JavaScript BigInt Library
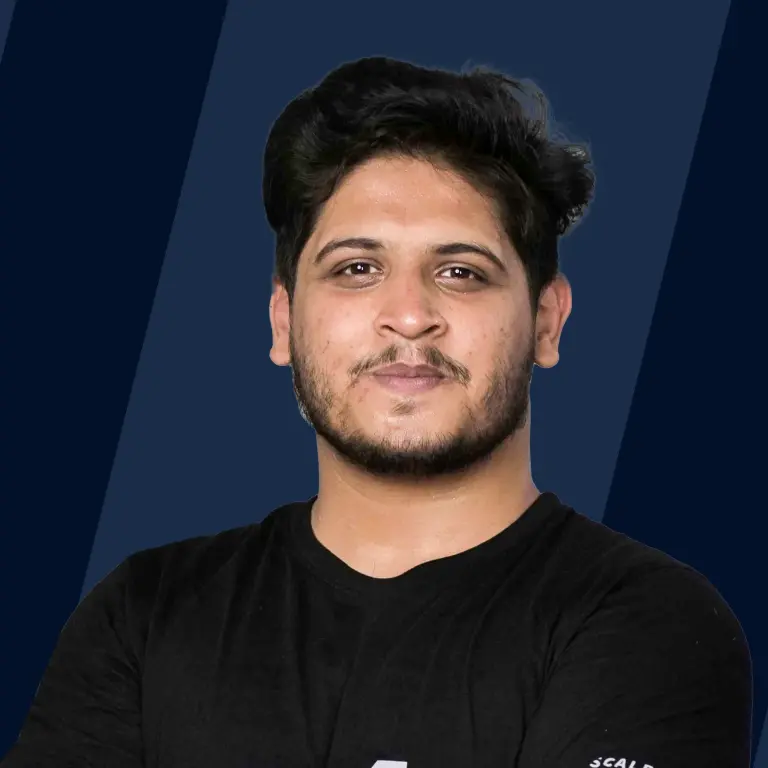
Overview
The JavaScript BigInt is a built-in object in javascript that is used to store the numbers that are greater than Number.MAX_SAFE_INTEGER. There are some rules to create a JavaScript BigInt value. We can make a JavaScript BigInt by adding n to the end of any integer literal that we want to change to a JavaScript BigInt value. Also, we can use the BigInt() function and then provide an integer value to the function to change the given integer to the JavaScript BigInt value.
JavaScript BigInt
The BigInt is a library in JavaScript that is used to store and represent numbers that are bigger than 15 digits in length. As we know in javascript, the maximum length of an integer that can be stored in a variable is 15 digits. After that, the number gets distorted or unexpected errors may happen. So, The JavaScript BigInt provides the user a way to store and represent the numeric values that are larger than that particular parameter Number.MAX_SAFE_INTEGER. There are eight data types supported in the javascript after the addition of the BigInt that are String, Bigint, Number, Boolean, Object, Null, Symbol, and Undefined.
In the javascript language, all the integer values are stored in a 64-bit floating point format (IEEE 754 standard). The JavaScript BigInt value is also known by simply calling it "BigInt". It is a primitive data type in javascript that can be created by adding n at the end position of the integer. We can also do this by using the BigInt() function and passing the integer value as the parameter of this function using the new operator.
While there are some similarities between BigInt and Number values, there are also some significant differences. BigInt values must be coerced to the same type to be utilized with built-in Math object methods or combined with Number values in operations. However, be cautious when coercing data back and forth as a BigInt value's accuracy may be lost when it is converted to a Number value.
Let us take an example to understand why we need a Javascript BigInt object.
The result for the above mathematical operation should be 9007199254740993 ideally. But here we are getting 9007199254740992 as the resultant output. This is happening because the javascript used 64-bit for storing the integer values. And here we are creating a larger value as the output of the operation. So, the javascript is unable to store such a large value and we are not getting the precise output of the operation. To overcome this problem the javascript used a JavaScript BigInt library.
Syntax
The syntax of the JavaScript BigInt is as follows:
To convert an integer literal to a BigInt value, we can use the BigInt() function and under the function, we can assign any integer value. Then this function will convert the integer literal into the JavaScript BigInt value.
Parameter
The parameter of the JavaScript BigInt is as follows:
- Integer literals- The BigInt accepts integer literal as a string as its parameter.
Return type
The return type of the JavaScript BigInt is as follows:
- Return type- The BigInt returns the BigInt value as the return value.
Examples
Let us take a short and simple example to understand JavaScript BigInt.
Output:
Explanation: In the above example, we have taken three different types of integer integrals as the parameter. The variables are namely BigNum, BigHex, and BigInt and assigned the value of "123422222222222222222222222222222222222", "0x1ffffffeeeeeeeeef", and "0b1010101001010101001111111111111111" respectively. With each of these parameters, we used the BigInt function to change the integer integrals into the JavaScript BigInt value. Then we use the console.log to get the resultant output of the JavaScript BigInt function.
JavaScript BigInt Other Types Comparison
Now let us compare the JavaScript BigInt type with other types in javascript. The BigInt is almost similar to the integer values but they are not an integer. So, it is not advised to use the JavaScript BigInt values as integer values with the various method of the built-in Math object of JavaScript. Also, it should not be mixed with the instances of numbers while operations.
Let us see a comparison of JavaScript BigInt with a number.
