How to Remove a Character from String in JavaScript?
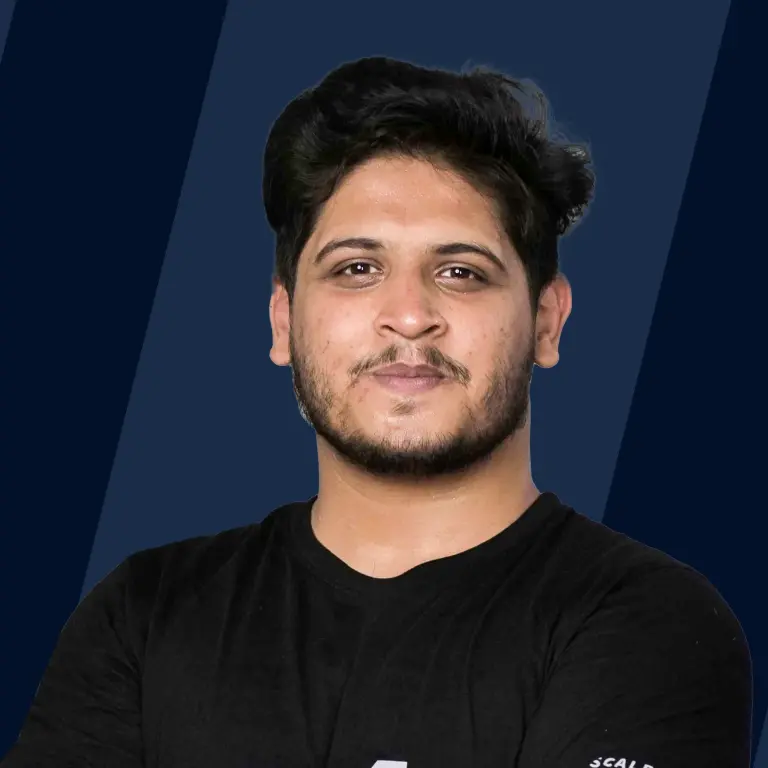
Overview
JavaScript provides users with a number of methods for manipulating strings, transforming them, and extracting useful information from them. Sometimes we write multiple lines of code to make modifications, search for a character, replace a character, or remove a character from a string. All of these operations become tough to complete, so JavaScript provides techniques to make the job easier. These methods make it simple for users to manipulate and alter strings.
Introduction
In JavaScript, removing a character from a string is a common method of string manipulation, and to do so, JavaScript provides a number of built-in methods which make the job easier for the users.
Removing a Character from String in JavaScript
JavaScript String replace()
The replace() method is one of the most commonly used techniques to remove the character from a string in javascript. The replace() method takes two parameters, the first of which is the character to be replaced and the second of which is the character to replace it with. This method replaces the first occurrence of the character. To remove the character, we could give the second parameter as empty.
Syntax:
Example:
Output:
Using a replace() Method with a Regular Expression
Unlike the previous approach, this one removes all instances of the chosen character. Along with the global attribute, a regular expression is utilized instead of the string. It will select every instance of the string and allow you to remove it.
Syntax:
Example:
Output:
Removing Character from String Using substring()
This method will take two indexes and then returns a new substring after retrieving the characters between those two indexes, namely starting index and ending index. It will return a string containing characters from starting index to ending index -1. If no second parameter is provided, then it retrieves characters till the end of the string.
Syntax:
Example:
Output:
The above code will remove the character present at the 1st index, which is e in this case.
Remove Character from String in Javascript Using substr()
This method will take a starting index and a length and then returns a new substring after retrieving the characters from starting index till the length provided. If no second parameter is provided, then it retrieves characters to the end of the string.
Syntax:
Example:
Output:
Removing Character from String Using slice()
This method is the same as the substring method, but with a distinction that if starting index > ending index, then substring will swap both the arguments and returns a respective string, but the slice will return an empty string in that case.
Syntax:
Example:
Output:
Using split() Method
Another way to eliminate characters in JavaScript is the split() method, which is used along with the join() method. To begin, we utilize the split() method to extract the character we want, which returns an array of strings . These strings are then joined using the join() method.
Syntax:
Example:
Output:
JavaScript is the language that powers the modern web. Join our JavaScript certification course and become a front-end development expert.
Conclusion
- Javascript provides various methods for string manipulation, and one of the very common methods for string manipulation is removing a character in the string.
- Javascript provides various methods like replace(), substring(), substr(), split(), slice() etc which makes the task of removing a character in a string very easy.