What is JavaScript do while?
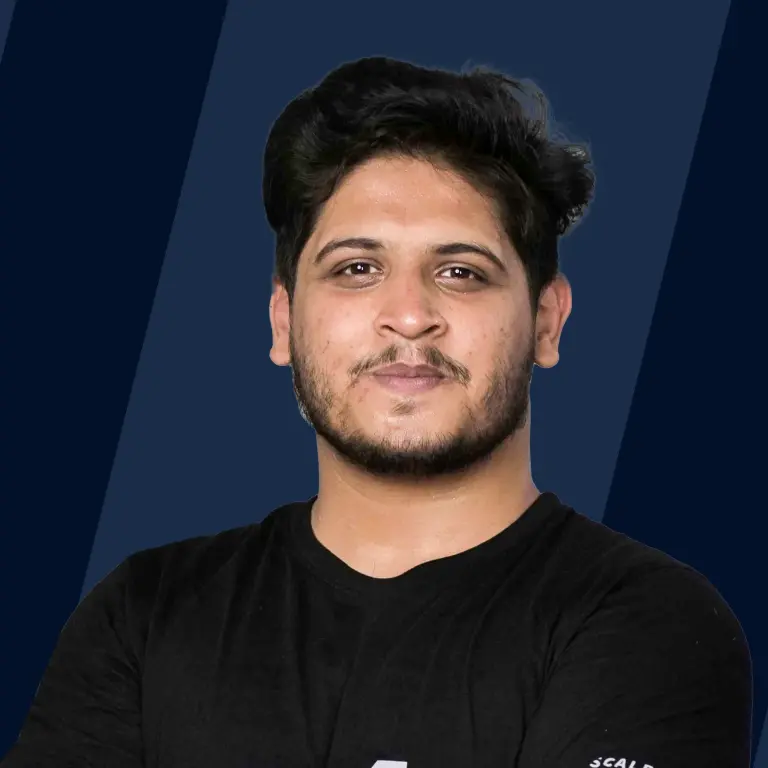
What is JavaScript do while?
In programming, loops are used to execute a block of code repetitively. The loops often make it easier to run a block of code as many times as required. One such loop in the programming is the do while loop.
The do while in javascript is a loop statement that creates a loop that executes until the condition inside the while statement becomes false.
Syntax
Given below is the syntax of the do-while loop.
Statement
The statement or the body of the do-while loop is the code that is to be executed.
Condition
The condition is some expression that can be evaluated to either be true or false, and it decides whether the statement written in the loop will be executed or not. If the condition is true, then the statement will be executed, else it will not be executed.
Description
In Javascript, we use a do-while loop when we are not sure how many times the statement needs to be executed, but we know that the statement must be executed at least once. We are using the do-while loop in this case because the condition is checked at the end of the loop.
How does do while Loop Work in JavaScript?
The do while loop in the Javascript executes the statements written inside the do statement until the condition written inside the while block becomes false. Unlike a simple while loop, the do-while loop will run at least one time because the condition is checked after the complete execution of the loop. Because of its property that the do while loop evaluates the condition after each iteration, it’s often known as the post-test loop.
Do while Flow Diagram
As shown in the diagram, the do-while loop work as follows
- First, the statements written in the block will be executed
- After the first execution, the condition in the while will be checked, if the condition is true, then step 1 will be repeated again
- Else, the loop will terminate
JavaScript Loop Statements
There are many statements in Javascript including the loop statements. Below given is the table of the statements with their description.
Statements | Description |
---|---|
continue | It skips all the statements written after it and goes back to starting of the loop in the next iteration |
break | As the name suggests, it breaks out of a loop |
while | executes the code block while the condition evaluates to true |
do while | executes the code block once, and then while the condition evaluates to true |
for | executes the code block while a condition evaluates to true |
for...in | executes the properties of an object for which it runs |
for...of | executes the values of any iterable object |
Examples
Example 1: Simple JavaScript do..while Statement Example
The following example uses the do-while statement to print the first five natural numbers to the console.
Output
Explanation
In the above example, we performed the following 2 steps :
- Created a count variable, and initialized it with value 1.
- Printed the count variable and incremented its value in each iteration until its value becomes greater than 5.
Example 2: Using an Assignment as a Condition
In this example, we are going to use an assignment operator (=) inside the while statement to write the condition.
Output
Explanation
In the above code, we can see that we replaced the greater than an operator with the assignment operator in the first example. This has to lead to an infinite loop because the assignment operation always returns true after assigning the given value to the variable. So in the first iteration, after printing 1, the value assigned to the count variable is 5. Now from the next iteration onward, the program will print 5 continuously because the condition inside the while statement we always are true.
Example 3: Using the JavaScript do while Statement to Make a Simple Number Guessing Game
In this example, we will create a simple number-guessing game using the do-while loop in Javascript.
The above game is created and played as follows
- First, we defined the minimum and maximum values which is the range of the numbers.
- Then we created a secret number variable named secretNumber and initialized it with a random number between the minimum and the maximum numbers by using the random function in javascript
- Then we created the required variables i.e. guesses (to store the total number of guesses), hint (for storing the hint), and num (which is the guessed number).
- Then we started a do-while loop and performed the following operations
- incremented the guesses variable
- if the guessed number is greater than the secret number, then give the hint to enter a lesser number
- else if the guessed number is less than the secret number, then give the hint to enter a greater number
- else if the guessed number is equal to the secret number, print the congratulations message
- Finally, after the end of the iteration, if the guessed number is equal to the secret number, then the loop will terminate
Conclusion
- The do while loop work similarly to the while loop, but the difference is that the statement in the do while loop will be executed at least once.
- Never use the assignment operator inside the while statement for creating a condition, because it can lead to an infinite loop.
- Use the do while loop, when we are sure that the statements written inside the do block should be executed at least once.