JavaScript Queue
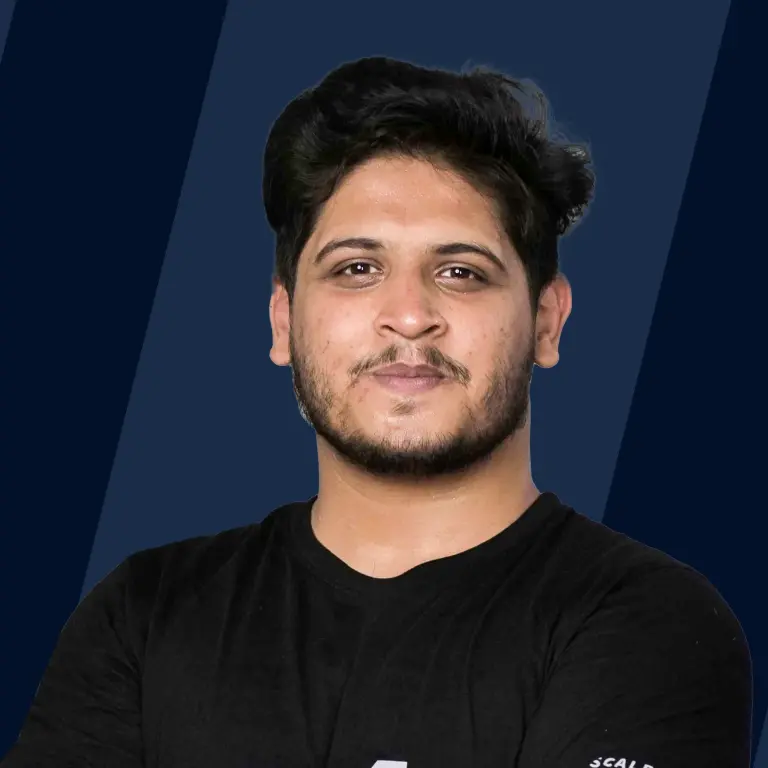
Overview
Javascript Queue is one of the linear data structures used to store data in the memory. The queue is a linear data structure that stores data sequentially based on the First In First Out (FIFO) manner and is also known as the First Come First Served data structure. The queue has two ends, namely- rear and front. The data or an element is inserted from the rear end, on the other hand, elements are removed from the front end.
Introduction to the Queue data structure
A data structure is a way of organizing data for easy, faster, and more effective usage.
JavaScript Queue is one of the linear data structures used to store data in the memory. We can define a queue as a linear data structure that stores data sequentially based on the First In First Out (FIFO) manner. So, the data which is inserted first will be removed from the queue first. Since the first element inserted is served and removed first, the queue data structure is also known as the First Come First Served data structure.
In our day-to-day life, we can see a lot of examples of queues. The queue for a movie ticket is an example of a queue data structure. The person standing in front of the ticket window has come first, and he/she will get the ticket first.
The JavaScript Queue can be implemented in a variety of ways. The JavaScript Queue has two pointers or ends, namely- rear and front. The data or an element is inserted from the rear end, on the other hand, elements are removed from the front end.
A pictorial representation of a queue can be:
Note: A linear data structure is a collection of elements such that each element is arranged sequentially, and each member element is connected to its previous and next element.
There are various ways of implementing a javascript queue. Let us learn how to implement the javascript queue in different ways in the next sections.
An Example of Queue Class Using an Array
As we know, we can implement javascript queue in various ways, let us see the implementation using an array.
Note: An array is a collection of similar data elements stored at contiguous memory locations.
Example:
Output:
As we can see, the element inserted first got removed first, i.e., 1 was inserted first and it got removed first as well.
Note: To remove the first element from an array in javascript, we use the array. shift() method.
Implement a JavaScript Queue Using an Object
The above-mentioned approach i.e. array-based approach of javascript queue implementation is a straightforward approach. We can also implement the javascript queue using classes and objects.
Note: A class can be defined as a template or blueprint that describes the behavior of a real-world entity called an object. An object is an instance of a class.
