Add Two Numbers in JavaScript
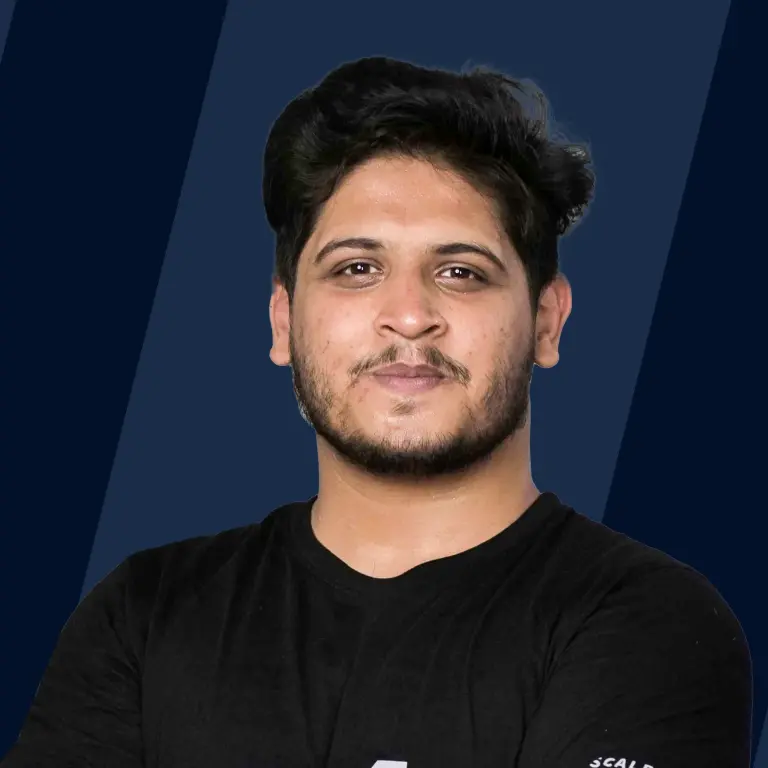
Overview
JavaScript program to add two numbers is one of the fundamental programs that every beginner comes across. After learning fundamental concepts of JavaScript like variables, and functions, adding two numbers would be the best way to implement them. We can add two numbers in JavaScript by using the addition operator +. The Addition operator takes two operands and returns their sum.
Add Two Numbers in JavaScript
Let us see the easiest way of adding two numbers in JavaScript
Let us understand the above JavaScript code; Here, we have initialized two variables, num1 and num2. We assigned 10 to num1 and 30 to num2. Now the statement sum gets initialized with num1+num2 (10 + 30), so the sum of the two numbers 10 and 30 will be assigned to the sum variable. The value 40 will be assigned to the sum variable as a result sum operation.
JavaScript Program to Add Two Numbers in HTML
Let us see how to write JavaScript code that adds two numbers and displays the result in a web browser. To display the result in a web browser, we write the code in a .html file.
Output:
Now Save the above code with the .html extension. Go to any web browser and open the recently saved .html file. We could see that the browser displays the sum of two numbers on the screen.
Explanation :
We have taken two variables as num1 and num2 and initialized them with values 10, and 40, respectively, the sum of these two variables is stored in the sum variable. The result of this sum operation is displayed in the web browser.
JavaScript Program to Add Two Numbers Using Form and TextBox
Let us see the actual JavaScript program that lets the user give the input using Form and Textbox.
Output :
Now, we can see that browser displays two text boxes where one text box is for taking input of num1, and another textbox is for taking input of num2. We will provide values of num1, num2 and then click on the add button, which displays the sum of two numbers.
Explanation:
In the above code, we have taken two HTML input elements which are used for capturing the input numbers. These two numbers have the id of firstnumber and secondnumber, respectively (we will be using these ids at a later stage). We have also taken a button to call the add function after taking the inputs.
Now that we have taken inputs and clicked on add button the flow goes to add a function which is written in a script tag, In the script tag, we have taken three variables as num1, num2, and sum.
Here, num1 and num2 will be used for storing the previously taken two input values. The interesting thing is that the line parseInt(document.getElementById("firstnumber").value) in JavaScript will help us to get hold of firstnumber, In the above line replacing the firstnumber with secondnumber will help us to get hold of secondnumber. By this point, we got our two input numbers stored into num1, num2. So now we will compute the sum using num1+num2
We have taken a HTML element with just input id=”answer”, using this input id we display the sum on the browser, i.e. document.getElementById("answer").value = sum; this line captures the answer id and writes to its value property.
Get User Input One by One
Let us look at one more method for JavaScript Program to add two numbers.
This method looks much similar to the above-discussed method.
Major difference for this implementation is that, here user would give input for only one element at a time.
Explanation:
Just like the previous implementation, we have taken two HTML input elements, but the catch is that we will not display both the HTML input elements at a time, we display one field at a time, and after giving the user input and clicking on enter we display the next HTML input element then we click add so that sum of the two numbers would be displayed.
For the second HTML input element with p id="blockTwo" we have added style attribute as style="display:none;" this ensures that this field will not be displayed. We have done the same thing with p id="blockThree".
Once the user enters a number and clicks on Enter button, the FirstNum() function gets called, and here we get the number by using its id and store it in num1. Now, we check if the number is a true value, if it is true, then by using internal css, we hide the blockOne HTML field by setting temp.style.display = "none"; and display the blockTwo HTML field by setting temp.style.display = "block".
Now we give the input for the second number and click on add so that SecondNum() function gets called, and we get the number by using its id and store it in num2.
In the SecondNum() function, we check if both num1 and num2 are true or not, if both are true then we hide the secondInput by setting temp.style.display = "none". Now we will compute sum and display it on browser by using blockThree, as it was hidden, we will display it by setting temp.style.display = "block"; and display the result by document.getElementById("res").innerHTML = sum.
Here, we enter 7 as the first number and then 8 as the second number, thus getting 15 as the sum.
Conclusion
- As stated previously, adding two numbers in JavaScript is a program that every beginner faces because it clarifies many doubts regarding how to use JavaScript functions, storing sum in a different variable.
- We use the addition operator + to add two numbers in JavaScript.
- In javaScript, we can use various methods to add two numbers, like using JavaScript functions, form and textboxes.
- In javascript, we can take only one input from the user at a time and add them to return the result.