JavaScript appendchild() Method
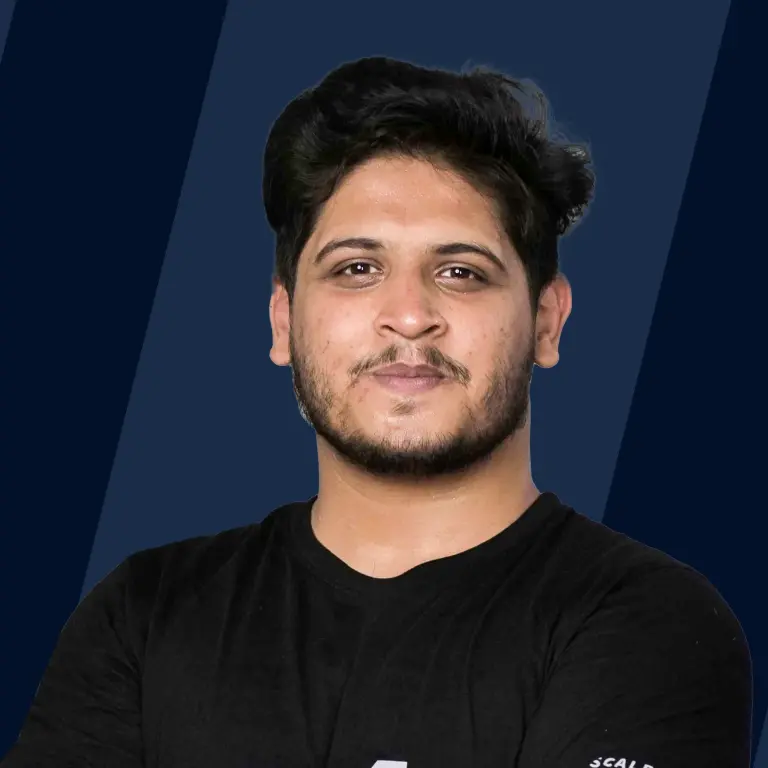
Overview
The JavaScript's appendChild() method is a method of the node interface (an abstract base class in javascript on which many document API objects are based) that adds a child node at the end of the specified parent element.
Meaning the appendChild() method is used to add a new child element at the end of a parent element, along with this, the method can also be used to move an existing child element within the document from one parent element to another.
Syntax of JavaScript appendChild() Method
The method appendChild in JavaScript is applied on the parent node in which we want to append the created child.
Parameters of JavaScript appendChild() Method
The method appendchild in javascript in JavaScript takes only one parameter
- childNode: The node that we want to append to the parent node
Return Value of JavaScript appendChild() Method
The method appendchild in javascript returns the appended child node, but if the passed childNode is a DocumentFragment object ie, a document object that has no parents, then in that case the empty DocumentFragment is returned.
Exceptions of JavaScript appendChild() Method
There are as such no exceptions or exceptional use cases to the method appendchild in javascript
However, the method might throw some exceptional warnings, if the constraints of the DOM tree are violated, ie. if either of the following cases happens
- If the parent of the childNode is not a Document, a DocumentFragment, or an Element
- If the insertion of the childNode will lead to a loop cycle, ie. if the childNode is a parent of the considered parent node
- If the childNode is not a DocumentType, DocumentFragment, Element or CharacterData
- If the childNode is Text, and the parent node is Document
- If the childNode is of DocumentType, and the parent is not a Document (as a doctype should always be the direct descendent of a document)
- If the parent of the childNode is Document, and the childNode is DocumentFragment with more than one Element child or having a Text child
- If the insertion of childNode would lead to a Document with two or more elements as children.
Example
In the below example, we are having an unordered list with only 1 child, and then using JavaScript's appendChild() method, we are adding more child elements to the parent list
Output before using appendChild() method
Output after using appendChild() method
How does the JavaScript appendChild() Method Work?
The JavaScript’s appendChild() method adds a child node at the end of the specified parent element, but if the passed child is a reference to an existing child element in the document, then the appendChild() method automatically moves it from its present position to the new position ie. we need not to worry about removing an element from one parent and then appending the removed child to the new parent element, the appendChild() method automatically does that for us.
Taking the above example, the appendChild() method in javascript can be applied to the parent elements in the DOM. Firstly we capture that specific parent element using query selectors, and then we call the appendChild() method on that parent, by passing a childNode or a function which will create the childNode, the method then will loop through the existing child nodes of the parent element and will append the passed child node at the end, meaning on successful execution, a child node is appended at the end of the specified parent element.
More Examples
Example 1. Appending Multiple Child Nodes
In this example, we are appending multiple child nodes to the parent element. Here, firstly we are capturing the unordered list parent element, and then with the help of the appendChild() method, we are inserting an array of numbers as children into the parent list.
Output before using appendChild() method
Output after using appendChild() method
Example 2. Moving a Child Node Within the DOM
We can also use the appendChild() method to move a child element, from one parent to another. Here in this example, we are having two lists having Alphabets and number elements respectively, what we are doing here is, we are capturing the first parent and after that, we are also getting the first child of the first parent element, and then we are capturing the second parent element and finally we are appending the first child of the first parent onto the end of the second parent.
Remember the method appendChild() moves an element from one parent to another, which means as soon as it is appended to the second parent, it will be removed from the first parent, ie. it can not exist in both places at the same time, but if we want to do so, then we can create a new element same as the first child and then can append that to the second parent instead of moving the original one.
Output before using appendChild() method
Output after using appendChild() method
Supported Browsers
Browser Name | Supported on versions |
---|---|
Internet Explorer | version >= 6 (Aug 2001) |
Mozila Firefox | version >= 2 (Oct 2006) |
Safari | version >= 3.1 (Mar 2008) |
Google Chrome | version >= 4 (Jan 2010) |
Microsoft Edge | version >= 12 (Jul 2015) |
Conclusion
- The appendChild() method in JavaScript is used to append a new child element at the end of a parent element.
- It can also be used to move a child element within the document ie. moving a child element from one parent to another.
- Syntax : parentNode.appendChild(childNode);
- The appendChild() method takes only one parameter, a childNode that we want to append to the parent element
- The function returns the appended child node itself, but if the passed child is a DocumentFragment object ie. a document object that has no parents, then in that case the empty DocumentFragment is returned.