What is the instanceof Operator in JavaScript?
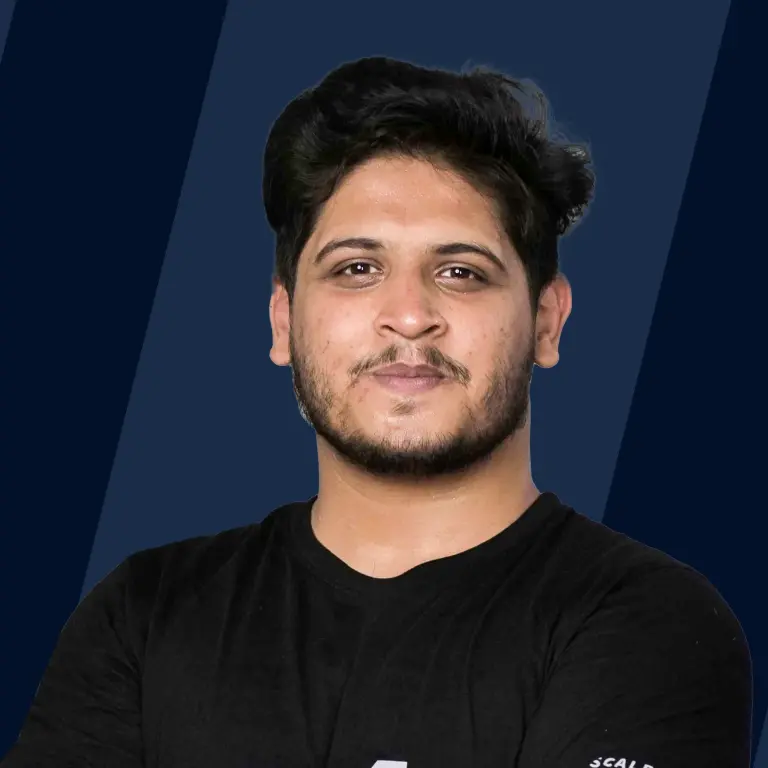
The JavaScript instanceof operator is used to check the type of an object at the run time and returns either true or false. It will return true if the object is an instance of a particular class, else it will return false.
Syntax
Parameters
-
ObjectName: The name of the object.
-
ObjectType: The Type for which the instance of the object is tested.
Exceptions
- An exception is thrown if ObjectType is not an object.
- If the ObjectType does not contain a @@hasInstance method, then the ObjectType should be a function so that the @@hasInstance is not required.
Description
The JavaScript instanceof operator is used to check the type of an object at the run time and returns either true or false. It will return true if the object is an instance of a particular class, else it will return false.
Instanceof and Multiple Realms
JavaScript execution environments let it be windows, frames, etc. have different built-ins (different global objects, different constructors, etc.). This may result in unexpected results.
For example
The above code will return false, because
This is a big issue because the scripts are dealing with multiple frames and windows, and passing objects from one context to another via functions with help of functions.
Why do We Need an Instanceof in JavaScript?
In JavaScript, we do not explicitly define a type while declaring a variable i.e. we just use var abc; which could be an integer, character, string, array, or any user-defined datatype as there are in other languages. Hence, in Javascript, we are having an instanceof operator to check if an object belongs to which of the specified data type or classes.
The Instanceof Operator and Inheritance
Here we will see how we can use the instanceof operator for checking whether the object is an instance of the given class or not.
Javascript Implementation
Output
Explanation
- In the first step, we created a class named Month and used the Symbol.hasInstance method.
- Then inside the class we used the if condition, to check whether the passed object is an instance of the class or not.
- Then we have created an object obj in which the value of January is initialized as true
- Finally, we have printed the result.
Symbol.hasInstance
As we know that with the help of the instanceof operator we can check if an object is an instance of a class or not, in the same manner, we can check whether the class contains an instance of an object or not with the help of the Symbol.hasInstance. The Symbol.hasInstance will return true if the class contains an instance of an object, else it will return false.
Javascript Implementation
Output
Explanation
- In the first step, we created a class named Day and created a constructor inside it.
- Then we created an object Sunday and initialized it as the instance of the class Day.
- Finally, we have checked whether the class Day has any instance of the object Sunday or not by using the Symbol.hasInstance method.
Examples
Example 1: Using instanceof with String
In this example, we will see how the instanceof operator behaves while using the String objects.
Javascript Implementation
Output
Explanation
- In the first step, we created a sentence and initialized it with "Today is Monday".
- Then we created a string object.
- Finally, we have checked which object is an instance of which class.
Example 2: Using instanceof with Date
In this example, we will see how the instanceof operator behaves while using the Date objects.
Javascript Implementation
Output
Explanation
- In the first step, we created an object of the Date class and named it new_date.
- Finally, we have checked that the object is an instance of which class.
Example 3 : Objects Created Using Object.create()
In this example, we will see how the instanceof operator behaves when we create the object using the Object.create() method.
Javascript Implementation
Output
Explanation
- In the first step, we created obj1 by using the {}.
- Then we created a null object by using the Object.create method.
- Finally, we have checked which object is an instance of which class.
Example 4: Not an instanceof
In this example, we will see how we can use Not operator with the instanceof operator to check whether an object is an instance of a class or not.
Javascript Implementation
Output
Explanation
- In the first step, we created two functions named Class1() and Class2() and these functions are empty.
- Then we created an object obj and we made it as the instance of Class2().
- Then we checked which object is an instance of which class.
- Then inside the if condition, we have used the Not an instanceof method, by applying the ! (not) operator in front of the instanceof method, and if the condition is true, we have made the object the instance of Class1().
Conclusion
In this quick tutorial, we have discussed the typeid() operator in C++. We can extract the following conclusions from the article.
- The JavaScript instanceof operator is used to check the type of an object at the run time.
- The JavaScript instanceof operator will return true if the object is an instance of a particular class, else it will return false.
- The JavaScript instanceof operator accepts two parameters as an argument i.e. ObjectName and ObjectType.
- The JavaScript instanceof operator will throw an exception if the ObjectType is not an object