Using Java For Web Development (for Beginners)
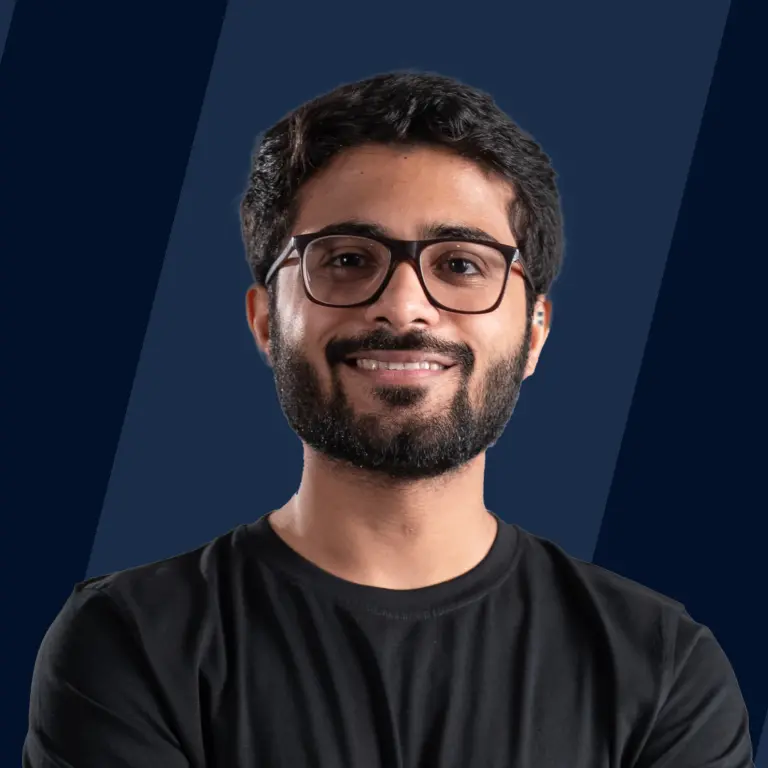
Overview
Using Java for web development is a popular and powerful approach in the world of software development. Java, known for its portability, scalability, and robustness, has evolved to become a versatile language for building web applications. Developers can leverage various Java-based technologies, such as Servlets, JSP (JavaServer Pages), Spring Framework, and Java EE (Enterprise Edition), to create dynamic and feature-rich web applications.
Java Web Development
Java web development encompasses both front-end and back-end development, offering a robust ecosystem of tools, frameworks, and libraries to build scalable and feature-rich web applications.
Front-End Development:
In Java web development, front-end development often includes the following key components:
- HTML/CSS
- JavaScript
- Front-End Frameworks: Java developers can utilize front-end frameworks like React, Angular, or Vue.js.
Back-End Development:
In Java web development, the back end typically consists of the following components:
- Servlets and JSP
- Frameworks: Developers often use frameworks like Spring, Spring Boot, and Java EE to streamline back-end development.
- Database Connectivity: Java supports various database technologies, and developers use JDBC (Java Database Connectivity) or Object-Relational Mapping (ORM) frameworks like Hibernate to interact with databases, store and retrieve data.
- Security: Java web applications prioritize security. Developers implement authentication, authorization, and other security features to protect user data and the application itself.
- APIs and Web Services: Java is well-suited for creating RESTful APIs and web services using frameworks like Spring REST and JAX-RS, enabling communication between the front end and back end or integration with other systems.
Java Server Pages
JavaServer Pages (JSP) is a technology used for developing dynamic web applications in Java. It allows developers to embed Java code within HTML pages, which are then processed by a JSP container or server. JSP simplifies the creation of web pages that can generate dynamic content by enabling the use of Java code to interact with data, perform calculations, and generate HTML dynamically.
Implementation:
Here are the basic steps for implementing JSP:
- Set up a Web Application Project: Create a web application project using a Java IDE like Eclipse or IntelliJ IDEA, or by manually setting up a directory structure.
- Create JSP Files: Create JSP files with a .jsp extension within your web application project. These files will contain a mix of HTML and Java code.
