What are JavaScript Bitwise Operators?
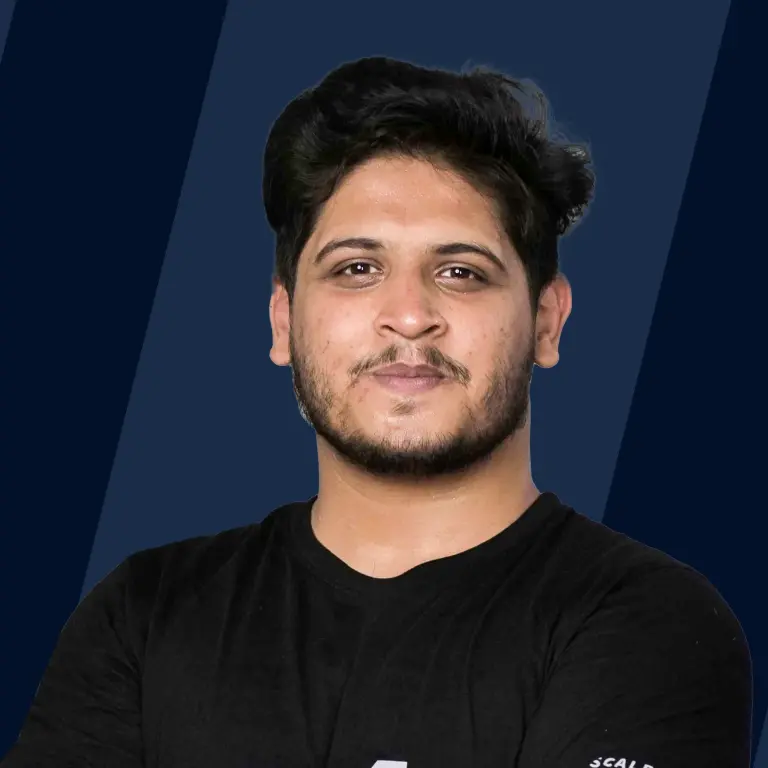
Javascript has binary operators to handle numbers as a sequence of 32 bits. Normally, javascript stores numbers as 64-bit integers, and then when performing a bitwise operation, the numbers are first converted to 32-bit. These bitwise operators can be used to carry out simple arithmetic operations like addition, multiplication, etc.. much faster than using normal methods.
The bitwise operators in Javascript are,
Name | Operator | Example |
---|---|---|
Bitwise AND | & | a&b |
Bitwise OR | | | a|b |
Bitwise XOR | ^ | a^b |
Bitwise NOT | ~ | ~a |
Bitwise Left Shift | << | a<<b |
Bitwise Right Shift | >> | a>>b |
Zero fill left shift | <<< | a<<<b |
Zero fill right shift | >>> | a>>>b |
The above table gives the names and examples of the bitwise operators in Javascript. The a and b represent the operands(numbers) that must be used with the operator. It is important to note that the bitwise operators work separately on each bit of the numbers.
A bit has a value of 1 or 0. Each bit has an assigned value based on the decimal place using the formula,. The first decimal place is counted from the right and starts from 0. Consider the number 5, it can be expressed in bits as, 00101.
Examples for JavaScript Bitwise Operators
Let us deeply understand each bitwise operator with examples,
JavaScript Bitwise AND
The Bitwise AND operator checks the bits on the same decimal position of both the numbers and returns a 1 if both the bits are 1 or returns 0. The bitwise AND is represented by the & operator. The truth table for AND operator is,
Operand 1 | Operand 2 | Output(&) |
---|---|---|
1 | 1 | 1 |
1 | 0 | 0 |
0 | 1 | 0 |
0 | 0 | 0 |
Let us use the & operator on the numbers 10 and 12,
10 = 0 0 0 0 1 0 1 0
& 12 = 0 0 0 0 1 1 0 0
-----------------------
0 0 0 0 1 0 0 0 = 8
From the above example, we can see that the bits become 1 only when the bits in both numbers are 1.
Note: Please note that every number in Javascript will be represented as a sequence of 32 bits, we have taken fewer numbers of bits for simplicity.
We can implement the above example using Javascript as follows,
The output will be,
JavaScript Bitwise OR
The Bitwise OR operator checks the bits and returns 1 if any one of the numbers has 1 and returns 0 only if both the numbers have 0 bits. The bitwise OR operator is represented by the | symbol. The truth table for the OR operator is,
Operand 1 | Operand 2 | Output(|) |
---|---|---|
1 | 1 | 1 |
1 | 0 | 1 |
0 | 1 | 1 |
0 | 0 | 0 |
Let us use the | operator on the numbers 10 and 12,
From the above example, we can state that the bits become 0 only if both the bits are 0 and in all other cases the result will be 1.
The above example can be implemented in Javascript as follows,
The output will be,
JavaScript Bitwise XOR
The Bitwise XOR operator returns 1 when both bits are different and returns 0 when both bits are the same. The bitwise XOR operator is represented by the ^ symbol. The truth table for the XOR operator is,
Operand 1 | Operand 2 | Output(^) |
---|---|---|
1 | 1 | 0 |
1 | 0 | 1 |
0 | 1 | 1 |
0 | 0 | 0 |
Let us perform a bitwise XOR operation between 10 and 12,
From the above example, we can see that the operator returns 1 only if both bits are different.
