What is an Iterator in JavaScript?
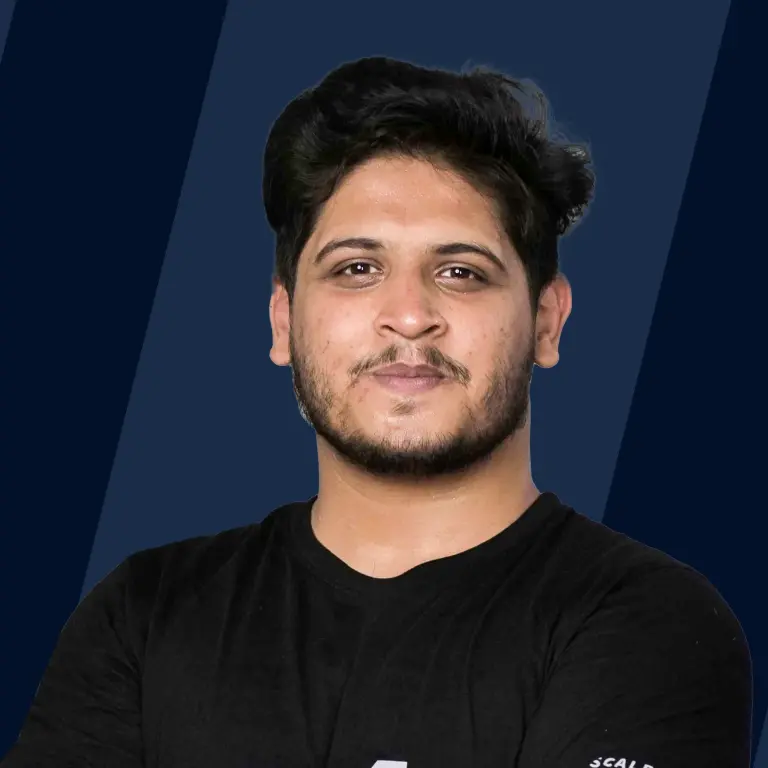
JavaScript provides a protocol to iterate over the collection types like Array, Set, Map, and other data structures. It defines the sequences and allows us to traverse over a collection or list using the for...of the loop. Javascript iterator must implement a next() function and it returns the object that contains the { value and done }. Here done is the boolean value determining if the last value of the sequence has been finished then it’s true, false otherwise. and the value is the next value in the iteration sequence.
Making any Object Iterable
To make any object iterable, we need to add a method to the object i.e Symbol.iterator.
- Let’s understand this better with the help of an example.
- In the above example, if we try to iterate over the cities object, The following error message will appear:
- First, we must add an @iterator function in order to make this object iterable. This symbol is reachable through Symbol.iterator as shown below.
- As mentioned, the javascript iterator function returns an object containing the two attributes { value and done } as follows:
- In the above code done is the boolean value determining if the last value of the sequence has been finished or not, and the value is the next value in the iteration sequence. By calling the next function of the iterator object, we can test it.
In this example, using for...of will not generate any results because done was set to false immediately. The reason we wanted to make this object iterable is that we don't get any user names by implementing it this way using the "for...of" loop.
The For Loop Issues
Let’s take a case when we have them in data an array, we will use a for-loop to iterate over the array elements as shown below:
Output
Here, The variable i is used in the for-loop to keep track of the index of the cities array. Every time the loop runs, the value of i increments while the value of i is less than the total number of elements in the city's array.
Using the for-loop is simple and easy to understand. But when you nest one loop inside another, the complexity increases. And while using for-loop, we have to take care of the conditions ( such as defining the range ) for the variable i Otherwise infinite loop will occur. Also, it is error-prone to keep track of multiple variables inside the loops. In order to overcome these issues and errors caused by keeping track of loop indexes, we use for...of the loop.
Explanation
The for...of is more advanced than the for-loop because it shows the code's real intent, that is to access each element in the sequence by iterating over an array. Not just an array, the for...of loop can also make a loop over any iterable object.
Implementing the Iterator Function
To implement the javascript iterator function, we must first access the keys of the object by calling Object.keys on the this keyword that represent cities. Here, this keyword refers to the parent of the function, cities array in our case. We can only use this to access the keys if the iterable function was defined with the function keyword.
- Two additional variables are required to keep track of our iterations.
In order to maintain the data between iterations, we define these variables outside of the next function but inside the iterator function.
- Using the indexes we defined earlier, we can get the array of users of the current user and the current city in the next function as shown below,
- Increment the indexes in each iteration. Traverse and increment the user index every time until the last user of a given city set the user index to 0, and increment the city index on reaching the last user.
Be careful not to iterate with for...of on this object. This will lead to an infinite loop because done always equals false. Now, as the last step, we have to add an exit condition i.e set the done to true.
Our javascript iterator function will look like this, after putting everything together,
- Finally, Using a for...of loop, we can quickly retrieve all the names from our object as a result.
Output
Iteration Protocols
There are two types of iteration protocols as discussed below, iterator protocol and iterable protocol.
-
Iterator protocol: The javascript iterator protocol defines a standard way to generate a proper sequence of finite or infinite values. A javascript object is an iterator when it has a next() method that returns an object with two attributes as follows,
- done: It is the boolean value determining if the last value of the sequence has been finished or not.
- value: It is the next value in the iteration sequence.
-
Iterable protocol: A javascript object is said to be iterable when it contains a method called Symbol.iterator that returns the object which conforms to the iterator protocol and takes no argument.
The relationship between iterables, iterators, and next may be easier to understand with the help of the below diagram.
Iterators
A javascript iterator is an object which defines the sequences and allows us to traverse over a collection or list using the for...of loop. Iterators are only used when necessary, but arrays can be allocated in their entirety. As a result, iterators are able to express sequences with an infinite length, such as the range of integers between 0 and infinity. Let's see the example for the same,
Then the iterator will look like this:
Output
Explanation
We have simply created a range iterator that defines a sequence of integers from start to end, separated by one step. The final return value is the sequence size it created, tracked by the variable count.
JavaScript next() Method
Javascript iterator implements a next() method that returns the next item in the sequence and it contains the two attributes value and done.
- done: It is the boolean value determining if the last value of the sequence has been finished or not. If the iteration is completed, the done property is set to true, else it is set to false.
- value: It is the next value in the iteration sequence and can be of any data type.
We can call the next() method repeatedly to iterate over the iterator object. The done property is set to false only when the next() method reaches the end of the sequence.
User-Defined Iterator
To access the next element, we can also make our own iterator and use the next() method. For example,
Output
Explanation
Every time the next() method is called, the printData() function gets executed only once and shows the value of an array. At last, when there is no more data left in an array, the done is set to true, with the value as undefined.
Conclusion
- Javascript iterator defines the sequences and allows us to traverse over a collection or list using the for…of the loop.
- JavaScript provides a protocol to iterate over the collection types like Array, Set, Map, and other data structures.
- Javascript iterator must implement a next() function and it returns the object that contains the { value and done }.
- done is the boolean value determining if the last value of the sequence has been finished or not. If the iteration is completed, the done property is set to true, else it is set to false.
- value is the next value in the iteration sequence and can be of any data type. To make any object iterable, we need to add a method to the object i.e Symbol.iterator.
- A javascript object is said to be iterable when it contains a method called Symbol.iterator that returns the object which conforms to the iterator protocol and takes no argument.
- Iterators are only used when necessary as iterators are able to express sequences with an infinite length, such as the range of integers between 0 and infinity.