JavaScript Promise.all() Method
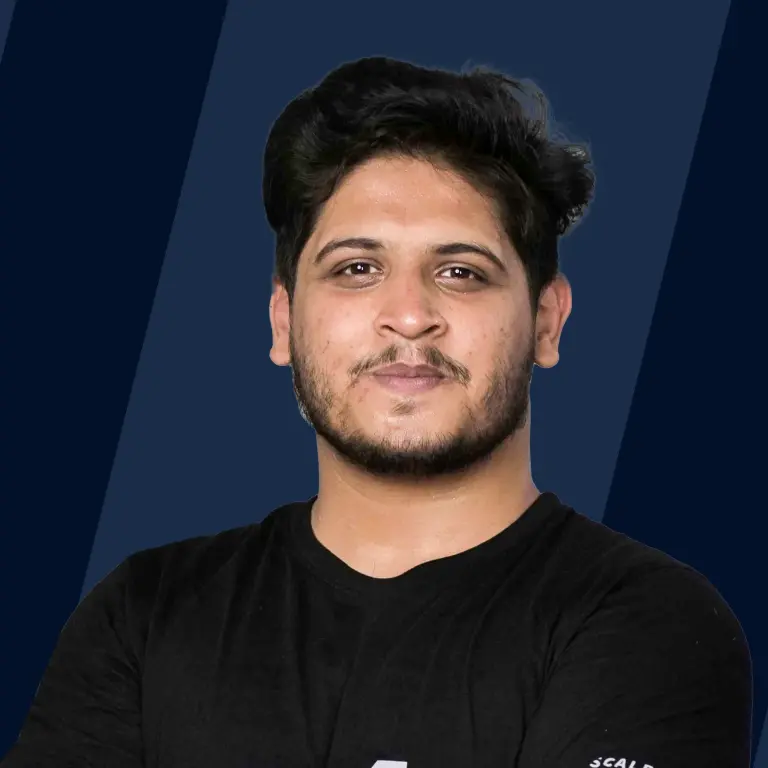
Overview
Promise.all in JavaScript is a built-in functional method that takes in an array of promises as input and returns a single promise only after resolving all the promises of the input array in sequential order. Promise.all in JavaScript throws an error if any one of the passed promises in the input gets rejected, independent of the results of other promises. If the promise returned by this function is resolved, it returns an array consisting of all the returned resolved values of the promises array that was passed as an argument.
Syntax of Promise.all Methods in JavaScript
The syntax of Promise.all method in JavaScript is as follows:
Parameters of Promise.all Methods in JavaScript
The Promise.all method in JavaScript takes in an iterable as a single parameter which denotes an array consisting of promises. An Iterable is basically an object that denotes an array of promises.
Return Value of Promise.all methods in JavaScript
Promise.all in JavaScript has some conditions for returning a single promise and they are as follows:
- It returns an already resolved promise if the functional parameter i.e. iterable passed in the function is empty.
- It returns an asynchronously resolved promise if the iterable passed in the function contains no promises. It simply means Promise.all methods will not wait when there is no promise in the input array to be resolved/rejected and hence, all other operations will be executed without any interruption.
- For all other cases, it always returns a pending promise that can be either resolved or rejected depending on whether all the promises inside the iterable have been resolved or if any of the promises get rejected respectively.
Note: If any of the promises being passed in the function gets rejected, then the value returned by Promise.all in JavaScript will also be rejected irrespective of whether other promises have been resolved or not.
The returned promise is fulfilled in the following cases:
- If we pass an empty iterable as the functional parameter, then the returned promise will be fulfilled synchronously in sequential order. Here, the resolved value will be an empty array.
- If we pass a non-empty iterable as a functional parameter where all the promises are either fulfilled, or it doesn't contain any promises, in that case, the promise returned will be fulfilled asynchronously. It means other operations will keep on executing without any waiting time or interruption.
Short Example to Illustrate Promise.all in JavaScript
As we can see in the below code, we have implemented Promise.all in JavaScript. Initially, we created three promises: p1 with resolved value as "pi" using Promise. resolve() method, p2 with a resolved value of 3.14 and p3 with resolved value as "Maths". For p3, we have used the new Promise() method where we can resolve or reject any value. After that, we used Promise.all methods and have passed an iterable (an array containing all three promises: p1, p2, and p3). Clearly, all three promises will be checked first and when they get resolved, Promise.all will return a resolved value as an array containing resolved values of all those three promises: (["pi", 3.14, "Maths"]). Using .then() method, we will extract the result of returned_promise and will print that on console window. Let us understand this with a diagram indicating the process flow:
Output:
As we can see, the Promise.all in JavaScript has returned a single promise and the resolved value contains an array of values.
How does Promise.all in JavaScript work?
