How do You Break a JavaScript Statement?
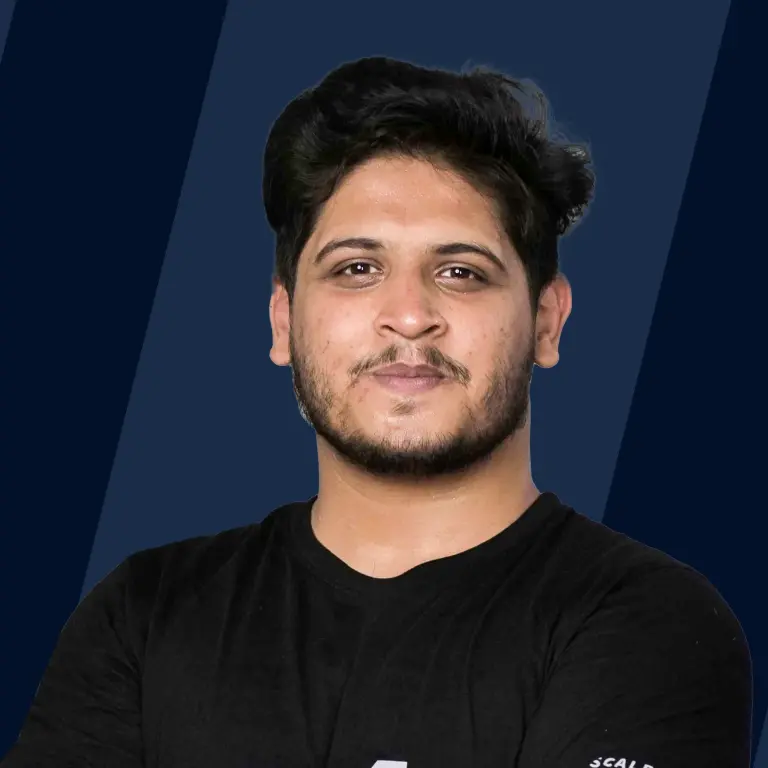
The break statement in javascript is used to break or terminate the loops and the statements immediately as soon as the break keyword is encountered.
Syntax
The break keyword is used to terminate or break out of the loop. It can be applied in the for loop, while loop, do while loop, etc... But it can not be applied in statements where there are no loops. So in that case we have to use the label keyword.
Syntax
Description
The break statement as we know is used to break or terminate the loop immediately as soon as it is encountered. Although It can only be applied inside the loops like for loop, while loop, do while loop, etc... But it can not be applied in statements where there are no loops. In that case, we have to use the label with the break keyword, to terminate the statement.
The break statement has many advantages. It helps the programmer to terminate the statement or loop whenever further statements do not need to be executed. Also, the while loop saves us from the situation of an infinite loop.
Working of JavaScript break Statement
Output
In the above example, syntax and working of break are shown. We can see how we can break the loop according to the requirements. Here we have used the break statement as soon as the value of the counter reaches 4, and accordingly, the loop is terminated, showing the above output.
Output
In the above example, syntax and working of break with label are shown. We can see how we can break the statement according to the requirements. Here we have used the break statement as soon as we have printed the name of the first 3 courses, and accordingly, the statement is terminated, showing the above output.
Examples
Break with for loop
The following example shows the break keyword used inside a for loop. As soon as the counter becomes 7, the for loop will terminate showing the following output.
Output
Break with while Loop
The following example shows the break keyword used inside a while loop. The program finds the factorial of 5, and as soon as the x becomes greater than 5, the loop will terminate showing the following output.
Output
Break with Nested Loop
The above example shows the break keyword used in a nested for loop. The break is applied inside the if statement when the value of i is either 1 or 2, the inner loop will terminate showing the following output.
Output
JavaScript Labeled break
The following example shows the break keyword used with a label in a list. The break is applied as soon as we print the first 3 cities, showing the following output.
Output
Break in Labeled blocks that throw Error
The following example shows the break keyword used with the label in the labeled blocks. The break is applied inside block1 with the label as block2, but the compiler does not understand the meaning of block2 because block2 is declared after block1.
Break within Functions
The following example shows the break keyword used within the function. The break is used inside the function which is not allowed in Javascript, so the compiler will throw an error.
Browser Compatibility
The browser compatibility of the break keyword is as follows
Browser | Version |
---|---|
Google Chrome | 1 |
Microsoft Edge | 12 |
Mozilla Firefox | 1 |
Opera | 4 |
Safari | 1 |
Chrome Android | 18 |
Firefox for Android | 4 |
Opera Android | 10.1 |
Safari on iOS | 1 |
Samsung Internet | 1.0 |
WebView Android | 4.4 |
Deno | 1.0 |
Node.js | 0.10.0 |
Conclusion
- In this quick tutorial, we have discussed and learned how to use break in Javascript.
- We learned the syntax and working of javascript with the help of relevant examples, which covers every case
- Finally, we saw the browser compatibility of the break statement.