One point that needs to be mentioned is that the comparisons with Object-wrapped BigInt values behave like comparisons with the other objects, with the same object instance serving as the only criterion for equality. Certain conditions need to be followed while doing the comparison. The BigInt value also follows the same rule as numbers when:
- A JavaScript BigInt value is converted into a boolean value by the use of the boolean function.
- When we use the logical operators with the JavaScript BigInt values.
- When the JavaScript BigInt values are used with conditional operators like the if or else statement.
Sorting in JavaScript BigInt
While working with the JavaScript BigInt library, there are some situations in which both the BigInt value and primitive data types are present in the program. So, we use the sorting technique to sort the given parameters in ascending order. For sorting the parameters in the array, we use the sort() method.
Let us see an example of sorting the BigInt values and normal Number values using the sort() method.
Output:
Explanation: In the above example, we have used the sort() method for sorting the JavaScript BigInt values and normal Number values in an array. First, we created a variable namely array_1 and assigned it the values [6, 3, 4n, 7n]. Then we used the sort() method with the variable name array_ to sort the array. After that, we used the console.log with array_1 as the parameter to get the output.
JavaScript BigInt Usage Recommendations
There are also some drawbacks to the JavaScript BigInt library. So, the fields where the use of the JavaScript BigInt library is not recommended are discussed below:
Coercion: When coercion is done between the Number values and the BigInt values, it leads to a lack of precision in the calculation that alternatively affects the resultant outcome. That's why there is some recommendation to overcome this which are as follows:
- We should use the JavaScript BigInt value only when there are expectations that the value will get larger than .
- We should avoid doing coercion between the BigInt value and the Number value.
Cryptography: Timing attacks can be used against the supported operations on BigInt values because they are not constant-time. Therefore, using BigInt value in encryption without any protective measures could be risky. In a very general example, an attacker may calculate the time difference between 101n ** 65537n and 17n ** 9999n and use that information to estimate the size of secrets, like private keys. If you must continue to utilize BigInt value, consult the Timing attack rules FAQ for general guidance on the matter.
Use with JSON: BigInt numbers aren't serialized in JSON by default, so using JSON.stringify() with any BigInt value will result in a TypeError. A backdoor is left open for BigInt values by JSON.stringify(), which will attempt to call the BigInt's toJSON() method. In no other case does it apply to primitive values. In light of this, you can buy a toJSON() method ():
A string will be created in this case despite the JSON.stringify() that will look like this:
If the user does not want to rebuild the BigInt.prototype, the user should use the parameter namely replacer of the JSON.stringify to serialize the Javascript BigInt values. Given below is the representation of how we can use this:
If we have the JSON that has some values in them, we can also use the parameter namely reviver of the JSON.parse because the value will be larger in this case.
Browser Compatibility
The BigInt value is supported by almost all modern browsers. Given below are the name of some popular browsers that supports the JavaScript BigInt library.
Browser Name | Supported on versions | Version date |
---|---|---|
Mozilla Firefox | 68.0 and above | July 2019 |
Microsoft Edge | 79.0 and above | January 2020 |
Google Chrome | 67.0 and above | May 2018 |
Safari | 14.0 and above | September 2020 |
Opera | 54.0 and above | June 2020 |
More Examples for Understanding
Let us take another example where we can use the JavaScript BigInt library to calculate the prime numbers.
FAQs
There are some frequently asked questions about the JavaScript BigInt data types that we are going to cover in the given section:
Q: How to create a JavaScript BigInt?
A: For creating the JavaScript BigInt, two ways are as follows:
- We can add n to the need of the given number.
- We can use the BigInt constructor.
Q: What are the arithmetic operations that can be performed on a BigInt?
A: BigInt math operations are largely analogous to math operations on normal numbers. However, the fractional portion of the result is eliminated when we divide. We can also do operations such as equality comparison, relational operations, conversion to boolean, and conversion to string.
Q: What are Minimum and Maximum Safe Integers?
A: The min and max are the two properties that were added by the ES6 to the Number object.
-
MAX_SAFE_INTEGER- The MAX_SAFE_INTEGER is a constant that is used to represent the maximum integer value in javascript that is safe to use in any operation of the javascript. The MAX_SAFE_INTEGER in javascript is (253 – 1).
-
MIN_SAFE_INTEGER- The MIN_SAFE_INTEGER is a constant that is used to represent the smallest integer value in javascript that is safe to use in any operation of the javascript. The MIN_SAFE_INTEGER in javascript is -(253 - 1).
Conclusion
- The JavaScript BigInt is a built-in object in javascript that is used to store the numbers that are greater than Number.MAX_SAFE_INTEGER.
- There are two methods of converting an integer value into a JavaScript BigInt value.
- The first one is we can make a JavaScript BigInt by adding n to the end of any integer literal.
- The second method is can use the BigInt() function and then provide an integer value to the function to change the given integer to the JavaScript BigInt value.
- The syntax of the JavaScript BigInt is BigInt(number).
- The parameter of the BigInt is Integer literals and the return type of the BigInt is BigInt value.
- The JavaScript BigInt is almost similar to the integer values but they are not an integer.
- the fields where the use of the JavaScript BigInt library is not recommended are when we perform coercion, Cryptography, and Use with JSON.
- Timing attacks can be used against the supported operations on BigInt values because they are not constant-time. Therefore, using BigInt value in encryption without any protective measures could be risky.
- Using the JSON.stringify() with any BigInt value will result in a TypeError.