How to Scroll to an Element with Javascript?
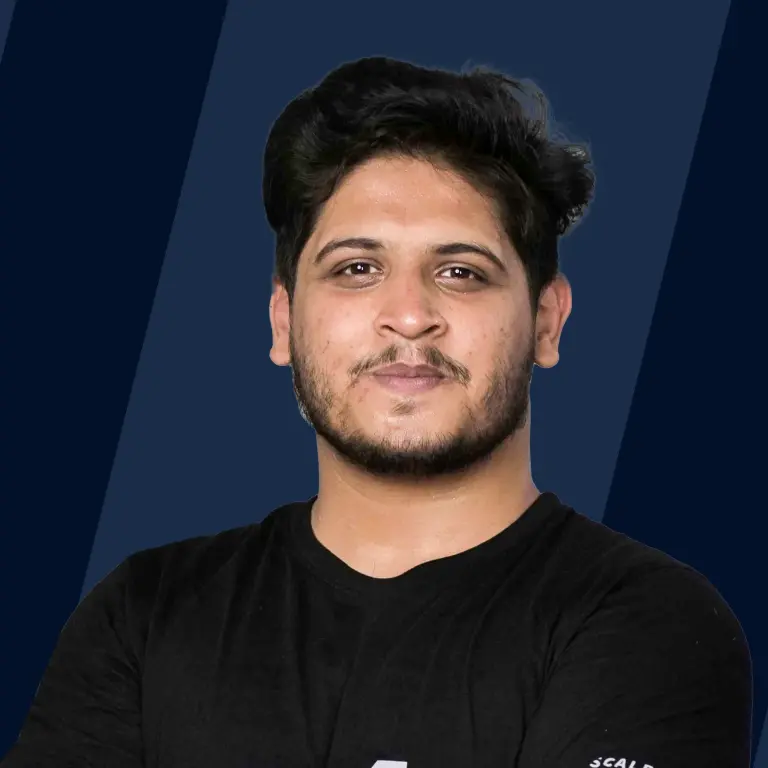
Overview
Scrolling to a specific element or part of a webpage is mainly done to keep a user intact with the webpage for a longer period. A scroll is a function that can be used also to scroll to the beginning of a webpage from the bottom of the webpage instantly. Instant scrolling functionality is done or implemented using JavaScript. Scrolling to a particular section of a webpage adds a single-click functionality without much intervention from the user.
There are different JavaScript scroll to element methods that are used to implement the functionality of scrolling to a particular element. These methods include:
- JavaScript scrollIntoView method.
- JavaScript scroll method.
- JavsScript scrollTo method. In this article, we will understand them thoroughly.
Introduction
Before learning about the JavaScript scroll to element, Let's learn a bit about JavaScript.
JavaScript is an object-oriented programming language used on web pages. JavaScript is the web programming language used on both the client and server sides to provide dynamic and interactive behavior in a webpage. It is a lightweight and easy-to-understand programming language. JavaScript is the most popular programming language in the world. It is a scripting language of a webpage that can be used even by non-browser environments like NodeJs, Adobe Acrobat, etc. Languages like HTML and CSS are used to provide structure and styling to a webpage respectively whereas JavaScript engages users by providing interactiveness to a webpage.
Now, let's learn about the Scroll function and the JavaScript scroll to element methods that implement the Scroll function.
Scrolling refers to functionality that moves the user from a specific part of the webpage to another. The scrolling in a website can be done by the physical components such as a scroll bar or with the help of buttons which are solely made to scroll in a specific element.
Scrolling to a specific element or part of a webpage is done using methods available in Javascript scroll to element which are as follows:
- scrollIntoView() method
- scrollTo() method
- scroll() method
Now, we will discuss each of the above-mentioned methods used for scrolling with proper explanations and examples.
The ScrollIntoView Method
The scrollIntoView method in JavaScript is used to scroll to a specific element of a webpage in a web browser. Specifically, in the JavaScript scrollIntoView method, the scrolling is done based on the scrollable parent element. In general, most of the time the parent element will be the window of the web browser but in some of the scenarios, When the required scrollable element is placed within another scrollable element then the scrolling is done concerning the parent element rather than the window of the web browser's window. The scrollIntoView() method helps to carry out the scrolling of an element into the visible area of the browser window.
