JavaScript Math.pow() Function
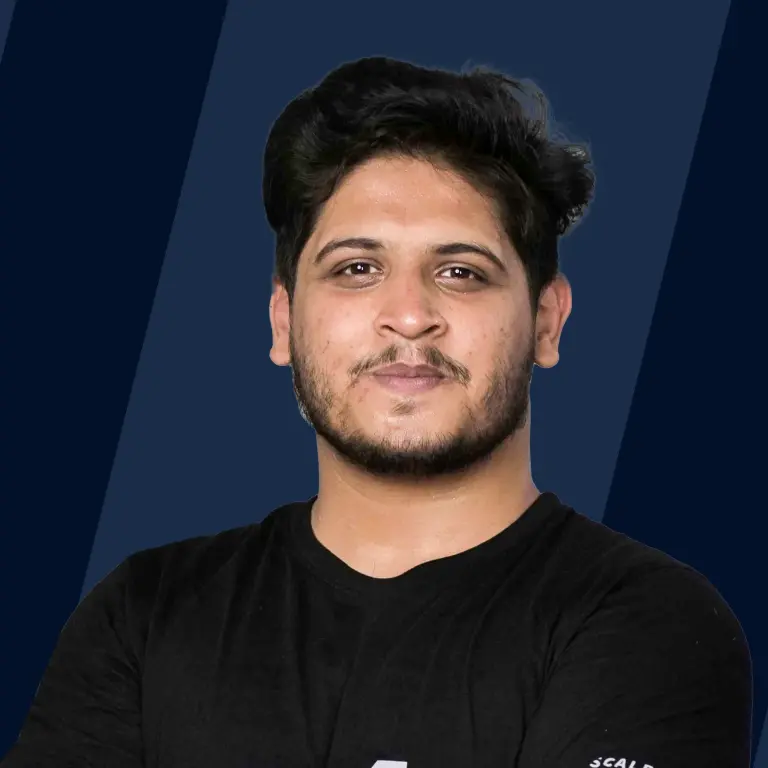
Overview
The Math.pow() function is an in-built function in JavaScript that returns a number equal to the base number raised to its exponent. It takes two parameters - the base and the exponent. The time complexity of the function is O(1).
Syntax of Math.pow() in Javascript
The syntax for Math.pow() function in JavaScript is given below:
Parameters of Math.pow() in JavaScript
The Math.pow() function in JavaScript takes two parameters as follows.
- base: It is the base number that is to be raised.
- exponent: It is the value used to raise the base.
Return Value of Math.pow() in Javascript
The Math.pow() function in JavaScript returns a number that is equal to the base raised to its exponent. However, if the base is negative and the exponent is fractional, it returns NaN.
Exceptions of Math.pow() in Javascript
The Math.pow() in JavaScript returns NaN if the base is negative and the exponent is fractional. This is because when a negative number is raised to a fractional number, the resulting value can be an imaginary number.
The Math.pow() function in Javascript returns NaN in the following cases.
-
If the base is negative and the exponent is a fraction.
Example 1: If the base is negative and the exponent is in fraction, the Math.pow function returns NaN.
Output:
Explanation: We store the output of the function in the variable result. We are taking a negative number(-7) as the base and a fractional number as the exponent. We are printing the output in Line 4. The output is NaN.
-
If the base or the exponent is NaN.
Example 2: If the base is NaN, the Math.pow function returns NaN.
Output:
Explanation: We store the output of the function in the variable result. We are taking NaN as the base and a 2 as the exponent. We are printing the output in Line 4. The output is NaN.
Example 3: If the exponent is NaN , the Math.pow function returns NaN.**
Output
Explanation We store the output of the function in the variable result. We are taking 2 as the base and NaN as the exponent. We are printing the output in Line 4. The output is NaN.
-
If the base is 1 and the exponent is Infinity.
Example 4: If the base is 1 and the exponent is Infinity ,the Math.pow function returns NaN.
Output:
Explanation: We store the output of the function in the variable result. We are taking 1 as the base and Infinity as the exponent. We are printing the output in Line 4. The output is NaN.
Example 5: If the base is -1 and the exponent is Infinity ,the Math.pow function returns NaN.
Output:
Explanation: We store the output of the function in the variable result. We are taking -1 as the base and Infinity as the exponent. We are printing the output in Line 4. The output is NaN.
Example 6: If the base is 1 and the exponent is -Infinity ,the Math.pow function returns NaN.
Output :
Explanation: We store the output of the function in the variable result. We are taking 1 as the base and -Infinity as the exponent. We are printing the output in Line 4. The output is NaN.
Example 7: If the base is -1 and the exponent is -Infinity ,the Math.pow function returns NaN.
Output:
Explanation We store the output of the function in the variable result. We are taking -1 as the base and -Infinity as the exponent. We are printing the output in Line 4. The output is NaN.
Note: NaN stands for Not a Number.
What is Math.pow() in JavaScript ?
Math.pow() is a built-in function in JavaScript that returns the value of the base number when it is raised to the exponent. The time complexity of the function is O(1).
It can be mathematically expressed as Math.pow(a,b) =
Note: It is a static method under the Math class, i.e., we do not need to create an object to use this method.
More Examples of Math.pow() in JavaScript
-
Example 1: When the base and exponent are positive integers, the Math.pow function returns a positive integer.
Output:
Explanation: We store the output of the function in the variable result. We are taking 2 as the base and 10 as the exponent. We are printing the output in Line 4. The output is 1024.
-
Example 2: When the base and exponent are positive integers, the Math.pow function returns a positive integer.
Output:
Explanation: We store the output of the function in the variable result. We are taking 10 as the base and 3 as the exponent. We are printing the output in Line 4. The output is 1000.
-
Example 3: When the base is a positive integer and the exponent is a fraction, the Math.pow function returns a rational number.
-
Output:
Explanation:
We store the output of the function in the variable result. We are taking 7 as the base and 0.6 as the exponent. We are printing the output in Line 4. The output is 3.2140958497160383. -
Example 4: When the base is a positive integer and the exponent is a fraction, the Math.pow function returns a rational number.
Output:
Explanation: We store the output of the function in the variable result. We are taking 32 as the base and 0.2 as the exponent. We are printing the output in Line 4. The output is 2.
-
Example 5: When the base is a positive integer and the exponent is a fraction, the Math.pow function returns a rational number.
-
Output:
Explanation: We store the output of the function in the variable result. We are taking 81 as the base and 1/4 as the exponent. We are printing the output in Line 4. The output is 3.
-
Example 6: When the base is a negative integer and the exponent is a positive integer, the Math.pow function returns a rational number.
Output:
Explanation: We store the output of the function in the variable result. We are taking -7 as the base and 3 as the exponent. We are printing the output in Line 4. The output is -343.
Supported Browsers
- Google Chrome 1 and above
- Microsoft Edge 12 and above
- Internet Explorer 3 and above
- Firefox 1 and above
- Opera 3 and above
- Safari 1 and above
- Chrome Android 18 and above
- Firefox for Android 4 and above
- Opera Android 10.1 and above
- Safari on iOS 1 and above
- Samsung Internet 1.0 and above
- WebView Android 4.4 and above
- Deno 1.0 and above
- Node.js 0.10.0 and above
Conclusion
- The Math.pow() function in JavaScript returns the value which is equal to the base raised to the exponent number.
- It takes two parameters, the base, and the exponent.
- It returns NaN if the base is negative and exponent is fractional.