Sort Array Alphabetically in JavaScript
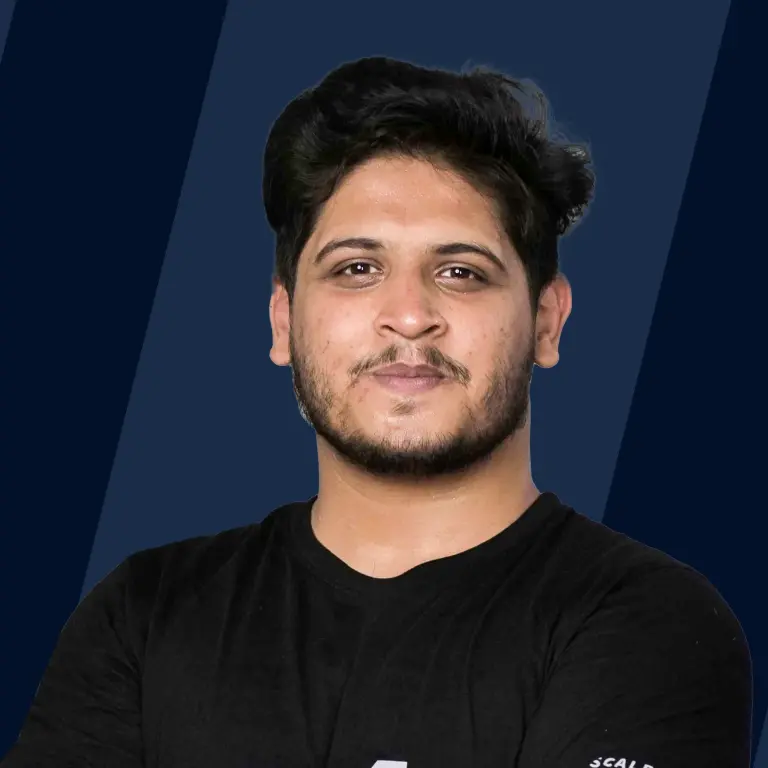
Overview
When you have a list of words, you might occasionally want to alphabetize each term (from a-z). Alternatively, you might wish to sort people according to their names and have an array of objects with user information, such as names. The sorting methods in javascript mean the swapping of the position of the elements of an array based on some criteria. These criteria are defined by the user. We can sort the characters of an element as well as we can sort various elements stored in an array.
Introduction
In JavaScript, there are various methods for sorting the different data types such as strings and integers. For example, an array of objects can be sorted using the sort() method and then retrieved in alphabetical order. It is possible thanks to the alphabet's index values. Every alphabet has its distinct index value. Any string is retrieved in either ascending or descending order based on these values.
The two strings are also compared using the localeCompare() function. The localCompare() method is used for comparing strings as well as alphabets and arranging them in ascending or descending order as per the user's choice.
JavaScript Program to Sort Array Alphabetically Using the sort() Method
The sort() method in javascript is used to sort the given array in place and then it returns the sorted array. The sort() method in javascript sorts the array in ascending order by default. The method works by the principle of UTF-16 code unit values. In this, the given elements of the array are first converted into strings, and after that their UTF-16 digit values are compared. After comparison, the elements are sorted in ascending order.
If we use the sort() method for sorting the integer values then there may be incorrect output in some cases. For example, let us we have to compare the integer values 46 with 125. The sort method will consider the value 46 bigger than 125 because 4 is greater than 1.
Syntax of the sort() Method in Javascript:
Let us see an example of sorting the array alphabetically using the sort() method.
Output:
Explanation: In the above example, first, we created an array namely city. Then we added four city names that are Kolkata, Delhi, Bangalore, and Mumbai as the elements of the array city. Then we created a new variable namely sortedArray to store the new sorted array. After that, we used the sort() method appended with city. Then we used the console.log to print the sorted array as the output.
JavaScript Program to Sort Array Alphabetically Using the localeCompare() Method
Before going to the topic of what is javascript localecompare() method is, let us have a brief discussion over what are the methods in javascript. The methods in javascript are defined as the set of code that is used to perform some specific tasks. All the methods have some definitions that help in performing the task. These methods store some property values. Now coming to the javascript localecompare() method.
The javascript localecompare() method in javascript is used for making the comparison of the given two strings. The method is a built-in method in javascript that is used for returning a number and that number indicates if the reference string is coming before, or after, or if it is the same as the given string in sort order. The method returns a negative, a positive, or a zero value according to the compared analysis. If the reference string comes before the compare string, it gives a negative and if the reference string comes after the compare string, it gives a positive number If the reference string comes equivalent to the compare string, it gives a zero (0).
Talking about the parameters and the arguments of the javascript localecompare() method. There are three parameters of the javascript localecompare() method. These parameters are compareString, options, and locale. The compareString refers to the string that we need to compare with the reference string. This parameter is a required parameter.
The options parameter is sued for customizing the behavior of the given function. This parameter allows the application to specify the language of which language formatting convention should we need to use. The locale is an optional parameter. It is an array of the BCP 47 language tag that is used for determining the sort order. This language defines a language that contains two things that are a primary language code and an extension.
Example 1. Let us see a short and simple example to understand the working of the localecompare() method.
Output:
Explanation: In the above example, we simply created a variable result1 and passed the reference string c to it. Then we passed b as the compare string. After that, we used the javascript localecompare() method for the comparison of the reference string to the compare string and assigned the return value to result1. Since the reference string c comes after the compare string b, the method returns 1 as the return value.
Example 2.
Output:
Explanation: In the above example, we have created an array namely Name_arr in which we store three names of the boy as the elements of that array. Then we used the function iteration over the objects by utilizing the localeCompare() method on the names. Then we used the console.log() method to print the sorted array as the output.
Conclusion
- In JavaScript, there are various methods for sorting the different data types such as strings and integers.
- The sort() method in javascript is used to sort the given array in place and then it returns the sorted array.
- The sort() method in javascript sorts the array in ascending order by default.
- The javascript localecompare() method in javascript is used for making the comparison of the given two strings.
- The method returns a negative, a positive, or a zero value according to the compared analysis.
- If the reference string comes before the compare string, it gives a negative, and if the reference string comes after the compare string.
- It gives a positive number if the reference string comes equivalent to the compare string, it gives a zero (0).