Now, let's see the syntax of scrollintoview method.
Syntax
Parameter
There are several customizations available for the scrollIntoView() method which is done by passing scrollIntoViewOptions as the parameter.
scrollIntoView method takes a parameter which is known as the scrollIntoViewOptions. The scrollIntoViewOptions object contains the following customization properties:
-
Behaviour :- This property of scrollIntoViewOptions tells about the scrolling behavior. Whether the scrolling is done using scrollintoview method should contain an animation or there should be a normal instant scrolling without any animation up to the specific element. This property contains the following values which can be passed with the parameter:
- smooth: This value specifies smooth scrolling that is the scrolling with animation.
- auto: This value specifies instant scrolling to the required element without any animation.
-
Inline :- This property of scrollIntoViewOptions tells about the alignment mainly horizontal alignment of the required element concerning the parent scrollable element when using the scrollintoview method. This property contains the following values which can be passed with the parameter:
- start: This value specifies that the required element is aligned to the left or start of the parent scrollable element.
- center: This value specifies that the required element is aligned to the center of the parent scrollable element.
- end: This value provides that the required element is aligned at the end or right of the parent scrollable element.
- nearest: This value specifies that the element should be aligned according to the situation. If the element is at the right or the end then the required elements will also be aligned at the end. Similarly, if the element is at the left or the starting then the required elements will also be aligned at the left.
-
Block :- This property of scrollIntoViewOptions tells about the vertical alignment of a required element concerning the parent scrollable element when using the scrollintoview method. This property contains the following values which can be passed with the parameter:
- start: This value specifies that the required element is aligned at the top or start of the parent scrollable element.
- center: This value specifies that the required element is aligned to the center of the parent scrollable element.
- end: This value specifies that the required element is aligned at the bottom of the parent scrollable element.
- nearest: This value specifies that the element should be aligned according to the situation. If the element is at the top then the required elements will also be aligned at the top. Similarly, if the element is at the bottom then the required elements will also be aligned at the bottom.
Now, let's take an example to understand the scrollIntoView() method with proper implementation and explanation.
Code:
Output:
Explanation:
In the above example, we have made a simple HTML page. On the HTML page, we have added an H1 heading tag inside which the method name is written. Inside the <body> tag we have created a button named Scroll with the onclick() event which calls the scrollFunction() method. The scrollFunction() method will get the required element using the document.getElementById() method and then scrollIntoView() is called to get the scroll functionality. Inside the <style> tag, we have written the CSS code where we have specified the dimensions and color.
Now, let's take another example by using the parameter of the method so that we can understand the working of scrollIntoView more clearly.
Code:
Output:
Explanation
The above program is almost the same program we discussed earlier in the section. Inside the <body> tag we have created a button named Scroll with the onclick() event which calls the scrollFunction() method. The scrollFunction() method will get the required element using the document.getElementById() method and then scrollIntoView() is called with a parameter behavior: "smooth" which makes sure that the scrolling should not only be instant scroll rather it should be with animation which is the smoothness of the scroll. Inside the <style> tag, we have written the CSS code where we have specified the dimensions and color.
The scroll Method
The scroll() or windows.scroll() method in JavaScript scroll to element is used to scroll to a specific element of a webpage in a web browser. It scrolls the element based on the coordinates of the values given in the document. Mainly windows.scroll() method is used to scroll to an image where the coordinates of an image are calculated using a function.
Syntax
In the above syntax, the x-coordinate indicates the x-coordinate, and the y-coordinate indicates the y-coordinate of the image.
Parameter
- x-coordinate is one of the parameters of the window.scroll() method which shows the pixel in the x-axis which is the horizontal axis.
- y-coordinate is one of the parameters of the window.scroll() method which shows the pixel in the y-axis which is the vertical axis.
The scroll method contains the following customization properties:
- top : This value specifies that the required element is aligned at the top.
- left : This value specifies that the required element is aligned at the left.
- behavior : This property of scroll tells about the scrolling behavior. Whether the scrolling should contain an animation (smooth is used) or there should be a normal instant scrolling (auto is used) without any animation up to the specific element.
