invoking object. |
Methods Detail
Now that we have seen the various methods in the Java util Date class, let us take a look at its syntax.
1) Boolean After(Date date)
This method is used to return if the current date is after the given date. It returns true if the current date is after the given date.
Syntax
2) Boolean Before(Date date)
This method is used to return if the current date is before the given date. It returns true if the current date is before the given date.
Syntax
3) int compareTo(Date date)
This method is used to compare the current date with the given date. It returns either 1, 0, or -1 based on the comparison made.
Syntax
The result can be: 0: if the argument date is equal to the given date. -1: if the argument date is greater than the given date. 1: if the argument date is less than the given date.
4) boolean equals(Date date)
This method is used to check whether two dates are equal or not based on their millisecond difference. It returns true if the given dates are equal.
Syntax
5) long getTime()
This method is used to return the time represented by this date object. It returns the number of milliseconds from 1st January 1970 till the current date.
Syntax
6) void setTime(long time)
This method is used to set the current date and time to the given date and time. It sets the date object to represent time in milliseconds after January 1, 1970, 00:00:00 GMT.
Syntax
7) String toString()
This method is used to convert this date object to a String-type object.
Syntax
The date object is converted into a string. The resultant string is in the following format-
day: day of the week month: month dd: day of the month hh: hour mm: minute ss: second zz: time zone yyyy: year
8) int hashCode()
This method is used to generate a hash code for the given object.
Syntax
9) Object clone()
This method is used to generate a clone for the invoking object. This method overrides the clone method in the Object class.
Syntax
java. util.Date Examples
Let us take a look at a few examples.
Example 1
In this example, we will use the setTime() and toString() methods of the Java util date class.
Output
First, we imported the Date class to use the methods and constructors provided in them. Then we created a date object whose time and date are the current time and date. Then we used the setTime() method where we set the time. The final date object is now converted into a string and displayed to the user.
Example 2
In this example, we have used the clone() method, after() method, and before() method to check for various possibilities.
Output
The above code displays the clone(), after() and the before() method. We have created 2 dates and made a comparison between these dates. We first checked if the date2 is after the date1 and then printed accordingly. Then we cloned date1, converted and displayed it in the form of a string. Then we checked if the date2 is before the date1. The output is printed accordingly.
Example 3
In this example, we will be dealing with the equals() method, compareTo() method, and getTime() method.
Output
Here we have compared two dates using the compareTo() method. As we have seen in the previous section about the return values of the comparison. Then we checked whether the date is equally using the equals() method. Then we returned the time in milliseconds using the getTime() method.
java. util.Date vs java.sql.Date
- In Java, we have another class known as java.sql.Date. It is a class that extends java. util.Date class.
- It is used to represent SQL DATE, which keeps years, months, and days. No time data is kept.
- Here the date is stored as milliseconds since 1st January 1970 00:00:00 GMT.
- The java.sql.Date class should only be used while dealing with databases.
- It does not hold information about the timezone.
Conclusion
- To represent date and time in Java, we use the java util date class.
- The java util date class provides us with many methods and constructors to include date and time.
- The java. util. The date is a class that is inside java. util package. We need to import the java. util.date class to include its methods in our code.
- The class is used to a particular moment in time with millisecond precision elapsed since 1st January 1970 00:00:00 GMT.
- various methods can be used to deal with date and time in Java.
- There is another class java.sql.Date. It is a class that extends java.util.Date class. It is only used while dealing with databases.