- Write Java Code: You can embed Java code within JSP using <% %> tags. For example, you can declare variables, define methods, and execute Java code to generate dynamic content.
- Use JSP Tags: JSP provides custom tags, known as JSP tags or JSTL (JSP Standard Tag Library) tags, which simplify tasks like database access, looping, conditional statements, and more.
- Deploy and Run: Deploy your web application to the web server and access the JSP pages through a web browser. The server will compile JSP pages into servlets and execute them to generate dynamic HTML content.
Example:
Here's a simple example of a JSP page that displays a Hello, World! message using JSP code and tags:
In this example, <%= new java.util.Date() %> is an example of embedding Java code to display the current date and time.
JSP Tags:
JSP tags are categorized into standard actions and custom actions. Some commonly used JSP tags include:
- Core Tags (JSTL): These tags provide basic control structures, iteration, and conditional logic. Examples include <c:forEach>, <c:if>, and <c:choose>.
- Custom Tags: You can create your custom tags to encapsulate reusable functionality in JSP.
- Expression Language (EL): EL is used to access JavaBean properties and perform simple operations in JSP. You can use ${} syntax to access variables and properties.
- Scriptlet Tags: <% %> tags allow you to embed Java code directly into your JSP pages.
- Action Tags: These tags perform specific actions like forwarding, including other resources, and error handling. Examples include <jsp:include>, <jsp:forward>, and <jsp:useBean>.
Servlets
Servlets are Java-based server-side components that handle requests from clients (typically web browsers) and generate dynamic web content. They are a fundamental part of Java web development and provide a way to create dynamic web applications. Here are some common methods in servlets, followed by a brief overview of servlet implementation and an example:
Common Methods in Servlets:
- getParameter(String paramName): This method is used to retrieve the value of a request parameter sent by the client. It takes the parameter's name as an argument and returns its value as a string. For example:
- getRequestDispatcher(String path): This method is used to obtain a request dispatcher for a given resource (usually a servlet or JSP page). It is used for including or forwarding requests to other resources. For example:
- forward(request, response): This method is used in combination with getRequestDispatcher() to forward a request to another resource (servlet or JSP). It transfers control from one servlet to another. For example:
Implementation:
To implement a servlet, follow these steps:
Create a Java Class: Create a Java class that extends javax.servlet.http.HttpServlet and override the doGet() or doPost() method to handle client requests. The servlet class should be part of a Java web application project.
Configure Servlet: Configure the servlet in the web application's deployment descriptor (usually web.xml) to specify its URL mapping and other parameters.
Implement Servlet Logic: Write the servlet logic within the doGet() or doPost() method. This is where you process incoming requests and generate responses.
Compile and Deploy: Compile the servlet class and package it in a WAR (Web Application Archive) file along with other necessary resources. Deploy the WAR file to a servlet container like Apache Tomcat.
Setting up CI/CD pipelines: Setting up a Continuous Integration/Continuous Deployment (CI/CD) pipeline for Java web applications involves automating the process of building, testing, and deploying your application. This ensures that code changes are consistently and reliably delivered to production. Here's are some tools and prectices to set up a CI/CD pipeline for a Java web application:
- Use a version control system like Git to manage your Java web application's source code. Popular platforms include GitHub, GitLab, and Bitbucket.
- Choose a build tool like Apache Maven or Gradle to manage dependencies and build your Java application. Define a pom.xml (for Maven) or build.gradle (for Gradle) file to specify project settings, dependencies, and build instructions.
Example:
Here's a simple servlet example that retrieves a parameter from a client request and sends a response:
Explanation:
In this example, the servlet retrieves a "name" parameter from the client's request and responds with a personalized greeting. You can access this servlet by sending a GET request with the "name" parameter to its URL.
To deploy and access this servlet, you need to configure it in the web.xml file of your web application and deploy the application to a servlet container. Then, you can access it using a URL like http://localhost:8080/your-app-name/hello?name=John.
