JavaScript Program to Insert Item in an Array
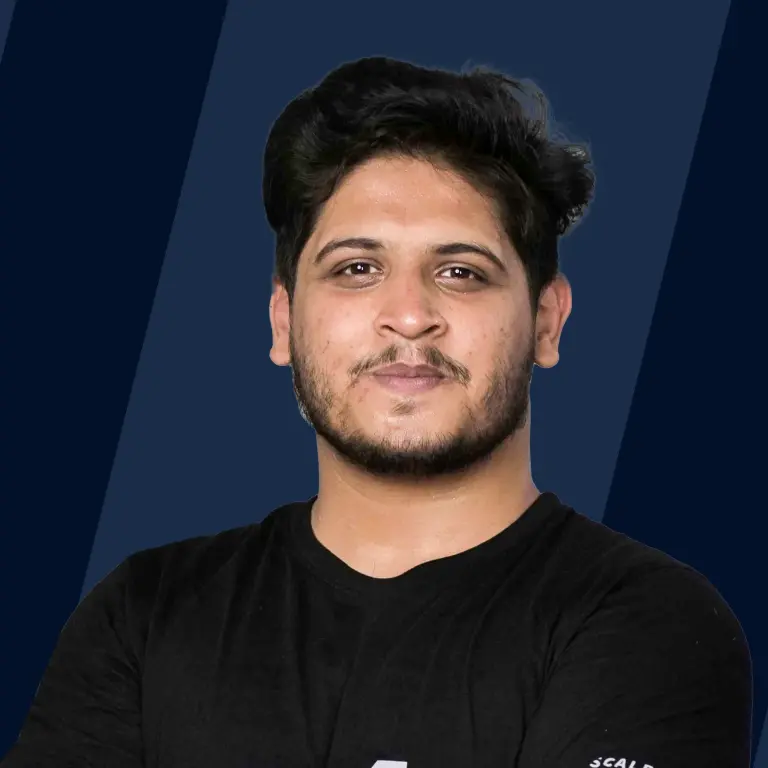
Overview
JavaScript is a scripting language that allows you to do almost everything, including dynamically updating the material, managing multimedia, etc. Arrays are unique kinds of objects. Modifying an array in terms of its values and size is also possible in JavaScript, which is impossible in many other languages like C++, C, etc. This makes an array a very powerful tool, as it stores this information so that it can be easily retrieved by using square brackets "[]" and the item's index.
Introduction
JavaScript doesn’t have any inbuilt functions to modify an array. There are some techniques that we have to use if we want to insert, delete or replace an element in an array. We can divide these techniques into two parts based on whether it modifies the original array or not.
Note: Array's items are stored in 0 indexed form means the first element is at index 0, the second at index 1, and so on.
JavaScript Program to Insert Item in an Array Using splice()
The splice method is used to insert, delete, and replace an item in an array. We can also insert more than one item at a time using this method. This method changes the original array instead of making a copy of it.
Syntax:
Parameters:
- arrayName - is the name of the array in which we have to insert an item.
- Position - is the index from where we have to insert our items.
- deleteCounts - denotes the number of elements we want to delete starting from our given position. But for insertion only, we keep the variable equal to zero always.
- Item1, item2, ….., itemN - represents the items we want to insert starting from index “position.”
- newArray - it will contain the deleted elements in the form of an array.
Example:
Output:
In the above example, we have changed the original array by inserting item 4 at position 3. The console also shows an empty array at the second line because the splice() method returns the deleted elements in the form of an array, but in our case, we put deleteCounts equal to 0; that’s why it will return an empty array in case of only insertion.
JavaScript Program to Insert Item in an Array Using for Loop
When we need to repeat/loop a line of code until a specific condition is not equal to false, we use the For loop. We can use it to iterate over our containers or, in our case, all the items in an array. When we want to insert a new item into our array at a specific index (position), then all the elements with an index i>=position must move one position to the right, i.e., the ith item will now be at position i+1th index, i+1th index to i+2th index, and so on.
