JavaScript Array join()
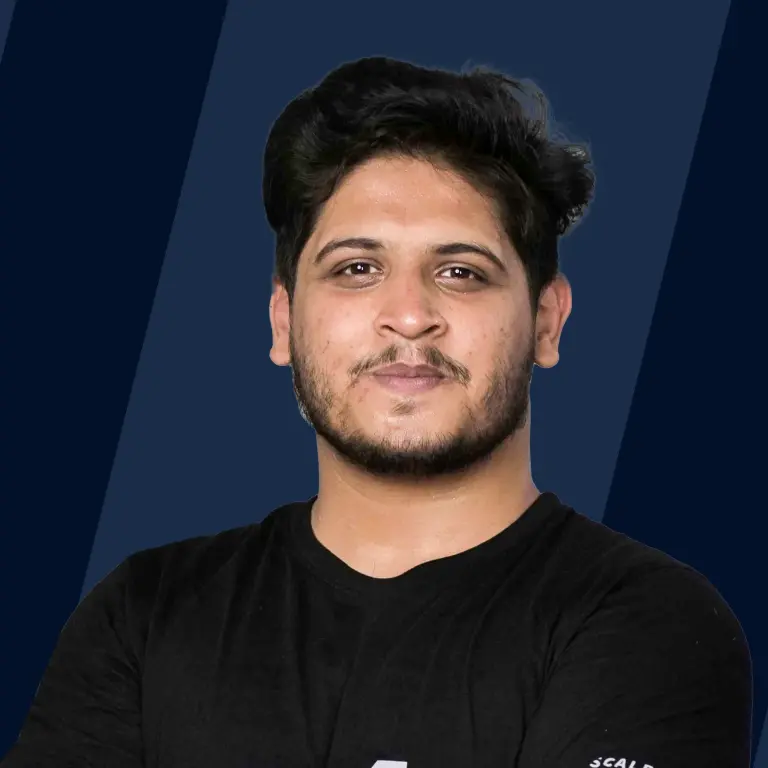
Overview
As the name suggests, the join() method in JavaScript is used to join all the elements of an array to a string and return that resultant string, i.e., a new string is returned. If a separator has been provided, the elements of the array are then separated using the separator, or else, a comma acts as a default separator for join in JavaScript.
Syntax of Array join() in JavaScript
The following is the syntax of Array join() in JavaScript:
The input is an array and the output is a string. It doesn't change the original array.
Parameters of Array join() in JavaScript
The following is the parameter of Array join() in javascript:
- Separator(optional): The separator is an optional parameter. It specifies a string that is used to separate the elements of the array and return the string. The default separator is a comma(,).
Return Value of Array join() in JavaScript
Return Type: string
The join method in JavaScript returns a string with all the elements joined. If the size of the input array is 0, an empty string is returned. If the array is null or undefined, an error will be thrown- Cannot read properties of undefined or Cannot read properties of null for the input array whose value is set as undefined or null, respectively.
Example
Let us now join an array using the join() function.
Code
The image shows the representation of the array join() function in JavaScript.
Output
Explanation
In the above code, we can see that the array has been joined using the JavaScript array join function, and since we haven't provided any separator, the default separator is a comma.
What is Array join() in JavaScript?
As a programmer, many times, we have to return the elements of the array together in the form of a string instead of an array. At that time, the join() function plays a significant role.
The JavaScript array join() is a function provided by JavaScript which takes an array as an input and returns a string that consists of all the elements of the array joined by a separator. The separator is an optional parameter as the default value is a comma(,). All the elements present in the array will be joined. Using this method, we cannot join specific elements in the array.
The join in JavaScript doesn't change the input array, it only returns the string form of the input array.
More Examples
1. Joining an Array Four Different Ways
Let us create an array and join it using four different ways.
Output
Explanation: We have used four different ways to join the array, i.e., using the default separator, using an empty string, using plus symbol, and using a comma followed by a space.
2. Joining an Array-like Object
In JavaScript array join, we can join an array-like object(arguments) by calling Function.prototype.call on Array.prototype.join. The elements in the nested array are also joined.
Output
Explanation: As we can see in the above example, the elements in both the nested array are also joined.
Browser compatibility
The join method in javascript is supported by all the browsers such as Chrome, Edge, Safari, Opera, etc.
Conclusion
- The join() method in JavaScript is used to join the elements of an array to a string and return that resultant string.
- The input is an array and the output is a string. It doesn't change the original array.
- If a separator has been provided, the elements of the array are then separated using the separator, or else, a comma acts as a default separator for join in JavaScript.