Flatten an Array in JavaScript
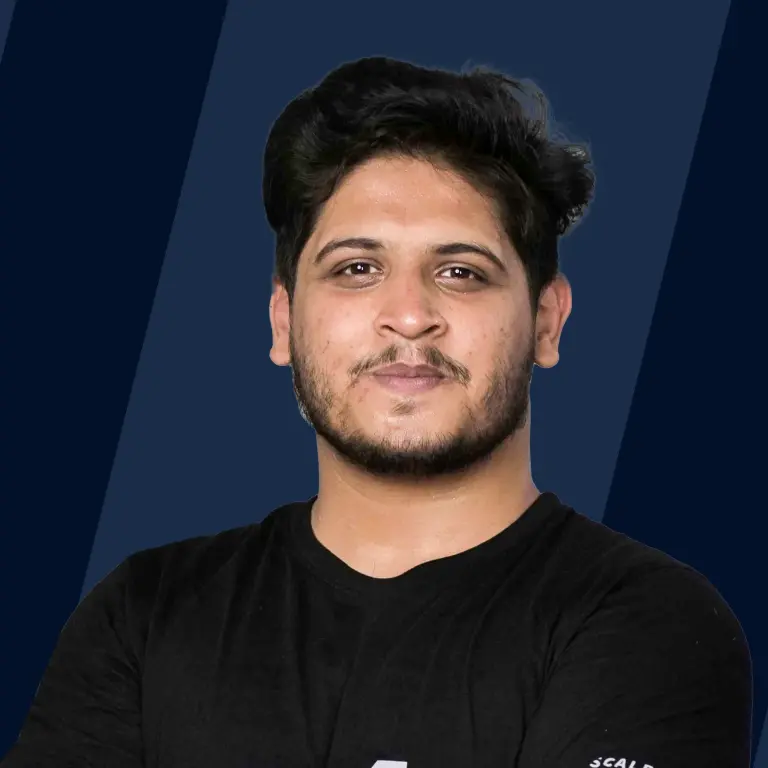
Overview
Flattening an array is a process to reduce the dimensions of an array from n dimensions to 1 dimension. In simple words it means to merge a group of nested arrays present inside a single array, to make it a one-dimensional array.
Introduction
Flattening an array simply means reducing the dimensions of the array. It is a process of taking all the nested elements inside an array and putting them into a single one-dimensional array. For example -
JavaScript Program to Flatten an Array Using concat() and apply()
In this method, we will use the concat() and apply() methods to flatten an array in JavaScript.
JavaScript Implementation
Output
Explanation
In the first step, we have created an array that we want to flatten. Then we have used the concat() and apply() functions to flatten the array. The apply() function will accept a parameter array as an argument and convert the array into one dimension and the concat() function will concatenate the result to make it a single array. Finally, we have printed our flattened array.
JavaScript Program to Flatten an Array Using Spread Operator in ES6
In this method, we will use the Spread Operator in ES6 to flatten an array in JavaScript.
JavaScript Implementation
Output
Explanation
In the first step, we have created an array that we want to flatten. Then we used the Spread Operator '...' in ES6 to flatten the array. The Spread Operator '...' is used before the array to flatten the array and the concat() function will concatenate the result to make it a single array. Finally, we have printed our flattened array.
JavaScript Program to Flatten an Array Using the reduce method
In this method, we will use the reduce method to flatten an array in JavaScript.
JavaScript Implementation
Output
Explanation
In the first step, we have created an array that we want to flatten. Then we used the reduce method to flatten the array. Finally, we have printed our flattened array.
JavaScript Program to Flatten an Array Using forEach()
In this method, we will use the forEach() loop to flatten an array in JavaScript.
JavaScript Implementation
Output
Explanation
In the first step, we have created an array that we want to flatten. Then we used the forEach() loop to flatten the array. Inside the forEach() loop, we have used the Spread operator '...' to flatten the array. Finally, we have printed our flattened array.
JavaScript Program to Flatten an Array Using reduce, concat, and isArray Recursively
In this method, we will use the reduce(), concat(), and isArray() methods recursively to flatten an array in JavaScript.