Promise.all in JavaScript is a built-in function that takes in a list of promises as input and returns a single promise when all the promises passed in the input are resolved. When the returned promise by this function is resolved, we get an array of resolved values of all the promises passed in the argument. The Promise.all method in JavaScript helps us to perform async operations and it helps to aggregate the results of multiple promises. It simply means that we can execute multiple promises first by passing them in an array in the function, before moving ahead and processing the rest of the code. This method is helpful in JavaScript when we want the system to execute some of the promises first before executing the rest of the code which is indirectly dependent on the results of those promises. If any promise gets rejected, the rest of the code will also not execute or produce any result.
Notethat if any of the input promises passed to this function gets rejected, Promise.all will also be rejected and will return an error, by not taking into account, the results of other promises being resolved or rejected.
More Examples to Understand Promise.all in JavaScript
Example 1
We have only studied the theory till now that if one promise rejects, the Promise.all in JavaScript will immediately get rejected without taking other promises' results into account.
Have you ever wondered how this thing actually works practically?
As we can see in the below example code, we have created three promises: P1 (using the Promise.resolve() method having resolved value "Promise P1"), P2 (using the new Promise method that can resolve and reject values, containing rejected promise with value "Promise P2") and P3 (with resolved value "Promise P3"). Using Promise.all in JavaScript, we observe that promise P2 is rejected using the new Promise() functional method to resolve/reject values, and therefore, in the final result while printing the resultant values, it will generate an error ignoring the resolved values returned by promises P1 and P3.
Output: This error originated because of rejecting a promise (Promise P2) which was not handled with the .catch() block.
Example 2
In this JavaScript example code, we have three promises (p1, p2, and p3) that contain the pending promise to be resolved/rejected after executing the Promise.all in JavaScript. Note that the Promise.all methods that is returning the p3 promise will contain rejected promise as an input parameter and as a result, p3 will be rejected immediately too. We have used the setTimeout() function that will be executed after the stack becomes empty. It will first generate an error message after executing the P3 promise and then the code written inside the setTimeout() function will be executed. It will basically print the promise states of p1, p2, and p3 i.e, they are returned resolved/rejected values.
Output:
Note that after generating an error and when the stack becomes empty, the setTimeout function block will be executed that will print the promises.
Example 3
Have you ever wondered what if we need to look at the results of all the returned values (despite errors generated due to any rejected promise) of the promises passed as an input to the Promise.all() method?
In this JavaScript example code, we have implemented the Promise.all methods using the good practice of writing code and we have used the .catch() functional block to handle and print the errors generated while execution. Clearly, we have three promises: p1, p2, and p3 with p2 having rejected error values that we get from the new Promise() method to resolve/reject values. While executing Promise.all in JavaScript, the .catch() block will be tested to check and handle the errors and, if there is no error generated, then after receiving the pending promise from the Promise.all methods, we will print the returned values of all the promises being passed as an input array.
Output As we can observe in the output, irrespective of the error generated due to promise P2 while executing the Promise.all method in JavaScript, other promises' results will also be printed. By using the .catch() functional block, we have taken care of errors generated.
Example 4
In this JavaScript example code, we will try to check the order and synchronization in executing promises and will print the time taken to resolve the values. We have three promises initialized: p1, p2, and p3 & we have used the setTimeout() function to resolve or reject the values to be returned. We have used the try-catch block to execute Promise.all in JavaScript and also, to handle and print errors (if generated). Clearly, we will get a promise array returned in the output that will contain the returned resolved values of promises along with the time taken as the message: p1, p2, and p3 executed orderwise.
Output:
Using the try-catch block, the pending promise that we get after executing Promise.all in JavaScript will be fulfilled and resolved and then the resultant resolved values' array will be printed.
Supported Browsers
Some of the browsers that Promise.all in JavaScript supports are as follows:
- Google Chrome 32 & above
- Firefox 29 & above
- Edge 12 & above
- Opera 19 & above
- Safari 8 & above
Note that Internet Explorer is no anymore supported by the method - Promise.all in JavaScript.
Conclusion
- Promise.all in JavaScript is a built-in functional method that takes in an array of promises as input and returns a single promise only after resolving all the promises of that input array.
- Promise.all in JavaScript throws an error if any of the passed promises gets rejected, independent of the results of other promises being resolved or rejected.
- The syntax of Promise.all method in JavaScript is as follows:
- When the returned promise by Promise.all in JavaScript is resolved, we get an array of resolved values of all the promises passed in the argument.
- It returns already resolved promise if the functional parameter i.e. the iterable passed in the function is empty.
- It returns asynchronously resolved promise if the iterable passed in the function contains no promises.
- For all the other cases, it always returns a pending promise that can be either resolved or rejected.
- This method is helpful in JavaScript when we want the system to execute some of the promises first before executing the rest of the code which is indirectly dependent on the results of those promises.
- Promise.resolve(value) –> It is an inbuilt JavaScript function that makes a resolved promise with the given value.
- Promise.reject(error) –> It is an inbuilt JavaScript function as well that makes a rejected promise with the given error.