JavaScript Merge Objects
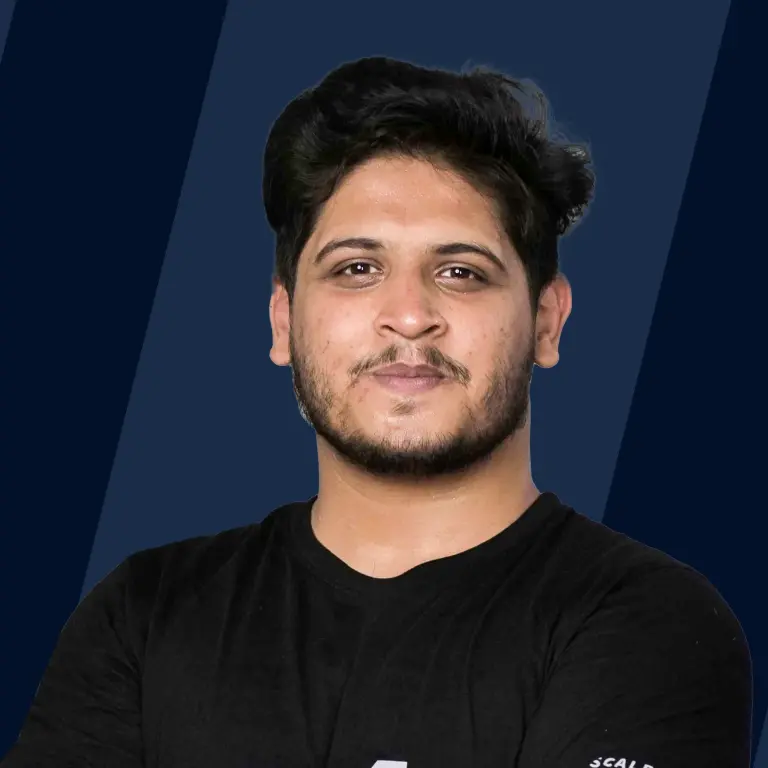
Overview
While working with JavaScript objects, we are often required to merge two or more objects into one; this may be needed while working with some data, handling API calls, or even while we are writing normal programs; knowing how to merge objects proves to be really helpful.
Introduction
Merging is simply a process of combining two entities into one. We may store the resultant as a new entity or can overwrite any existing one. In the same way, by merging two objects in JavaScript, we can combine their different properties into a single unit as one entity and operate on that as needed.
But how it happens? A simple JavaScript object is a collection of key value pairs separated by a comma, meaning we have a key in there and corresponding to that, we have its respective value. So now, when we try to merge two objects, the properties from both objects are brought together and stored inside a single parent object. Sounds simple? Now, just a bit more understanding here. Alright, it's okay; we have two objects, we merged them, and now we have a combined entity, but what if two keys are identical? Then what should happen in that case?
That's where the understanding of shallow merge and deep merge comes into the picture. Let's now try to understand it with the help of examples.
How to Merge Two Objects in JavaScript
Merge Objects Using the Spread Operator (...)
We can merge two JavaScript objects using the built-in javascript's spread(...) operator. What it does is, it expands an iterable structure passed to it (ie. an array, an object etc.) into individual elements. And this helps in making our code concise and better computable.
Spread operator is generally used to make shallow copies of a javascript object. We will discuss what a shallow copy means in the later sections. But for now, let's understand it this way, if we have two objects, and in one or more keys are identical, then merging them using the spread(...) operator will overwrite the former value.
Output
Merge Objects Using the Object.assign() Method
We can use the javascript's pre-defined Object.assign() method to merge two JavaScript objects. What it does is, it copies all enumerable own properties of a given object into a single object and then returns it.
The syntax is,
In the below example, we have used an empty object as the first parameter to the Object.assign() method, the reason for this is, we want to contain the finally merged values of all the sources, here obj1 and obj2 into a single empty object and then want to return it. But instead of it, if we want to merge all the source object to an existing object, then that can be done simply by passing that specific object as the first parameter.
Output
The Shallow Merge
Shallow merge is a way of merging in Javascript. It basically merges two JS objects, but there is a catch here, if we have a key or keys in both the objects with the same name, then doing a shallow merge will replace the value of the former object with the latter. It will not check if the pointed object is nested and contains some child objects having varying keys, it will just overwrite them completely.
Let's understand this with the help of an example.
Output
Here we can see that we have the key num2 in both the obj1 and obj2, and when we shallow merged them, the initial value of num2, which was an object, got overwritten with another object which was the initialized value of key num2 in obj2.
The Deep Merge
Opposite to shallow merge, deep merge performs the deep merging, by going inside the nested objects (if present). When we perform deep merge, it automatically does not replaces the value if two keys are identical, instead, it first tries to look, if the object is nested, and this continues till it reaches the base level of that key. And in this process if is finds two keys are identical and they do not have any nested children, then it replaces them, else it just concatenates that child key at its appropriate location in the parent object.
Lets' understand this with the help of an example.
Output
Here in this example, we can see that while deep merging the obj1 and obj2, the complete object was not overwritten directly; instead, it first went into the nested object, tried to look for identical keys, and as there were none at the base level, hence it finally concatenates them at an appropriate location into the parent object.
Using the Custom Function to Merge Objects
We can also create our own function to merge two objects in JavaScript.
Output
Explanation Here, we are first initializing the obj1 and obj2, then we are calling the merge() function with the created objects as parameters. Inside the merge() function, we are receiving the arguments using the spread operator, and are initializing an empty object to store the finally merged elements.
There we are initiating an internal function named merger() for merging of passed objects. It takes an object as an argument and inside there we are iterating over individual elements of it. We first have a conditional check to find whether the passed object has a property named prop in it, if returned true, then we are assigning that element to the empty target object, and this continues till the complete object is iterated. Once finished, then we are finally returning the target object, and are printing it.
Jquery’s $.extend() Method
We can leverage the functionality of the extend() method present in the JQuery library to merge two JavaScript objects into one.
Output
Explanation Here we are using Jquery's $.extend() method to merge two objects, the method simply takes some number of objects as parameters, and merges their contents into a single object. Here in this case obj1.
Lodash merge() Method
We can also use the lodash library's merge() method to merge two objects in JavaScript.
Output
Explanation Here in this example, we are using lodash library's _.merge() function to merge two objects, what it does is, it recursively merges properties of the source object into the target object. Here even if the keys are identical, then instead of overwriting the complete object, it simply concatenates the values. Use this JavaScript Beautifier to beautify your code.
Conclusion
- Often in our program, we are required to merge two or more JavaScript Objects.
- There are various ways using which we can do that. For example - JavaScript's spread(...) operator, Object.assign() method etc.
- While merging two JS objects, we should remember the concept of Shallow Merging and Deep Merging.
- Shallow Merging overwrites the complete content of the first object if any key between two objects is identical.
- Whereas Deep Merging also checks for nested values while overwriting and only replaces the conflicting values.
- We can also create our own function to merge two objects in JavaScript.
- Along with all these, we can also use Jquery's $.extend() method and the _.merge() method in JavaScript to merge two JS objects.