Let us implement javascript queue using Object:
Output:
Implement the Functions
Javascript Queue is one of the most important and commonly used data structures. We can perform various operations using a javascript queue. Let us learn about the various functions or methods associated with javascript queue.
- enqueue() : The operation of inserting an element of data into the queue is known as enqueue. An element is inserted or enqueued from the rear end of the queue. Since the rear end is always maintained, we can directly insert a new element into the queue. So, the time complexity of inserting an element in the javascript queue is O(1).
Note: If a queue is full, then we cannot insert any new element into the queue. This condition is known as overflow condition.
- dequeue() : The operation of deleting or removing an element of data from the queue is known as dequeue. An element is deleted or dequeued from the front end of the queue. Since the front end is always maintained, we can directly delete an element from the queue. So, the time complexity of deleting an element from the javascript queue is O(1).
Note: If a queue is empty, then we cannot remove any element from the queue. This condition is known as the underflow condition.
-
front() : The front is a kind of pointer that points to the element which is first inserted into the queue. The front of a queue helps us to get the oldest element present in the queue. So, the time complexity of getting the front element of the javascript queue is O(1).
-
rear() : The rear is a kind of pointer that points to the element which is last inserted into the queue. The rear of a queue helps us to get the newest element present in the queue. So, the time complexity of getting the rear element of the javascript queue is O(1).
Helper Methods
As we can see, nearly all of the operations associated with the queue in python take a linear time, i.e. O(1). Hence, the javascript queue is a fast and effective data structure.
So, the javascript queue supports a wide variety of methods. Let us learn about more methods or functions associated with the javascript queue.
-
isEmpty() : The isEmpty() method is provided by the javascript queue to check whether the queue is empty or not. The isEmpty() method uses the front pointer and returns a boolean value. If the isEmpty() method returns true, the queue is empty else, the queue has some elements in it.
-
peek() : The peek() method is provided by the javascript queue to get or extract the first or top element from the queue. The peek() method uses the front pointer to get the top element of the queue.
-
printQueue() : As the name suggests, the printQueue() method is used to print the entire queue.
-
isFull() : The isFull() method is vice versa of the isEmpty() method. The isFull() method is provided by the queue in python to check whether the queue is full or not. We can use the isFull method if we have deified the maximum size limit of the javascript queue. The isFull() method uses the rear pointer and returns a boolean value. If the isFull() method returns true, the queue is filled else, the queue has some space left.
-
size() : The size() method is provided by the javascript queue to get the size of the queue i.e., the number of elements present in the queue.
Implementation
As we have seen, the various methods are associated with the javascript queue. Let us implement the above-mentioned methods of the javascript queue.
Output:
Application: An Interesting Method to Generate Binary Numbers from 1 to n
We can use the javascript queue to generate binary numbers. Let us see how!
Output:
Apart from the above-mentioned use case(s) of javascript queues, there are various other uses of javascript queues:
- Queue data structure is used to implement FCFS CPU scheduling algorithm i.e., first come, first serve to schedule.
- Queue is also used for spooling in printers and as a buffer for various input devices.
- Queue is used in disk scheduling by the Operating Systems.
- Queue is used in handling website traffic.
- Queue is also used in maintaining the playlist in media players.
- Queue is also used to maintain the processes in the waiting queue for the CPU processing.
There are various other uses of the queue. A lot of tree and graph algorithms use queue data structures like level order traversal, etc.
Conclusion
- A data structure is a way of organizing data for easy, faster, and effective usage. Javascript Queue is one of the linear data structures used to store data in the memory.
- Queue is a linear data structure that stores data sequentially based on the First In First Out (FIFO) manner. The queue data structure is also known as the First Come, First Served data structure.
- The Javascript Queue has two ends, namely- rear and front. The data or an element is inserted from the rear end, on the other hand, elements are removed from the front end.
- Javascript Queue can be implemented using a list data structure, dequeue class of Collection module, Queue module, and by defining our class.
- Some of the most commonly used queue methods are: enqueue(element), dequeue(), isEmpty(), isFull(), front(), and rear().