What is Return Statement in JavaScript?
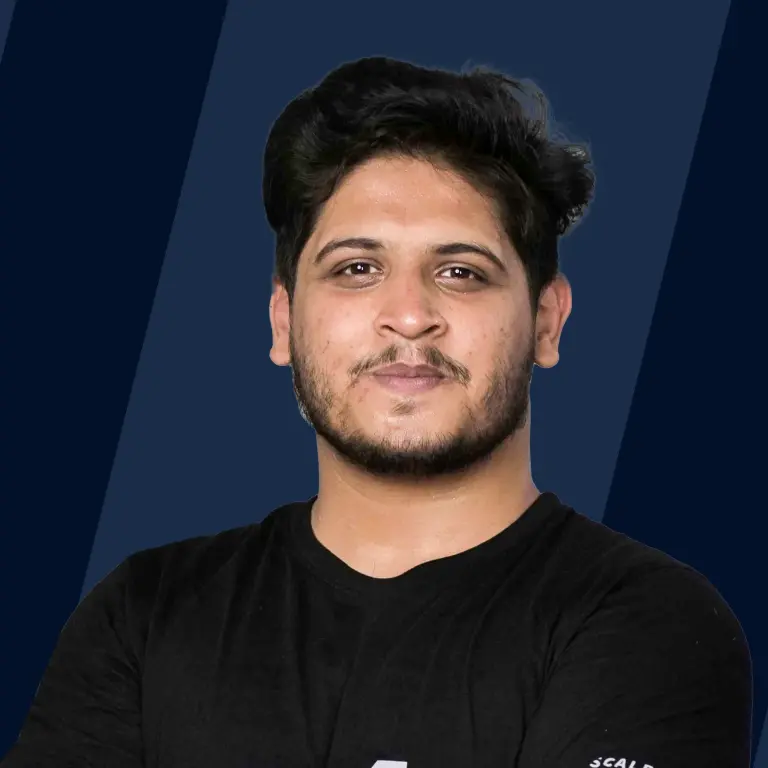
In return function in javascript the return statement in JavaScript is written at the conclusion of the function execution and it is used to stop any more function execution and return the value. The function's body's final statement is always the return statement. If any code is written below the return statement is always unreachable. If the expression is left out, the result is undefined.
Functions can return values in two forms:
- Primitive values (such as number, number, boolean, etc.)
- Object types (such as arrays, objects, functions, etc.)
Syntax
Here, (expression) is:
- It is optional
- It is the value to be returned from the function.
- if the expression after the return is left out, then it returns undefined.
Automatic Semicolon Insertion (ASI)
In the return function in javascript, the automatic inclusion of semicolons has an impact on the return statement (ASI). No line terminator should be used between the return keyword and the expression.
If the return statement is written as follows:
Then the above statements can be re-written by ASI in such way:
The above statements will give a warning that is unreachable code after return statement.
To avoid this issue(prevent ASI), the expression written after the return should be wrapped in parentheses:
Functions Can Return
Primitive Values
In the return function in javascript, the primitive values return values in the form of a string, number, Boolean etc.
- Returning a string:
- Returning a number:
- Returning a boolean:
Object Types
In return function in javascript, the object types return a sequence of elements that is arrays, objects, functions, etc.
Use of Array to return values:
Use of object to return value:
Examples
A Simple Example below for a Return Statement in JavaScript.
Example 1: Let's take a function that returns the product of two numbers-
Output:
Example 2: Calculate the area of the triangle-
Output:
Interrupting a Function Using the Return Statement.
It is defined as when a function gets terminated whenever return is called.
Let's take an example to understand the concept of interruption of a function-
Output:
Browser compatibility
Browser | Support |
---|---|
Safari | yes |
Opera | yes |
Internet Explorer | Yes |
Firefox | yes |
Edge | yes |
Chrome | Yes |
Conclusion
- Return statement is used to terminate the execution of the function in a program.
- Function can return values in two forms that are in primitive values and object types.
- Without a return statement you can't fetch the value from the function it will return undefined.
- When a function with an argument or code to return any value is called, the return statement halts execution and returns its value.
- ASI(Automatic Semicolon Insertion) gives an impact on the return statement that no terminator line should be used between the return keyword and the expression.