JavaScript For In
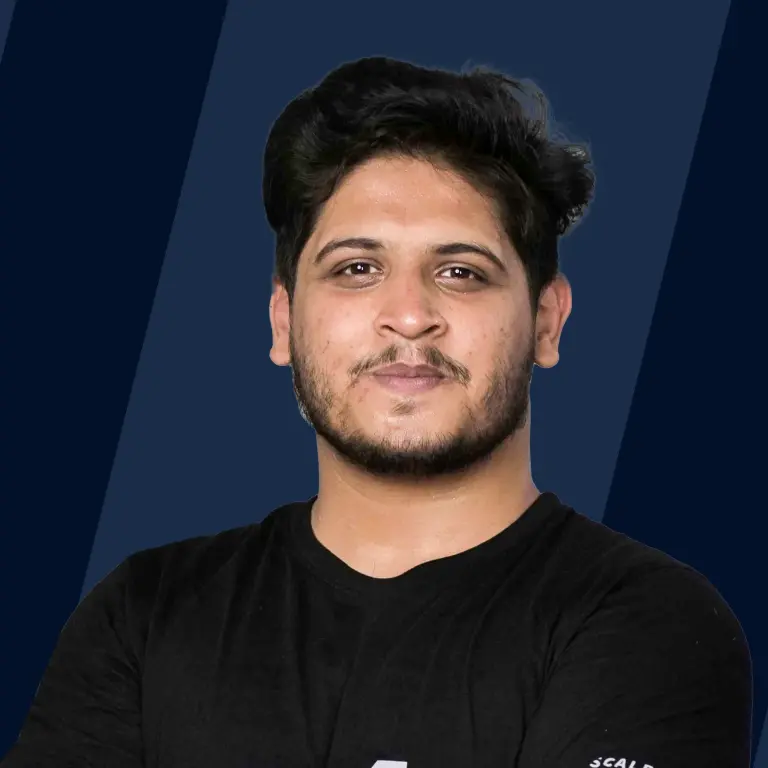
Introduction
JavaScript's 'for...in' loop makes it easier to iterate over object attributes. It's a powerful technique that allows you to easily browse enumerable properties. Unlike arrays, 'for...in' gets keys and provides access to matching values. It must be used with caution because it travels throughout the prototype chain. Use this ability for concise object investigation, but be cautious of inherited characteristics. Understanding 'for...in' provides you with a versatile tool for efficiently exploring object properties in JavaScript.
Syntax for JavaScript For In
The JavaScript 'for...in' loop is a versatile and effective mechanism for iterating over an object's attributes. Understanding its syntax and arguments is critical for maximizing its functionality in your project. Let's look into the complexities of the 'for...in' loop and the arguments that make it a popular choice for object traversal in JavaScript.
The syntax of the 'for...in' loop is quite straightforward:
In this context, 'variable' stands in for a different property name during each loop iteration, while 'object' refers to the object being looped over. The for...in loop goes through all the enumerable properties of the object, giving a short and simple way to access and change the object's properties.
Parameters for JavaScript For In
- Variable Declaration: The 'variable' in the loop header is a placeholder that represents the value of each enumerable property in the object throughout each iteration. This dynamic assignment enables you to operate fluidly with several property names within the loop body.
- Object Iteration: The 'object' option specifies the target object being iterated. It may be any JavaScript object with enumerable attributes. The loop moves through each property, allowing for efficient and simple code execution.
Examples of JavaScript For In
JavaScript, an efficient and dynamic programming language, provides several tools to help developers improve their coding experience. One such effective feature is the 'for-in' loop, which makes it easy to traverse over an object's attributes. In this article, we'll look at practical examples of how to use the 'for-in' loop, with an emphasis on its simplicity and adaptability.
Simple Example to Illustrate the For-in Loop
Let's start with a simple example to understand the fundamentals of the 'for-in' loop. Consider an object called 'user' with fields like 'name', 'age', and 'email'. Using the 'for-in' loop, we can easily go among these attributes, making our code more compact and understandable.
For In Over Arrays
The 'for-in' loop is not restricted to objects; it may also be used to iterate through the items of an array. Let's look at how this can be accomplished:
Iterating Own Properties
Sometimes you want to iterate over an object's attributes rather than any inherited ones. The hasOwnProperty function comes in handy in such circumstances, enabling you to filter out inherited properties:
Array.forEach()
JavaScript, the programming language that animates web design, provides an abundance of tools to streamline your code. One such treasure is the Array.forEach() method, a capable function that simplifies iterating through arrays.
Picture you have an array of information, and you want to carry out a particular task on each piece. Rather than using old-fashioned for loops, Array.forEach() comes to the rescue to simplify things. This method accepts a callback function as its input, running it once per array item. Example:
In this code snippet, we utilize the Array.forEach() method to loop through the fruits array. The forEach() method takes a callback function that gets invoked for each element in the array, passing the element itself and its index as arguments. This allows us to easily execute any custom logic on each item as we iterate through the array. By leveraging forEach() in this way, we can iterate over the array elements and perform operations in a concise, readable manner.
Browser Compatibility
Navigating the complexities of JavaScript's 'For In' loop necessitates an awareness of browser compatibility to enable smooth execution across several settings. When using the 'For In' loop, it is critical to examine the compatibility matrix, which includes common browsers such as Chrome, Firefox, Safari, and Edge.
The adaptability of JavaScript extends across browser ecosystems, although there are differences in how the 'For In' loop syntax is interpreted. While Chrome and Firefox execute the loop seamlessly, developers should exercise caution when dealing with Safari and Edge, since anomalies may occur.
Test it in many browsers to confirm that your script is universally compatible. Use technologies like Babel for transpilation to ensure cross-browser uniformity. This proactive strategy ensures a seamless user experience by reducing potential malfunctions across many browsing situations. Always study browser compatibility tables and documentation to remain up to speed on the latest upgrades and best practices, which will allow you to create powerful and globally compatible JavaScript apps.
Conclusion
- The "for...in" loop streamlines the process of enumerating object attributes, allowing you to easily navigate between keys.
- Using "for...in" improves the readability of your code when dealing with objects, speeds up the iteration process, and makes your code more succinct.
- The "for...in" syntax in JavaScript is more than simply iteration; it also allows for dynamic exploration of object attributes, allowing for more versatility when dealing with different data structures.
- The "for...in" loop organises your code and promotes maintainability by making managing and updating object values easier.
- Using "for...in" eliminates the need for hardcoding property names, resulting in a more flexible and scalable codebase that dynamically adapts to changes in the object's structure.
- One of the most notable aspects of "for...in" is its ability to cycle over an object's characteristics and those inherited from its prototype, demonstrating its adaptability in complicated settings.