Example:
Output:
The above example is similar to the example taken for the splice() method, but now insertion is done using a for loop. We iterate our array from the end and shift each item having an index greater than position by one item to its right. And finally, insert the item at index = position with the given item value, i.e. 4.
JavaScript Program to Insert Item in an Array Using push()
This is another method by which we can insert one or many items into the array. It will append items at the end of the array. This method changes the original array, like the splice() method.
Example:
Output:
In the above example, by using the push method in the days, first, we append "Wednesday" at the end of it, and then we append three items in one go as well. Its return type is the size of the new array formed.
JavaScript Program to Insert Item in an Array Using unshift()
We have a unshift() method by which we can insert one or many items into the array. Just like the push() method appends the items at the end, it will append items at the start of the array. It also returns the size of the new array formed.
Example:
Output:
In the above example, by using the unshift() method in the days, firstly, we append "Thursday" at the start, and then we append three items in one go.
JavaScript Program to Insert Item in an Array Using concat()
This method is used to append two arrays into one single array, and also we can insert new items which are previously not stored in an array. This method is a little different from the previous methods because this will create an entirely new array that doesn’t have any connection with the previous one, i.e., any changes in the new array don’t reflect in the previous original array.
Using this method, we can concatenate many items which have different types as well. There are two methods by which we can use the concat() method.
Parameters:
- array1 - It represents the first array that we want to join, which is another one, and array1 elements on the left side mean at the beginning.
- array2 - It represents the second array which is on the right side.
- newArray - It is the new array that will contain the items of both arrays, which is entirely independent of both arrays.
Parameters:
- array1 - It represents the first array that we want to join, which is another one, and array1 elements on the left side mean at the beginning.
- array2 - It represents the second array which is on the right side.
- newArray - It is the new array that will contain the items of both arrays, which is entirely independent of both arrays.
- [ ] - It represents we start with an empty array and then append both arrays one by one.
Example:
Output:
In the above example, we use both methods one by one, giving the same results, i.e., the array containing the items 1 to 6. After that, when we changed array1's value from 2 to 7, then the newArray1 and newArray2 didn't have any changes reflected in the console because it is independent of the original arrays.
JavaScript Program to Insert Item in an Array Using the New ES6 Spread Operator
We may rapidly duplicate all or a portion of an existing array or object into another array or object using the JavaScript spread operator (...). The use of the spread operator to clone an array or produce shallow copies of other JS objects is widespread.
When calling a function, we can pass an array element as a single argument by using the spread operator rather than giving the entire array as an argument. The spread (...) syntax is an eraser to remove the array's brackets.
It is similar to that of using the concat() method, but it's much easier than using the concat() method because it only requires you just to wrap the arrays you want to join into squared brackets "[ ]" and add three dots (...) before every array.
Example1:
Output:
newArray is the new array formed by merging the two arrays(array1 and array2). And when we change the array1 index one value to 10, it doesn’t show any changes reflected on the new array because it is a shallow copy of the two arrays.
Example2:
Output:
The above example clearly shows the changes only get reflected in the deep copy array and not in the shallow copy (created using the spread operator).
JavaScript Program to Insert Item in an Array Using the Arrays Length Property to Append an Element
This is the last technique by which we can insert an item in the array. Using the array length property means by using the length of an array, we will insert an item at the end of the array. As we already noted in the beginning that the array in JS is 0 indexed base, that’s why the last item in the array is at the index=array_length-1 and when we add a new item at the end of the array, then it should be at the index=array_length.
Note: By using this property, we can only insert items at the end of the array and not in any other positions because it only changes the values at other positions.
Example:
Output:
This technique also changes the original array and does not make a separate copy.
Conclusion
- JavaScript uses an array to store most of its information as it is easy to retrieve data from an array.
- Two types to categories the insertion technique are :
- Make a new array and then insert
- Make changes to the original array
- The various techniques to insert items in an array: using splice(), for loop, unshift(), push(), Concat(), spread operator(...), and an array length property.
- An item in an array can be added, deleted, or replaced using the splice method.
- Two arrays can be joined together using the Concat() technique, and additional items that weren't previously stored in an array can be added.