Let us perform the above operation using the Javascript bitwise operator,
The output of the above example will be,
JavaScript Bitwise NOT
The bitwise NOT operator is used to invert the bits or simply convert 0 to 1 and 1 to 0 on all bits of a number. It is represented by the ~ symbol. The truth table for NOT operator is,
Operand | Output(~) |
---|---|
1 | 0 |
0 | 1 |
Let us perform a NOT operation on the number 10,
The result is much larger than the number in which bitwise NOT operation is performed.
The binary output is called the 2's compliment and is used to represent negative numbers as binary digits.
Let us implement the above operation using Javascript,
The output will be,
The result of a bitwise NOT operation will always be -(number + 1). In our above example, the number was 10 and the result was -11.
JavaScript Bitwise Left Shift
The bitwise left shift operator in Javascript involves shifting a specified number of bits to the left in a number. The first operand is the number and the second operand is the number of bits to shift left in the number. The Javascript bitwise operator is represented by the << symbol.
Let us shift 3 bits to the left in the number 10,
Since the decimal places to the left have a greater value than the decimal places to the right, shifting the bits to the left has increased the value of the result. The bits that move out of the 32 decimal places will be discarded.
Let us implement the above option using Javascript,
The number of bits to be shifted will always be between 0 and 31, since Javascript performs a modulo operation with the number 32 on the operator b to make sure that the number of bits to be shifted is between 0 and 31. The above example will be executed as,
The output will be,
JavaScript Bitwise Right Shift
The bitwise right shift operator in Javascript is used to shift a specific number of bits to the right in a number. It is represented by the >> symbol.
Let us perform an operation in which 2 bits will be shifted to the right in the number 10,
Shifting the bits to right makes the result smaller than the number in which the operation is performed. This is because the decimal places on the right side have less value than decimal places on the left side. The bits that move out of the 32 decimal places will be discarded. In this example, the and bit of the number 10 are discarded.
Let us implement the above example using the bitwise right shift operator in Javascript,
The right shift operator also has the same property as the left shift operator in terms of the number of bits that can be shifted. There will be a modulo operation with the b operand and the number 32 to make the result be within 0 and 31.
The output of the above example will be,
We can perform all these operations in negative numbers too. As previously stated in the article, a 2's complement is used for negative numbers. For example, the number -8 will be represented as 11111000 in 2's complement. The subtraction operation takes place as an addition between the first argument and the 2's complement of the 2nd operand.
Why Do We Use JavaScript Bitwise Operators?
There are many use cases and advantages for bitwise operators in Javascript. These are explained in the following points,
- The Bitwise AND operator can be used to find whether a number is odd or even. A number will be odd only if the first bit is set to 1, we can utilize this property and perform a bitwise AND operation with 1 to find whether the number is odd or even. The following example illustrates this,
Let us visualize the AND operation in the above example for the value 10,
From the example, we can say the answer depends on the last bit only. The output will be,
- We can also turn off bits(set to 0) using the bitwise AND operator. While using the bitwise AND operator, if a bit in one number is 0, then the result is always 0. This property can be used to set bits to 0. The following example illustrates this,
The last four bits are turned off using the number 15. This can also be used to find if a bit is turned on(set to 1) at a particular position too.
- The Bitwise OR can be used to set bits in the result to 1. In OR if a bit in one number is 1, then the result will be 1. The following example illustrates this,
The last four bits are set to 1 by using the number 15.
- The Bitwise XOR is used to toggle bits or change a bit from 0 to 1 and 1 to 0. The following example illustrates toggling of all bits except the first four bits,
- The Bitwise NOT operator can be used as an indicator for methods that return -1 on error or failure. The value of -1 is 0 and we can use this property to check for errors. This can be used with methods like String.indexOf() which returns -1 when the index is not found.
The output will be,
- The Left shift operator can be used to convert color from RGB format to hex format. Normally colors are stored as 24-bits, with 8-bits each representing red, blue, and green colors respectively. We can shift each color to its respective position in the 24-bit sequence and perform a bitwise OR to get the hex value of the color,
The output will be,
The above example can be visualized as,
-
The left shift operator can also be used mathematically. The operation, is similar to the mathematical operation, KaTeX parse error: Expected 'EOF', got 'ⁿ' at position 4: a*2ⁿ̲.
-
The Right shift operator is used to extract the colors from RGB values. In all positions except those that can be used by a particular color, we use a bitwise AND operator with 0xff(11111111) to set the bits to 0.
This can also be implemented with Javascript as follows,
- The right shift operator can also be used mathematically. For example, the operation, is similar to the mathematical operation, KaTeX parse error: Expected 'EOF', got 'ⁿ' at position 4: a/2ⁿ̲.
Learn About Operator Precedence in JavaScript
Consider, the following example in which there are more than two bitwise operators in an expression,
In these cases, there is a problem with which bitwise operation will be executed first and which operation will be executed next. The concept of precedence solves this problem. The precedence determines the order of execution of the bitwise operators. In the above example, the b & c operation takes place before the operation. Learn more about precedence in this article.
Conclusion
- Javascript bitwise operators are used to handle numbers as a sequence of bits directly.
- There are different types of bitwise operators which can be used to perform different functions faster and with less memory than other methods.
- There are many use cases for the bitwise operator.
- The precedence of Javascript bitwise operators must be considered when more than two bitwise operators are present in an equation.