JavaScript Number toString()
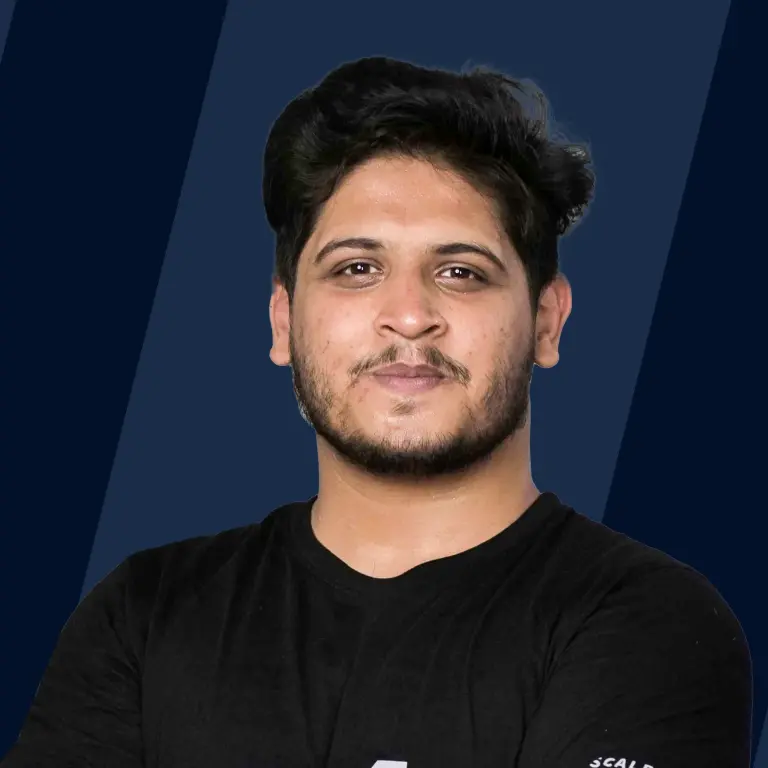
Overview
Every programming language has its methods of converting the variable from one data type to another data type. The number to string javascript is a method of javascript that is used to convert a numeric value to string type. The toString() method is used by Java internally when we need to display an object as text. Also, we can use this when we need to display an object as the string value.
Syntax of JavaScript Number toString()
The syntax for Number to String in the Javascript method is given below:
Parameters of JavaScript Number toString()
The parameters of the Number to String javascript method are as follows:
- Parameter- radix Radix is an optional parameter. It is an integer ranging from 2 to 36. These values specify the base that we will use for the representation of that particular numeric value. For example if there is num.toString(2), then it will convert the number into a binary number string. And if there is num.toString(10), then it will convert the number into a based-10 string and num.toString(16) will convert the number into a hexadecimal string.
Return value of JavaScript Number toString()
The return value of the number to string javascript method is as follows:
- Return Type- String The number-to-string method in javascript returns a numeric value as a string.
Exceptions of JavaScript Number toString()
The exceptions of the number to script javascript method are given below:
- RangeError- The number-to-string method works only for a certain range of radix that is from 2 to 36. If the radix is less than 2 and greater than 36, then it will throw an error known as RangeError.
Example
Let us see an example to understand the number to string javascript method using example. In this example, we will try to convert a number to a string.
Output:
Explanation In this example, we used the number ToString method for converting the number into a string value. First, we created a variable number and assigned the numeric value 15 to the variable. Then we created another variable text. After that, we used the number toString() method and converted the numeric value into the string value, and assigned it to the variable text.
What is JavaScript Number toString()?
When a programmer does coding, there comes a situation when the programmer needs to convert one data type into another data type. In JavaScript, toString() is a built-in method that is used for converting the numeric value to a string value. This method comes under the JavaScript Number object and using this method, we can convert a number type to a string type.
For using the number to string javascript method, we call the method on a number value. The toString() method can be also called immediately on a number value. But parenthesis() must be wrapped to the value. If not done then the JavaScript will throw an error named Invalid error or unexpected token error.
The number toString javascript can also accept a parameter such as radix, also known as the base parameter. This radix allows the user to convert a number to another form. Like we can convert a number from a decimal system whose base is 10, to a string.
More Examples
Now let us see some more examples of the number toString javascript method to understand the method in a better way.
Example 1 In this example, we will convert a number to a string whose base will be 2.
Output
Explanation In this example, we used the number ToString method for converting the number into a string value. First, we created a variable number and assigned the numeric value 20 to the variable. Then we created another variable text. After that we used the number toString() method under which we provided the base value 2 (radix) and then converted the numeric value into the string value and assigned it to the variable text.
Example 2. In this example, we will convert a number to a string whose base will be 8 (Octal) using the number toString javascript.
Output
Explanation In this example, we used the number ToString method for converting the number into a string value. First, we created a variable number and assigned the numeric value 20 to the variable. Then we created another variable text. After that, we used the number toString() method under which we provided the base value 8(radix) and then converted the numeric value into the string value and assigned it to the variable text.
Example 3 In this example we will convert the number to string value using the base whose base will be 16 (Hexadecimal)
Output
Explanation In this example, we used the number toString method for converting the number into a string value. First, we created a variable number and assigned the numeric value 20 to the variable. Then we created another variable text. After that, we used the number toString() method under which we provided the base value 16(radix) and then converted the numeric value into the string value and assigned it to the variable text.
Supported Browsers
The Number toString() javascript method supports a vast range of modern browsers that we generally use today. Following are the names of such browsers and their supported versions given below using a table.
Browser name | Versions |
---|---|
Google chrome | 1 and above |
Edge Browser | 12 and above |
Firefox | 1 and above |
Opera browser | 4 and above |
Safari | 1 and above |
Internet Explorer | 3 and above |
Conclusion
- The number to string javascript is a method in javascript that is used for converting a numeric value into a string value.
- The parameter of the number to string method is radix.
- Radix is defined as the number of unique digits that are used to represent a number in different forms like octal, Hexa, etc.
- The number-to-string javascript method returns the string as the return value.
- We can convert numbers to string in different forms like whose base will be 2 or base = 8, or base = 16
- The number to string method can throw an error named RangeError that occurs when radix is from the range 2 to 36.