JavaScript clearInterval()
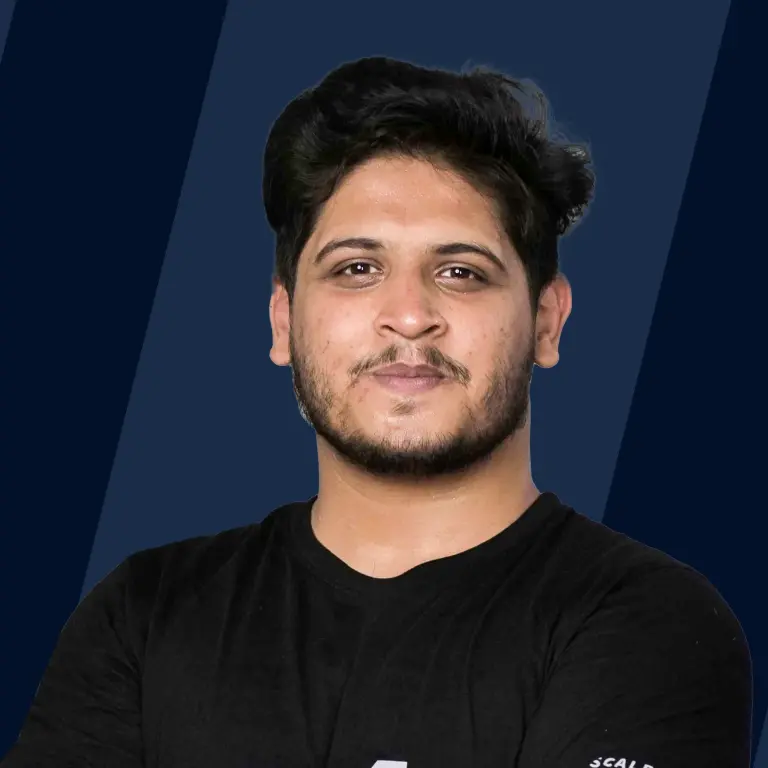
Overview
The clearInterval function in javascript is used to stop the event of the recurring calling of a function at a fixed delay set by the setInterval() function. This function can be used only when a setInterval() function is previously used.
Syntax of JavaScript clearInterval()
The syntax of clearInterval() function is,
Parameters of JavaScript clearInterval()
- We must pass a single parameter ID while calling the clearInterval() function.
- The ID represent the random event number returned by the setInterval() function.
- The clearInterval() function depends on setInterval() function which has the following syntax,
- The time_delay should be given in microseconds. For example, 3000 microseconds will be 3 seconds.
- The setInterval() will call the function repeatedly periodically with a delay of value,time_delay.
- This function returns a random number assigned to the generated event.
Return Value of JavaScript clearInterval()
-
The clearinterval javascript function has no return value and hence returns undefined.
-
The undefined is a primitive data type in javascript which is used to represent nothing or no value.
Exceptions of JavaScript clearInterval()
- The clearInterval() function needs the ID of the setInterval() function to work. It becomes hard to implement the clearInterval() function if the ID is not known or the ID is changed by calling setInterval function multiple times.
- On calling the setInterval() function for the first time it returns a random number as ID, and when the function is called again, the ID is just incremented
Consider the following example, in which a setInterval() function is called continuously two times. When the function is called multiple times, the ID value returned by the previous functions will be lost and we will not be able to use the clearinterval javascript method to clear that event generated by the setInterval() function
In the above example, we have called the callSetInterval() function two times continuously, which has led to the loss of the id 24 returned by the first function call. Hence, the first event generated by the function with id 24 can't be cleared by the clearInterval() function.
The output for the following code will be
The output depicts the execution of the PrintFunction() function even after calling the clearInterval() function.
To solve the above problem, all the ID returned by the setInterval() function can be stored in an array, and the clearInterval() function is called on each element of the array separately. This is illustrated in the following example,
The clearInterval() function will not cause any error if the ID previously cleared. Therefore, we can just call the clearInterval() function for every ID stored in the id_array. This we clear all the setInterval() functions.
Example
A simple example of using the clearinterval javascript function will be to stop a counter and run the counter again for 1 second.
- In the above example, the setCount() method is used to start the counter or call the setinterval() function which in turn calls the PrintCount() function periodically for a period of 1 second.
- The PrintCount() function increments the count variable and prints it.
- The stopCount() function calls the clearInterval() function to stop counting.
The output will be displayed with a dealy of 1 second,
How Does JavaScript clearInterval() Work?
The setInterval() function is used to form an event that runs in the background and calls a particular function with a fixed time delay.
The event is created and managed by the Worker object in javascript. The worker object runs events in the background without disturbing the interaction interface. When creating this event, the function associates the event created with an ID, which is a randomly generated number. This ID can't be modified or changed after generation.
The clearInterval() is a method used to find the event with the specified ID and remove it. It is important to note that clearInterval() is the only function that can be used to remove an event started by the setInterval() function.
More Examples
We can create a progress bar that proceeds in a fixed interval of time and can be paused by using a clearinterval javascript function. The following code illustrates this,
- The arr is used to store all the ID generated by the setInterval() function. This is then used by the clearInterval() function to destroy all clear all setInterval() functions.
- We can increase the speed of the progress bar by clicking the StartInterval button as this creates a new setInterval() method on the moveSlider function.
- The progress bar will move until width becomes equal to 100, then will call the clear_events() function to invoke clearInterval function.
- The progress bar is made to move by increasing the width of the div element with id Slider. The following output will be produced by the example,
We can also create a clock and pause the clock with the clearinterval javascript function. The following example illustrates this,
In the above example, the startClock() function is used to call the setInterval() function to generate a event which calls the ChangeTime() function every second.
The clearInterval() function is used to remove the event and hence stop the clock from changing time. In this example, the local time of the browser is captured using the Date() Object in javascript.
The output for the above example will be,
Supported Browser
- The clearinterval javascript function is supported in all browsers.
- It is partially supported in the Node.js environment. This is because node.js needs the Timeout object as a parameter instead of the ID.
- The 'Timeout' is an object returned by the setTimeout function. The setTimeout() function is similar to the setInterval() function, but the function given in the parameter will be called only once.
- Javascript has objects called Workers which help to run an event in the background. The clearInterval() function has the availability to workers in all browsers too.
The following table illustrates the supporting browsers,
Browsers | Support |
---|---|
Chrome | Full Support |
Edge | Full Support |
Firefox | Full support |
Safari | Full support |
Node.js | Partial support |
Conclusion
- The clearInterval() function clears the event of calling a function periodically in a specified time by the setInterval() function.
- The function requires the ID generated by the setInterval() function as a parameter.
- This function can be used to implement timers,progress bar etc..
- The clearinterval javascript function is the only way to stop a event started by the setinterval() function.