Java Database
Java offers several mechanisms and technologies for working with databases. One of the most commonly used approaches is Java Database Connectivity (JDBC), which provides a standard Java API for connecting to and interacting with relational databases. Here's an overview of Java database access using JDBC:
JDBC
JDBC (Java Database Connectivity) is a Java API that provides a standard way to interact with relational databases. It allows Java applications to connect to databases, execute SQL queries, and retrieve or update data. Below, you'll find an overview of JDBC, an example of its implementation, and two commonly used methods: createStatement() and executeQuery().
Implementation
To use JDBC in a Java application, follow these general steps:
- Load the JDBC Driver: Before you can connect to a database, you need to load the appropriate JDBC driver. Different databases have their own JDBC drivers, and you must include the driver's JAR file in your project's classpath.
- Establish a Connection: Use the JDBC driver manager to create a database connection. You typically provide a JDBC URL, username, and password for authentication.
- Create a Statement: Once you have a connection, you can create a statement object. This statement is used to execute SQL queries against the database.
- Execute SQL Queries: Use the statement to execute SQL queries such as SELECT, INSERT, UPDATE, or DELETE. The executeQuery() method is used for SELECT queries, while executeUpdate() is used for other types of queries.
- Process Results: For SELECT queries, you can retrieve the results from the ResultSet object returned by executeQuery(). You can iterate through the ResultSet and access the data.
- Close Resources: It's important to close all resources (e.g., ResultSet, Statement, Connection) properly to release them and avoid memory leaks.
Example:
Here's a simple example of using JDBC to connect to a MySQL database, execute a SELECT query, and retrieve results:
In this example, we load the MySQL JDBC driver, establish a connection to a MySQL database, create a statement, execute a SELECT query, and process the results.
Common Methods of JDBC
- createStatement(): This method is used to create a Statement object that allows you to execute SQL queries. It returns an instance of the Statement interface.
- executeQuery(String sql): This method is used to execute a SQL query that returns a ResultSet. You provide the SQL query as a string parameter, and it returns the results of the query as a ResultSet object.
These methods are fundamental for interacting with databases using JDBC. createStatement() is used to create a statement object, and executeQuery() is used to execute a SELECT query and retrieve results in the form of a ResultSet.
Serve
Serve in web serving refers to the act of providing web content or services to clients, typically web browsers or other web-based applications. Web serving is a fundamental aspect of web development, and it involves responding to client requests for web resources like HTML pages, images, stylesheets, JavaScript files, and more.
Scalability and Performance:
Scaling Java web applications to handle increased traffic and maintain performance requires a combination of strategies, including load balancing, caching, and optimizing database queries. Here are some performance tuning techniques:
- Load Balancing: Load balancing distributes incoming traffic across multiple application servers to prevent any single server from becoming a bottleneck.
- Caching: Caching stores frequently accessed data in memory to reduce the need to fetch it from the database or generate it dynamically.
- Optimizing Database Queries: Efficient database queries are crucial for maintaining application performance.
- Code Profiling: Use profiling tools to identify performance bottlenecks in your code. Profiling helps pinpoint areas where optimization efforts will have the most impact.
FAQs
Q.What is a web server?
A. A web server is software or hardware that receives HTTP requests from clients (e.g., web browsers) and serves web content in response.
Q.How do web servers handle static files?
A. Web servers serve static files like HTML, CSS, and images directly from the file system without any processing.
Q.What is the difference between a web server and an application server?
A. A web server handles HTTP requests and serves static files, while an application server runs server-side code and generates dynamic content.
Q.What is load balancing in web serving?
A. Load balancing involves distributing incoming web requests across multiple servers to ensure scalability and even resource utilization.
Conclusion
- Java is a versatile programming language used for creating dynamic and interactive web applications.
- Servlets and JavaServer Pages (JSP) are core technologies in Java for web development. Servlets handle HTTP requests and responses, while JSP allows for embedding Java code within HTML pages.
- Java Database Connectivity (JDBC) is used to interact with databases, allowing web applications to store and retrieve data.
- Java has a variety of frameworks and libraries, including Spring, JavaServer Faces (JSF), and Hibernate, which simplify web application development.
- Java web applications often follow the Model-View-Controller (MVC) architecture to separate concerns and create maintainable code.