JavaScript Implementation
Output
Explanation
In the first step, we have created an array that we want to flatten. Then we have used the concat() and isArray() to flatten the array. The isArray() method accepts the elements of the array as an argument one by one, and the concat() function will concatenate the result to make it a single array. Finally, we have printed our flattened array.
JavaScript Program to Flatten an Array Using While Loop with a Recursive helper Function
In this method, we will use while Loop with a recursive helper function to flatten an array in JavaScript.
JavaScript Implementation
Output
Explanation
In the first step, we have created an array that we want to flatten. Then we used while Loop with a Recursive helper Function to flatten the array. The while loop we recursively call the helper function, until the array is reduced to a single dimension. Finally, we returned the flattened array and printed it.
JavaScript Program to Flatten an Array Using a Stack
In this method, we will use a stack to flatten an array in JavaScript.
JavaScript Implementation
Output
Explanation
In the first step, we have created an array that we want to flatten. Then we used the isArray() function and a stack to flatten the array. The isArray() function accepts the elements of the array as an argument one by one. If the value of the passed parameter is not a single element, then we will use the spread operator '...' to flatten the array, then we will push these elements into the stack Else if the passes parameter is a single element, we will simply push the element into the resultant list. Finally, we have reversed the resultant array 'flattened_arr' and returned this array.
JavaScript Program to Flatten an Array Using a Generator Function
In this method, we will use a generator function to flatten an array in JavaScript.
JavaScript Implementation
Output
Explanation
In the first step, we have created an array that we want to flatten. Then we used a generator function to flatten the array. The generator function will accept 2 parameters as an argument, i.e. array and depth. The depth signifies the maximum dimension of the array. The yield keyword is used inside the generator function to resume or pause a generator function. Whenever the function returns any value, it returns it with the help of the ‘yield’ keyword. Finally, we have printed the flattened array.
JavaScript Program to Flatten an Array Using the flat() Method
In this method, we will use the flat() function to flatten an array in JavaScript. Here we can get an error i.e. arr.flat is not a function, because the flat() function is not supported by many browsers, it is supported in NodeJS.
JavaScript Implementation
Output
Explanation
In the first step, we have created an array that we want to flatten. Then we have used the flat() function to flatten the array The flat() function will accept an array as an argument and it will return the flattened array. Finally, we have printed the flattened array.
JavaScript Program to Flatten an Array Using the flatten() Method
In this method, we will use the flatten() function to flatten an array in JavaScript. But for using the flatten() method, we have to use the underscore module in JavaScript. So, first, we must install the underscore library using npm install underscore, and then we can use the flatten() function.
JavaScript Implementation
Output
Explanation
In the first step, we have created an array that we want to flatten. Then we have used the flatten() function to flatten the array The flatten() function can only be used by first installing the underscore module. The flatten() function will accept an array as an argument and it will return the flattened array. Finally, we have printed the flattened array.
JavaScript Program to Flatten an Array Using the flattenDeep() Method
In this method, we will use the flattenDeep() function to flatten an array in JavaScript. But for using the flatten() method, we have to use the lodash module in JavaScript. So, first, we must install the lodash library using npm install lodash, and then we can use the flattenDeep() function.
JavaScript Implementation
Output
Explanation
In the first step, we have created an array that we want to flatten. Then we have used the flattenDeep() function to flatten the array The flattenDeep() function can only be used by first installing the lodash module. The flattenDeep() function will accept an array as an argument and it will return the flattened array. Finally, we printed the flattened array.
Conclusion
In this quick tutorial, we have discussed 11 different approaches to flatten an array in JavaScript and we can conclude the following
- Flattening an array is a process to reduce the dimensions of an array from n dimensions to 1 dimension
- It is a process of taking all the nested elements inside an array and putting them into a single one-dimensional array
- The yield keyword is used inside the generator function to resume or pause the generator function and whenever the function returns any value, it returns it with the help of the ‘yield’ keyword.
- The flat() function is not supported by many browsers so it may give an error, it is however supported in NodeJS.
- We must install the underscore library using npm install underscore, to use the flatten() function.
- We must install the lodash library using npm install lodash, to use the flattenDeep() function.