What is Continue Statement in JavaScript?
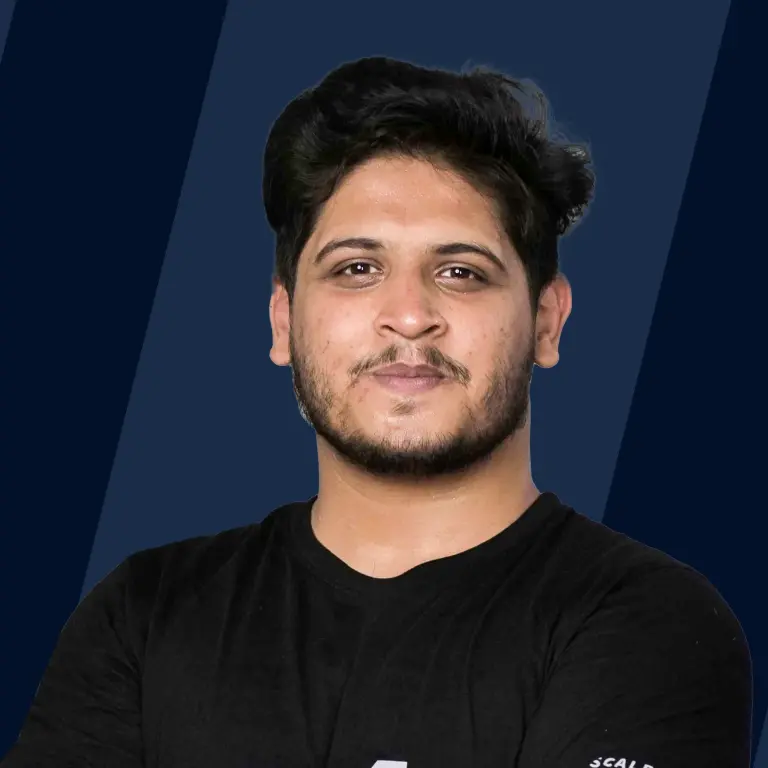
The JavaScript continue statement allows us to terminate the execution upon a condition in the current iteration of the current or labeled loop and continues the loop execution for the next iteration.
When the program encounters the JavaScript continue statement, the control of the program immediately moves to the loop statement where it continues with the next iteration if the condition in the loop still holds.
Syntax
Using the label reference, where the label is optional.
Description
Unlike the break statement, the JavaScript continue statement does not terminate the loop but jumps over to the next iteration.
The continue statement includes an optional label to allow the program to skip to the next iteration of a labeled loop statement instead of looping through the current loop.
The JavaScript continue statement behaves differently depending on the type of loop being executed:
- In a while loop, the loop is executed again only if the condition is true.
- For loops evaluate the increment expression (for example, i--), then test whether another iteration should occur based on the condition.
Working on JavaScript Continue Statement
In the below figure, you'll see how the control of the program moves from the continue statement to the loop statement.
As you can see above, there is a for loop and an if condition which will be executed only if the condition is true. If the condition gets true then the continue statement is encountered and it will skip the rest of the code inside the loop for the current iteration and will jump to the next iteration.
In the case of the while loop, the control of the program only moves to the loop when the condition inside the while loop is still true.
Examples
- Using the continue statement in a for loop
Code:
Output
In the above example, when you carefully see there is no output when the i was equal to 8. Here comes the continue statement which skipped the iteration was the value was 8 due to the if condition.
- Using continue with while
Code:
Output
While loops work very similarly to the for loops except that it checks for the condition first and runs the next iteration.
In the above example, if you see the increment i++; is done before the continue statement because if it is after the continue statement and i is equal to 5 then it will encounter the continue statement and skip the iteration meaning there won't be any increment in the value of i.
- continue with Nested Loop
Code:
Output
In the above example, we use the continue statement inside the inner loop. The continue statement skips the iteration only for the inner loop as you must have seen from the output.
- Using continue with a label
Code:
Output
In the above example, the outer loop is labeled as the FirstLoop and the inner loop is labeled as the SecondLoop. We have the nested loops and the continue statement inside the inner loop.
As you can see from the output, the iteration is skipped when the value of j is 6 and the value of j again starts from 5.
Browser Compatibility
Browsers | Version |
---|---|
Chrome | 1 |
Edge | 12 |
Firefox | 1 |
Opera | 4 |
Safari | 1 |
Chrome Android | 18 |
Firefox for Android | 4 |
Opera Android | 10.1 |
Safari on iOS | 1 |
Samsung Internet | 1.0 |
WebView Android | 4.4 |
Node.js | 0.10.0 |
Deno | 1.0 |
Conclusion
- The continue statement in JavaScript is used to skip the current iteration and move to the next iteration depending on the condition.
- The continue statement in JavaScript can only be used inside loop statements defined with conditional statements.
- The continue statement in JavaScript can also use the label reference, which is optional.
- When a continue statement is encountered, the control of the program moves from the continue statement to the loop statement and is executed only if the condition is true.
- The article covers various code snippets to better understand the use cases.