Java.lang.Object.wait() Method
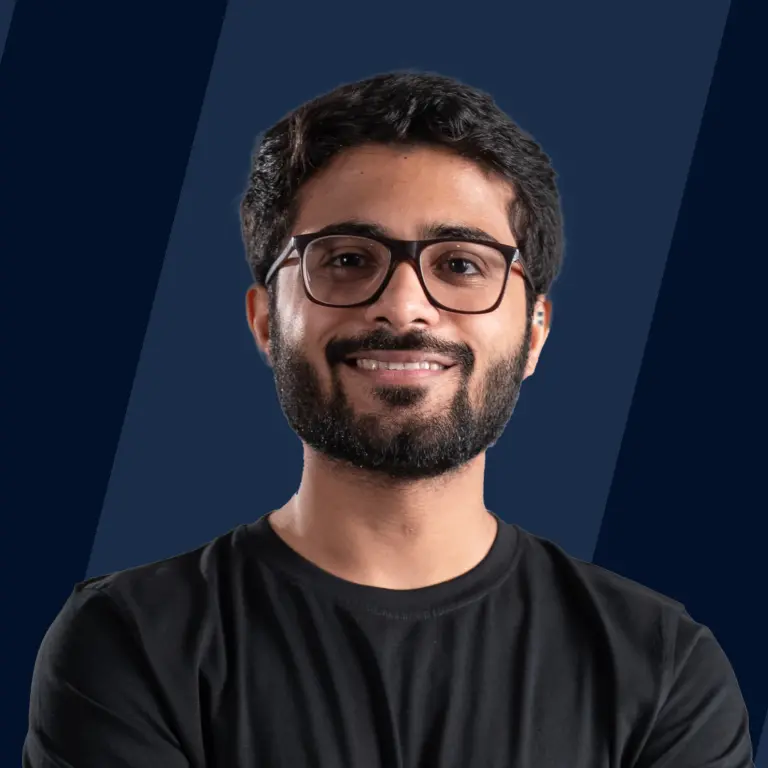
In Java, the wait() method is used to pause the execution of a thread until another thread signals that it can resume. When a thread calls wait() on an object, it releases the lock on the object and waits until another thread calls notify() or notifyAll() on the same object.
Syntax
InterruptedException is thrown when a thread that is waiting on an object using the wait() method is interrupted by another thread.
Parameters
The wait() method has three variants:
- wait(): This method waits indefinitely until another thread calls notify() or notifyAll() on the same object.
- wait(long timeout): This method waits for a specified period of time until another thread calls notify() or notifyAll() on the same object, or until the specified timeout period has elapsed.
- wait(long timeout, int nanos): This method is similar to the previous variant, but it allows for more precise timing by specifying a number of nanoseconds in addition to the timeout period.
Return Value
The wait() method has no return value.
Exceptions in wait() method
Exceptions allowed in wait() method include:
- IllegalArgumentException- This is the exception thrown when the value of the arguments is not in the acceptable range (negative or out of bounds).
- IllegalMonitorStateException- The exception thrown if the thread being executed does not have permission to monitor the object's state.
- InterruptedException- The exception is thrown when another thread interrupts the execution of the current thread. Once this exception is thrown, the interruption clears.
Example of Java Wait()
Explanation:
- In this example, the SharedObject class represents an object that can be in a "ready" or "not ready" state.
- The waitForReady() method is used to wait for the object to become ready, while the setReady() method is used to signal that the object is ready.
- The WaitingThread class represents a thread that waits for the shared object to become ready by calling the waitForReady() method on the shared object.
- The main() method creates a new instance of SharedObject and a new instance of WaitingThread, starts the waiting thread, and then signals that the shared object is ready by calling the setReady() method.
- When the waiting thread calls the waitForReady() method, it enters a wait state until the shared object is ready.
- Once the setReady() method is called, the waiting thread is notified and can continue execution.
What is Java wait() Method?
To sum it up, the wait() method causes the current thread under execution to wait either until another thread invokes the notify() or notifyAll() method for the given object, or a specified amount of time has elapsed.
More Examples of Java wait()
Output:
Explanation:
- The program above demonstrates the use of the wait() and notify() methods to coordinate two threads accessing a shared resource.
- The shared resource in this case is the paper variable, which represents the number of sheets of paper available for printing.
- The PaperPrint class has two methods, print() and reprint(). The print() method takes an integer argument representing the number of sheets to print.
- It uses a for loop to iterate over the pages to be printed, and if the paper variable reaches 0 (meaning there is no paper available), it invokes the wait() method on the object's monitor.
- The wait() method causes the current thread (in this case, the thread executing the print() method) to wait until another thread calls notify() on the same object.
- In this program, the reprint() method is responsible for reloading the printer and resuming the printing process. It uses the notify() method to wake up the waiting thread.
- The Main class creates two new threads, one to invoke the print() method and one to invoke the reprint() method.
- When the print() method is invoked, it will print 60 sheets of paper, but since the initial value of paper is 40, it will wait for the reprint() method to be invoked to reload the printer with more paper.
- Once the reprint() method is invoked and reloads the printer with 40 more sheets of paper, it calls the notify() method to wake up the waiting thread, which can then continue the printing process until all 60 pages have been printed.
Difference Between wait() and sleep() in Java
wait() | sleep() |
---|---|
The wait() method is a member of the Object class. | The sleep() method belongs to the Thread class. |
The wait() method is a dynamic method | The sleep() method is a static method. |
The wait() method can be called only from a synchronized context and releases lock during synchronization only. | The sleep() method doesn't need to be called from a synchronized context and it does not release the lock on the object during synchronization. |
The wait() method has three overloaded methods. | The sleep() method has only two overloaded methods. |
Conclusion
- The Java wait() method is used to pause the execution of a thread until another thread signals that it can resume.
- When a thread calls wait() on an object, it releases the lock on the object and waits until another thread calls notify() or notifyAll() on the same object.
- The wait() method has three variants and can throw exceptions like IllegalArgumentException, IllegalMonitorStateException, and InterruptedException.