Now, let's take an example to understand the scroll() method with proper implementation and explanation.
Code
Output
Explanation
In the above example, we have made a simple HTML page. On the HTML page, we have added an H1 heading tag inside which the method name is written. Inside the <style> tag, we have written the CSS code where we have specified the dimensions and color.
Inside the <body> tag we have created a button named Scroll with the onclick() event which calls the scrollFunction() method. Inside the scrollFunction method, we will invoke the windows.scroll() method which will have coordinates of the element. The coordinate of the element is calculated using the method findPosition() method.
Inside the findPosition() method we have taken an argument obj and we have initialized a variable named currentTop with 0. Then we applied the offsetparent property which gets access to the nearest parent element and returns it. After this, we have incremented the value of currentTop using the offsetTop property that returns the top position concerning the parent element.
The ScrollTo Method
The scrollTo method in JavaScript scroll to element is used to scroll to a specific element of a webpage in a web browser. The scrollTo method scrolls the elements to the given coordinates which will be passed as the parameter in the method. This method can also be used to get or scroll to the element concerning its associated id.
Syntax
In the above syntax, the x-coordinate indicates the x-coordinate, and the y-coordinate indicates the y-coordinate of the image.
Parameter
- x-coordinate is one of the parameters of the window.scroll() method which shows the pixel in the x-axis which is the horizontal axis.
- y-coordinate is one of the parameters of the window.scroll() method which shows the pixel in the y-axis which is the vertical axis.
The scrollTo method contains the following customization properties:
- top : This value specifies that the required element is aligned at the top.
- left : This value specifies that the required element is aligned at the left.
- behavior : This property of scrollTo tells about the scrolling behavior. Whether the scrolling should contain an animation (smooth value is used) or there should be a normal instant scrolling (auto value is used) without any animation up to the specific element.
Now, let's take an example to understand the scrollTo() method with proper implementation and explanation.
Code:
Output:
Explanation
In the above example, we have made a simple HTML page. On the HTML page, we have added an H1 heading tag inside which the method name is written. Inside the <style> tag, we have written the CSS code where we have specified the dimensions and color. Here, everything works in the same way as it works in the scroll() method.
Inside the <body> tag we have created a button named Scroll with the onclick() event which calls the scrollFunction() method. Inside the scrollFunction method, we will invoke the windows.scrollTo() method which will have coordinates of the element. The coordinate of the element is calculated using the method findPosition() method. The function works in the same way as discussed in the scroll() method example section.
Browser Support
The JavaScript scroll to element methods: scrollIntoView(), scrollTo(), and scroll() used for scrolling functionality are supported in almost all web browsers available.
Browser | Chrome | Firefox | Edge | Apple-Safari | Samsung Internet | Safari-on-iOS | Opera |
---|---|---|---|---|---|---|---|
Support | YES | YES | YES | YES | YES | YES | YES |
Some web browsers are available that do not support the parameter part of the scrollIntoView() method.
Browser | Apple-Safari | Safari-on-iOS |
---|---|---|
Support | NO | NO |
Conclusion
- Scrolling to a specific element or part of a webpage is mainly done to keep a user intact with the webpage for a longer period.
- The scrolling in a website can be done by the physical components such as a scroll bar or with the help of buttons which are solely made to scroll in a specific element.
- Scrolling to a specific element or part of a webpage is done using JavaScript scroll to element methods available in Javascript which are as follows:
- scrollIntoView() method
- scrollTo() method
- scroll() method
- In the scrollIntoView method, the scrolling is done based on the scrollable parent element.
- When we use the scrollintoview method the required scrollable element is placed within another scrollable element then the scrolling is done concerning the parent element.
- scroll() method scrolls the element based on the coordinates of the values given in the document.
- windows.scroll() method is mainly used to scroll to an image where the coordinates of an image are calculated using a function.
- The scrollTo method scrolls the elements to the given coordinates, which will pass as the method's parameter.
- The JavaScript scroll-to-element methods likescrollIntoView(), scrollTo(), and scroll() used for scrolling functionality are supported by almost all web browsers.